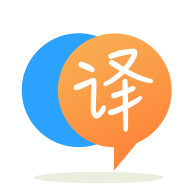
[英]Get the file name without GET parameters from a full path using JavaScript?
[英]How to get the file name from a full path using JavaScript?
有沒有辦法從完整路徑中獲取最后一個值(基於“\\”符號)?
例子:
C:\\Documents and Settings\\img\\recycled log.jpg
在這種情況下,我只想從 JavaScript 的完整路徑中獲取recycled log.jpg
。
var filename = fullPath.replace(/^.*[\\\/]/, '')
這將處理路徑中的 \\ OR /
只是為了性能,我測試了這里給出的所有答案:
var substringTest = function (str) {
return str.substring(str.lastIndexOf('/')+1);
}
var replaceTest = function (str) {
return str.replace(/^.*(\\|\/|\:)/, '');
}
var execTest = function (str) {
return /([^\\]+)$/.exec(str)[1];
}
var splitTest = function (str) {
return str.split('\\').pop().split('/').pop();
}
substringTest took 0.09508600000000023ms
replaceTest took 0.049203000000000004ms
execTest took 0.04859899999999939ms
splitTest took 0.02505500000000005ms
獲勝者是Split 和 Pop風格的答案,感謝bobince !
在 Node.js 中,你可以使用Path 的 parse 模塊...
var path = require('path');
var file = '/home/user/dir/file.txt';
var filename = path.parse(file).base;
//=> 'file.txt'
路徑來自哪個平台? Windows 路徑不同於 POSIX 路徑不同於 Mac OS 9 路徑不同於 RISC OS 路徑不同...
如果它是一個文件名可以來自不同平台的網絡應用程序,則沒有一種解決方案。 然而,一個合理的方法是同時使用 '\\' (Windows) 和 '/'(Linux/Unix/Mac 以及 Windows 上的替代方案)作為路徑分隔符。 這是一個非 RegExp 版本的額外樂趣:
var leafname= pathname.split('\\').pop().split('/').pop();
Ates,您的解決方案不能防止空字符串作為輸入。 在這種情況下,它會因TypeError: /([^(\\\\|\\/|\\:)]+)$/.exec(fullPath) has no properties
失敗TypeError: /([^(\\\\|\\/|\\:)]+)$/.exec(fullPath) has no properties
。
bobince,這是處理 DOS、POSIX 和 HFS 路徑分隔符(和空字符串)的 nickf 版本:
return fullPath.replace(/^.*(\\|\/|\:)/, '');
以下 JavaScript 代碼行將為您提供文件名。
var z = location.pathname.substring(location.pathname.lastIndexOf('/')+1);
alert(z);
不比 nickf 的answer更簡潔,但這個直接“提取”答案而不是用空字符串替換不需要的部分:
var filename = /([^\\]+)$/.exec(fullPath)[1];
一個詢問“獲取沒有擴展名的文件名”的問題參考此處,但沒有解決方案。 這是從 Bobbie 的解決方案修改而來的解決方案。
var name_without_ext = (file_name.split('\\').pop().split('/').pop().split('.'))[0];
我用:
var lastPart = path.replace(/\\$/,'').split('\\').pop();
它替換了最后一個\\
因此它也適用於文件夾。
不需要特別處理反斜杠; 大多數答案不處理搜索參數。
現代方法是簡單地使用URL
API並獲取pathname
屬性。 API 將反斜杠標准化為斜杠。
為了將結果%20
解析為空格,只需將其傳遞給decodeURIComponent
。
const getFileName = (fileName) => new URL(fileName).pathname.split("/").pop(); // URLs need to have the scheme portion, eg `file://` or `https://`. console.log(getFileName("file://C:\\\\Documents and Settings\\\\img\\\\recycled log.jpg")); // "recycled%20log.jpg" console.log(decodeURIComponent(getFileName("file://C:\\\\Documents and Settings\\\\img\\\\recycled log.jpg"))); // "recycled log.jpg" console.log(getFileName("https://example.com:443/path/to/file.png?size=480")); // "file.png"
.as-console-wrapper { max-height: 100% !important; top: 0; }
添加.filter(Boolean)
的前.pop()
如果你總是希望在路徑的最后一個非空部分(例如file.png
從https://example.com/file.png/
)。
如果您只有一個相對 URL 但仍然只想獲取文件名,請使用URL
構造函數的第二個參數來傳遞基本來源。 "https://example.com"
就足夠了: new URL(fileName, "https://example.com")
。 它也可以在前面加上"https://"
你的fileName
-的URL
構造函數接受https://path/to/file.ext
作為一個有效的URL。
<script type="text/javascript">
function test()
{
var path = "C:/es/h221.txt";
var pos =path.lastIndexOf( path.charAt( path.indexOf(":")+1) );
alert("pos=" + pos );
var filename = path.substring( pos+1);
alert( filename );
}
</script>
<form name="InputForm"
action="page2.asp"
method="post">
<P><input type="button" name="b1" value="test file button"
onClick="test()">
</form>
包含在您的項目中的小功能,用於從 Windows 的完整路徑以及 GNU/Linux 和 UNIX 絕對路徑確定文件名。
/**
* @param {String} path Absolute path
* @return {String} File name
* @todo argument type checking during runtime
* @see https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/includes
* @see https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/slice
* @see https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/lastIndexOf
* @example basename('/home/johndoe/github/my-package/webpack.config.js') // "webpack.config.js"
* @example basename('C:\\Users\\johndoe\\github\\my-package\\webpack.config.js') // "webpack.config.js"
*/
function basename(path) {
let separator = '/'
const windowsSeparator = '\\'
if (path.includes(windowsSeparator)) {
separator = windowsSeparator
}
return path.slice(path.lastIndexOf(separator) + 1)
}
完整的答案是:
<html>
<head>
<title>Testing File Upload Inputs</title>
<script type="text/javascript">
function replaceAll(txt, replace, with_this) {
return txt.replace(new RegExp(replace, 'g'),with_this);
}
function showSrc() {
document.getElementById("myframe").href = document.getElementById("myfile").value;
var theexa = document.getElementById("myframe").href.replace("file:///","");
var path = document.getElementById("myframe").href.replace("file:///","");
var correctPath = replaceAll(path,"%20"," ");
alert(correctPath);
}
</script>
</head>
<body>
<form method="get" action="#" >
<input type="file"
id="myfile"
onChange="javascript:showSrc();"
size="30">
<br>
<a href="#" id="myframe"></a>
</form>
</body>
</html>
<html>
<head>
<title>Testing File Upload Inputs</title>
<script type="text/javascript">
<!--
function showSrc() {
document.getElementById("myframe").href = document.getElementById("myfile").value;
var theexa = document.getElementById("myframe").href.replace("file:///","");
alert(document.getElementById("myframe").href.replace("file:///",""));
}
// -->
</script>
</head>
<body>
<form method="get" action="#" >
<input type="file"
id="myfile"
onChange="javascript:showSrc();"
size="30">
<br>
<a href="#" id="myframe"></a>
</form>
</body>
</html>
成功為您的問題編寫腳本,完整測試
<script src="~/Scripts/jquery-1.10.2.min.js"></script>
<p title="text" id="FileNameShow" ></p>
<input type="file"
id="myfile"
onchange="javascript:showSrc();"
size="30">
<script type="text/javascript">
function replaceAll(txt, replace, with_this) {
return txt.replace(new RegExp(replace, 'g'), with_this);
}
function showSrc() {
document.getElementById("myframe").href = document.getElementById("myfile").value;
var theexa = document.getElementById("myframe").href.replace("file:///", "");
var path = document.getElementById("myframe").href.replace("file:///", "");
var correctPath = replaceAll(path, "%20", " ");
alert(correctPath);
var filename = correctPath.replace(/^.*[\\\/]/, '')
$("#FileNameShow").text(filename)
}
對於“文件名”和“路徑”,此解決方案更簡單和通用。
const str = 'C:\\Documents and Settings\\img\\recycled log.jpg';
// regex to split path to two groups '(.*[\\\/])' for path and '(.*)' for file name
const regexPath = /^(.*[\\\/])(.*)$/;
// execute the match on the string str
const match = regexPath.exec(str);
if (match !== null) {
// we ignore the match[0] because it's the match for the hole path string
const filePath = match[1];
const fileName = match[2];
}
var file_name = file_path.substring(file_path.lastIndexOf('/'));
function getFileName(path, isExtension){
var fullFileName, fileNameWithoutExtension;
// replace \ to /
while( path.indexOf("\\") !== -1 ){
path = path.replace("\\", "/");
}
fullFileName = path.split("/").pop();
return (isExtension) ? fullFileName : fullFileName.slice( 0, fullFileName.lastIndexOf(".") );
}
<script type="text\javascript">
var path = '<%=Request.Url.GetLeftPart(UriPartial.Authority) + Request.ApplicationPath %>';
</script>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.