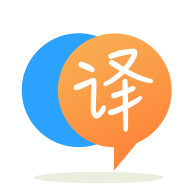
[英]How does this work - Java 8 Optional.of and Optional.ofNullable
[英]Java 8 flatMap + Optional.of doesn't compile
我正在嘗試在Java中使用flatMap
Optional
。 這是一個簡化的例子:
List<String> x = Arrays.asList("a", "b", "c");
List<String> result = x.stream().flatMap((val) -> val.equals("b") ? Optional.empty() : Optional.of(val)).collect(Collectors.toList());
我從編譯器收到此錯誤消息:
Error:(10, 27) java: incompatible types: no instance(s) of type variable(s) T exist so that java.util.Optional<T> conforms to java.util.stream.Stream<? extends R>
怎么了? 以下是我在Scala中嘗試實現的示例:
List("a", "b", "c").flatMap(x => if (x == "b") None else Some(x))
它返回:
res2: List[String] = List(a, c)
正如所料。
如何將其轉換為Java以便編譯?
flatMap
應該將輸入Stream
的元素映射到不同的Stream
。 因此,它必須返回Stream
而不是Optional
。
因此,你應該這樣做:
List<String> x = Arrays.asList("a", "b", "c");
List<Optional<String>> result =
x.stream()
.flatMap((val) ->
val.equals("b") ? Stream.of(Optional.empty()) :
Stream.of(Optional.of(val)))
.collect(Collectors.toList());
請注意,如果您的目標只是刪除某些值(示例中為“b”),則根本不需要使用Optional。 您只需過濾流:
List<String> result =
x.stream()
.filter (val -> !val.equals("b"))
.collect(Collectors.toList());
這樣您就不需要flatMap
,輸出是List<String>
而不是List<Optional<String>>
。
正如Holger評論的那樣,返回Stream
of Optional
的解決方案可以通過使用map
而不是flatMap
來簡化,因為每個元素都映射到一個Optional
:
List<String> x = Arrays.asList("a", "b", "c");
List<Optional<String>> result =
x.stream()
.map((val) -> val.equals("b") ? Optional.empty() : Optional.of(val))
.collect(Collectors.toList());
這里沒有必要處理Optional
。
最簡單的直接解決方案是使用filter
List<String> result = x.stream()
.filter(val -> !val.equals("b"))
.collect(Collectors.toList());
如果你堅持使用flatMap
,你應該只使用Stream
而不是Optional
:
List<String> result = x.stream().flatMap(
val -> val.equals("b")? Stream.empty(): Stream.of(val))
.collect(Collectors.toList());
如果必須處理不可避免地產生Optional
的操作,則必須將其轉換為Stream
以使用Stream.flatMap
:
List<String> result = x.stream()
.map(val -> val.equals("b") ? Optional.<String>empty() : Optional.of(val))
.flatMap(o->o.map(Stream::of).orElse(Stream.empty()))
.collect(Collectors.toList());
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.