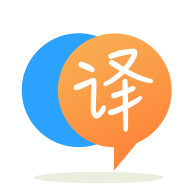
[英](Java) How to parse a coordinate string with multiple possible formats, and convert them into ints?
[英]How to read values in a text document and then make then parse them to ints in JAVA
我正在嘗試讀取文本文檔的行並取這些數字的平均值。 我的計划是首先讀取文本文件中的所有數據。 然后將字符串拆分為String數組,然后將每個索引解析為int
數組。 這是我的代碼,直到閱讀文檔為止。
我的文本文檔:
3,7,24,66,
10、50、20、40,
100、20、69、911,
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
public class Testing
{
public static void main(String[] args) throws IOException
{
try
{
String path;
path = "TestNumbers.txt";
File f = new File(path);
FileReader re = new FileReader(f);
BufferedReader in = new BufferedReader(re);
String line = "";
String store = "";
while((line = in.readLine()) != null)
{
store = store + line;
}
System.out.println(store);
}
catch (FileNotFoundException e)
{
e.printStackTrace();
}
}
}
輸出:3、7、24、66、10、50、20、40,100、20、69、911,
在理想的世界中,我希望值之間用“,”或“”分隔。
我試圖修改store = store + line + " ";
但這失敗了,因為即使readLine()
在空白行上,它也會迭代空間。
我可以遍歷數組以解析int
並取平均值,但是設置要拆分的字符串讓我很沮喪。 我嘗試了String.replace()
, String.trim()
和另一個使我失敗的方法。 這不是家庭作業,我在高中AP CS上,這是我自己的獨立研究。
感謝大家的幫助,你們都展示了很多方法。 最終使用.replace進行轉換,並將“”轉換為“”。 然后通過逗號分開。 我確實想嘗試一下正則表達式。 再次感謝大家。
其他兩種解決方案可能已經是您所需要的,但是這是一種更慣用的方法,可以正確處理邊緣情況:
{
String path = "TestNumbers.txt";
try (BufferedReader reader = new BufferedReader(new FileReader(path))) {
StringBuilder builder = new StringBuilder();
String line;
while ((line = reader.readLine()) != null)
builder.append(line);
String[] split = builder.toString().split("\\D+");
int[] numbers = new int[split.length];
for (int i = 0; i < split.length; i++ )
numbers[i] = Integer.parseInt(split[i]);
// 'numbers' now stores every digit segment there is in your string.
} catch(IOException e) {
e.printStackTrace();
}
}
值得注意的:
BufferedReader
聲明為Closeable
。 String
,而應使用StringBuilder
。 \\D+
以刪除所有非數字,而是獲取數字段作為最終數組中的元素。 像這樣嗎
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
public class Testing
{
public static void main(String[] args) throws IOException
{
try
{
//open a file
String path;
path = "TestNumbers.txt";
File f = new File(path);
FileReader re = new FileReader(f);
BufferedReader in = new BufferedReader(re);
String line = ""; //reads a line
String store = ""; //combines all the lines
while((line = in.readLine()) != null) //for each line
{
if(line.length() > 0){ //only add to store if the line contains something
store = store + line + " ";
}
}
//create the string array
String[] finalString = store.split(", ");
//and the integer array to hold the numbers
int [] intArray = new int [finalString.length];
//parse the string array and put each index to the integer array
for (int i = 0; i < finalString.length; i++){
intArray[i] = Integer.parseInt(finalString[i]);
}
//simply calculating the average
//summing up
int sum = 0;
for (int i = 0; i < intArray.length; i++){
sum = sum + intArray[i];
}
//printing the average
System.out.println("Average: " + sum / intArray.length);
}
catch (FileNotFoundException e)
{
e.printStackTrace();
}
}
}
您可以執行.replace以將所有“”字符交換為“,”字符。 如果您希望兩者都像“,”,則在某些情況下甚至可以先將它們替換為“,”。 這些基本上是技巧。
您可以看一下String.split(regex),因為正則表達式可以是“ [,] +”之類的模式,以數字之間的任意數量的空格和/或逗號作為分隔符。
這是我測試過的一個快速演示(跳過文件輸入,因為您似乎已經知道了):
public class tmp {
public static void main(String[] args) {
String input = "1,2, 3, 4\n5, 6, 7\n\n,8"; //what we would read from file
input = input.replace("\n"," "); //simulate removing newlines, ignore this
String[] items = input.split("[, ]+"); //regex split
for(String one : items) {
System.out.println(one);
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.