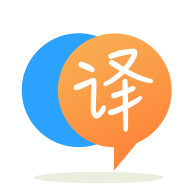
[英]How can I parse a period of date into a String[ ] day by day in Java?
[英]How can I increment a date by one day in Java?
我正在使用這種格式的日期: yyyy-mm-dd
。
如何將此日期增加一天?
像這樣的事情應該可以解決問題:
String dt = "2008-01-01"; // Start date
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar c = Calendar.getInstance();
c.setTime(sdf.parse(dt));
c.add(Calendar.DATE, 1); // number of days to add
dt = sdf.format(c.getTime()); // dt is now the new date
更新(2021 年 5 月):對於舊的 Java 來說,這是一個非常過時的答案。 對於 Java 8 及更高版本,請參閱https://stackoverflow.com/a/20906602/314283
與 C# 相比,Java 似乎確實遠遠落后於八球。 這個實用方法展示了在 Java SE 6 中使用Calendar.add 方法(大概是唯一簡單的方法)的方法。
public class DateUtil
{
public static Date addDays(Date date, int days)
{
Calendar cal = Calendar.getInstance();
cal.setTime(date);
cal.add(Calendar.DATE, days); //minus number would decrement the days
return cal.getTime();
}
}
要添加一天,根據所問的問題,請按如下方式調用:
String sourceDate = "2012-02-29";
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd");
Date myDate = format.parse(sourceDate);
myDate = DateUtil.addDays(myDate, 1);
在 Java 8 及更高版本上, java.time 包使這幾乎是自動的。 ( 教程)
假設String
輸入和輸出:
import java.time.LocalDate;
public class DateIncrementer {
static public String addOneDay(String date) {
return LocalDate.parse(date).plusDays(1).toString();
}
}
我更喜歡使用 Apache 的DateUtils 。 檢查這個http://commons.apache.org/proper/commons-lang/javadocs/api-2.6/org/apache/commons/lang/time/DateUtils.html 。 它非常方便,尤其是當您必須在項目中的多個地方使用它並且不想為此編寫一個 liner 方法時。
API 說:
addDays(Date date, int amount) :將天數添加到返回新對象的日期。
請注意,它返回一個新的 Date 對象,並且不會對前一個對象本身進行更改。
SimpleDateFormat dateFormat = new SimpleDateFormat( "yyyy-MM-dd" );
Calendar cal = Calendar.getInstance();
cal.setTime( dateFormat.parse( inputString ) );
cal.add( Calendar.DATE, 1 );
構造一個 Calendar 對象並使用方法 add(Calendar.DATE, 1);
Java 8 添加了一個用於處理日期和時間的新 API。
在 Java 8 中,您可以使用以下代碼行:
// parse date from yyyy-mm-dd pattern
LocalDate januaryFirst = LocalDate.parse("2014-01-01");
// add one day
LocalDate januarySecond = januaryFirst.plusDays(1);
看看 Joda-Time ( https://www.joda.org/joda-time/ )。
DateTimeFormatter parser = ISODateTimeFormat.date();
DateTime date = parser.parseDateTime(dateString);
String nextDay = parser.print(date.plusDays(1));
請注意,此行增加了 24 小時:
d1.getTime() + 1 * 24 * 60 * 60 * 1000
但這條線增加了一天
cal.add( Calendar.DATE, 1 );
在夏令時更改(25 或 23 小時)的日子里,您會得到不同的結果!
你可以使用簡單的 java.util lib
Calendar cal = Calendar.getInstance();
cal.setTime(yourDate);
cal.add(Calendar.DATE, 1);
yourDate = cal.getTime();
Date today = new Date();
SimpleDateFormat formattedDate = new SimpleDateFormat("yyyyMMdd");
Calendar c = Calendar.getInstance();
c.add(Calendar.DATE, 1); // number of days to add
String tomorrow = (String)(formattedDate.format(c.getTime()));
System.out.println("Tomorrows date is " + tomorrow);
這將給出明天的日期。 c.add(...)
參數可以從 1 更改為另一個數字以進行適當的增量。
如果您使用的是Java 8 ,那么就這樣做。
LocalDate sourceDate = LocalDate.of(2017, Month.MAY, 27); // Source Date
LocalDate destDate = sourceDate.plusDays(1); // Adding a day to source date.
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd"); // Setting date format
String destDate = destDate.format(formatter)); // End date
如果你想使用SimpleDateFormat ,那么就這樣做。
String sourceDate = "2017-05-27"; // Start date
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar calendar = Calendar.getInstance();
calendar.setTime(sdf.parse(sourceDate)); // parsed date and setting to calendar
calendar.add(Calendar.DATE, 1); // number of days to add
String destDate = sdf.format(calendar.getTime()); // End date
long timeadj = 24*60*60*1000;
Date newDate = new Date (oldDate.getTime ()+timeadj);
這需要從 oldDate 紀元以來的毫秒數,並添加 1 天的毫秒數,然后使用 Date() 公共構造函數使用新值創建日期。 此方法允許您添加 1 天或任意數量的小時/分鍾,而不僅僅是整天。
由於Java 1.5 TimeUnit.DAYS.toMillis(1)對我來說看起來更干凈。
SimpleDateFormat dateFormat = new SimpleDateFormat( "yyyy-MM-dd" );
Date day = dateFormat.parse(string);
// add the day
Date dayAfter = new Date(day.getTime() + TimeUnit.DAYS.toMillis(1));
在 Java 8 中,簡單的方法是:
Date.from(Instant.now().plusSeconds(SECONDS_PER_DAY))
很簡單,試圖用簡單的詞來解釋。 獲取今天的日期如下
Calendar calendar = Calendar.getInstance();
System.out.println(calendar.getTime());// print today's date
calendar.add(Calendar.DATE, 1);
現在通過采用(常量,值)的 calendar.add 方法將此日期提前一天。 這里常量可以是日期、小時、分鍾、秒等,value 是常量的值。 就像一天一樣,提前常量是 Calendar.DATE,它的值為 1,因為我們想要提前一天的值。
System.out.println(calendar.getTime());// print modified date which is
明天的日期
謝謝
startCalendar.add(Calendar.DATE, 1); //Add 1 Day to the current Calender
在 java 8 中你可以使用java.time.LocalDate
LocalDate parsedDate = LocalDate.parse("2015-10-30"); //Parse date from String
LocalDate addedDate = parsedDate.plusDays(1); //Add one to the day field
您可以將 in 轉換為java.util.Date
對象,如下所示。
Date date = Date.from(addedDate.atStartOfDay(ZoneId.systemDefault()).toInstant());
您可以將LocalDate
為字符串,如下所示。
String str = addedDate.format(DateTimeFormatter.ofPattern("yyyy-MM-dd"));
對於 Java SE 8 或更高版本,您應該使用新的日期/時間 API
int days = 7;
LocalDate dateRedeemed = LocalDate.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd/MM/YYYY");
String newDate = dateRedeemed.plusDays(days).format(formatter);
System.out.println(newDate);
如果您需要將java.util.Date
轉換為java.time.LocalDate
,您可以使用此方法。
public LocalDate asLocalDate(Date date) {
Instant instant = date.toInstant();
ZonedDateTime zdt = instant.atZone(ZoneId.systemDefault());
return zdt.toLocalDate();
}
對於 Java SE 8 之前的版本,您可以使用Joda-Time
Joda-Time 為 Java 日期和時間類提供了高質量的替代品,並且是 Java SE 8 之前 Java 的事實上的標准日期和時間庫
int days = 7;
DateTime dateRedeemed = DateTime.now();
DateTimeFormatter formatter = DateTimeFormat.forPattern("dd/MM/uuuu");
String newDate = dateRedeemed.plusDays(days).toString(formatter);
System.out.println(newDate);
Apache Commons 已經有這個 DateUtils.addDays(Date date, int amount) http://commons.apache.org/proper/commons-lang/apidocs/org/apache/commons/lang3/time/DateUtils.html#addDays%28java您使用的.util.Date,%20int%29或者您可以使用 JodaTime 使其更干凈。
只需在字符串中傳遞日期和接下來的天數
private String getNextDate(String givenDate,int noOfDays) {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
Calendar cal = Calendar.getInstance();
String nextDaysDate = null;
try {
cal.setTime(dateFormat.parse(givenDate));
cal.add(Calendar.DATE, noOfDays);
nextDaysDate = dateFormat.format(cal.getTime());
} catch (ParseException ex) {
Logger.getLogger(GR_TravelRepublic.class.getName()).log(Level.SEVERE, null, ex);
}finally{
dateFormat = null;
cal = null;
}
return nextDaysDate;
}
如果您想添加單個時間單位並且希望其他字段也增加,則可以安全地使用 add 方法。 請參閱下面的示例:
SimpleDateFormat simpleDateFormat1 = new SimpleDateFormat("yyyy-MM-dd");
Calendar cal = Calendar.getInstance();
cal.set(1970,Calendar.DECEMBER,31);
System.out.println(simpleDateFormat1.format(cal.getTime()));
cal.add(Calendar.DATE, 1);
System.out.println(simpleDateFormat1.format(cal.getTime()));
cal.add(Calendar.DATE, -1);
System.out.println(simpleDateFormat1.format(cal.getTime()));
將打印:
1970-12-31
1971-01-01
1970-12-31
試試這個方法:
public static Date addDay(int day) {
Calendar calendar = Calendar.getInstance();
calendar.setTime(new Date());
calendar.add(Calendar.DATE, day);
return calendar.getTime();
}
使用DateFormat
API 將String 轉換為Date 對象,然后使用Calendar
API 添加一天。 如果您需要特定的代碼示例,請告訴我,我可以更新我的答案。
其實很簡單。 一天包含 86400000 毫秒。 因此,首先您使用System.currentTimeMillis()
從 The System 獲取當前時間System.currentTimeMillis()
以毫秒為單位System.currentTimeMillis()
然后添加 84000000 毫秒,並使用Date
類生成毫秒的日期格式。
例子
String Today = new Date(System.currentTimeMillis()).toString();
字符串 今天將是 2019-05-9
String Tommorow = new Date(System.currentTimeMillis() + 86400000).toString();
弦樂明天將是 2019-05-10
String DayAfterTommorow = new Date(System.currentTimeMillis() + (2 * 86400000)).toString();
字符串 DayAfterTommorow 將是 2019-05-11
您可以使用來自“org.apache.commons.lang3.time”的這個包:
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Date myNewDate = DateUtils.addDays(myDate, 4);
Date yesterday = DateUtils.addDays(myDate, -1);
String formatedDate = sdf.format(myNewDate);
如果您使用的是 Java 8, java.time.LocalDate
和java.time.format.DateTimeFormatter
可以使這項工作變得非常簡單。
public String nextDate(String date){
LocalDate parsedDate = LocalDate.parse(date);
LocalDate addedDate = parsedDate.plusDays(1);
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-mm-dd");
return addedDate.format(formatter);
}
讓我們澄清一下用例:您想要進行日歷算術並以java.util.Date開始/結束。
一些方法:
考慮使用java.time.Instant :
Date _now = new Date();
Instant _instant = _now.toInstant().minus(5, ChronoUnit.DAYS);
Date _newDate = Date.from(_instant);
你可以在一行中做到這一點。
例如增加 5 天
Date newDate = Date.from(Date().toInstant().plus(5, ChronoUnit.DAYS));
減去 5 天
Date newDate = Date.from(Date().toInstant().minus(5, ChronoUnit.DAYS));
Date newDate = new Date();
newDate.setDate(newDate.getDate()+1);
System.out.println(newDate);
我認為最快的,永遠不會被棄用的,它是進入核心的
let d=new Date();
d.setTime(d.getTime()+86400000);
console.log(d);
它只有一行,只有 2 個命令。 它適用於日期類型,不使用日歷。
我一直認為最好在代碼方面使用 unix 時間,並在准備好向用戶顯示日期時顯示日期。
要打印日期 d,我使用
let format1 = new Intl.DateTimeFormat('en', { year: 'numeric', month: 'numeric', month: '2-digit', day: '2-digit'});
let [{ value: month },,{ value: day },,{ value: year }] = format1.formatToParts(d);
它設置 vars 月年和日,但可以擴展到小時分和秒,也可以根據國家標志用於標准表示。
在 Kotlin 中,您可以使用這 2 個函數來獲取當前日期和未來日期。
fun getCurrentDate(): String {
val currentDate = Calendar.getInstance().time
val dateFormat = SimpleDateFormat("yyyy-dd-MM", Locale.getDefault())
return dateFormat.format(currentDate)
}
fun incrementBy(days: Int): String {
val dateFormat = SimpleDateFormat("yyyy-dd-MM", Locale.getDefault())
val cal = Calendar.getInstance()
cal.time = dateFormat.parse(getCurrentDate())
cal.add(Calendar.DATE, days)
return dateFormat.format(cal.time)
}
通過調用 getCurrentDate(),輸出為
2021-26-07
並通過調用 incrementBy(3),輸出為
2021-29-07
我是初學者
嘗試獲取以下情況的代碼
Edittext1 =當前的蛋白質棒庫存Edittext2 =每日消耗的蛋白質棒
Textview1(剩余天數)=我還剩下多少天(edittext1 / editext2)
Textview2(重新訂購日期)=當前日期+ textview1-7天(交貨時間)
顯示重新訂購日期
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.