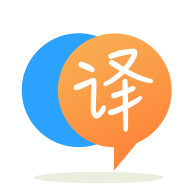
[英]parse a particular amount of data from json file using mongodb and java
[英]How can I import data to Mongodb from Json file using java
我正在努力將數據從Json
文件導入Mongodb
。
我可以使用mongoimport command
在命令行中執行相同的操作。
我探索並嘗試了很多,但無法使用 java 從 Json 文件中導入。
示例.json
{ "test_id" : 1245362, "name" : "ganesh", "age" : "28", "Job" :
{"company name" : "company1", "designation" : "SSE" }
}
{ "test_id" : 254152, "name" : "Alex", "age" : "26", "Job" :
{"company name" : "company2", "designation" : "ML" }
}
感謝您的時間。 ~甘尼什~
假設您可以分別讀取 JSON 字符串。 例如,您閱讀了第一個 JSON 文本
{ "test_id" : 1245362, "name" : "ganesh", "age" : "28", "Job" :
{"company name" : "company1", "designation" : "SSE" }
}
並賦值給一個變量(String json1),下一步就是解析它,
DBObject dbo = (DBObject) com.mongodb.util.JSON.parse(json1);
將所有dbo放入一個列表中,
List<DBObject> list = new ArrayList<>();
list.add(dbo);
然后將它們保存到數據庫中:
new MongoClient().getDB("test").getCollection("collection").insert(list);
編輯:
在最新的 MongoDB 版本中,您必須使用 Documents 而不是 DBObject,並且添加對象的方法現在看起來有所不同。 這是一個更新的示例:
進口是:
import com.mongodb.MongoClient;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
代碼是這樣的(參考編輯上面的文字):
Document doc = Document.parse(json1);
new MongoClient().getDataBase("db").getCollection("collection").insertOne(doc);
你也可以用列表的方式來做。 但是你需要
new MongoClient().getDataBase("db").getCollection("collection").insertMany(list);
但我認為這個解決方案有問題。 當你輸入:
db.collection.find()
在 mongo shell 中獲取集合中的所有對象,結果如下所示:
{ "_id" : ObjectId("56a0d2ddbc7c512984be5d97"),
"test_id" : 1245362, "name" : "ganesh", "age" : "28", "Job" :
{ "company name" : "company1", "designation" : "SSE"
}
}
這與以前不完全相同。
我自己也有類似的“問題”,最終將Jackson與POJO databinding和 Morphia 一起使用。
雖然這聽起來有點像用大錘敲碎堅果,但它實際上非常易於使用、健壯、性能良好且易於維護代碼明智。
小警告:如果您想重用它,您需要將test_id
字段映射到 MongoDB 的_id
。
您需要提示 Jackson 如何將數據從 JSON 文件映射到 POJO。 為了可讀性,我稍微縮短了課程:
@JsonRootName(value="person")
@Entity
public class Person {
@JsonProperty(value="test_id")
@Id
Integer id;
String name;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
至於嵌入的文檔Job
,請查看鏈接的 POJO 數據綁定示例。
在應用程序初始化期間的某個地方,您需要映射帶注釋的 POJO。 由於您已經應該有一個 MongoClient,我將重用它 ;)
Morphia morphia = new Morphia();
morphia.map(Person.class);
/* You can reuse this datastore */
Datastore datastore = morphia.createDatastore(mongoClient, "myDatabase");
/*
* Jackson's ObjectMapper, which is reusable, too,
* does all the magic.
*/
ObjectMapper mapper = new ObjectMapper();
現在導入給定的 JSON 文件變得非常簡單
public Boolean importJson(Datastore ds, ObjectMapper mapper, String filename) {
try {
JsonParser parser = new JsonFactory().createParser(new FileReader(filename));
Iterator<Person> it = mapper.readValues(parser, Person.class);
while(it.hasNext()) {
ds.save(it.next());
}
return Boolean.TRUE;
} catch (JsonParseException e) {
/* Json was invalid, deal with it here */
} catch (JsonMappingException e) {
/* Jackson was not able to map
* the JSON values to the bean properties,
* possibly because of
* insufficient mapping information.
*/
} catch (IOException e) {
/* Most likely, the file was not readable
* Should be rather thrown, but was
* cought for the sake of showing what can happen
*/
}
return Boolean.FALSE;
}
通過一些重構,這可以在 Jackson 注釋的 bean 的通用導入器中轉換。 顯然,我遺漏了一些特殊情況,但這超出了本答案的范圍。
使用 3.2 驅動程序,如果您有一個 mongo 集合和一個 json 文檔集合,例如:
MongoCollection<Document> collection = ...
List<String> jsons = ...
您可以單獨插入:
jsons.stream().map(Document::parse).forEach(collection::insertOne);
或批量:
collection.insertMany(
jsons.stream().map(Document::parse).collect(Collectors.toList())
);
運行時 r = Runtime.getRuntime();
進程 p = 空;
//dir 是 mongoimport 所在的路徑。
File dir=new File("C:/Program Files/MongoDB/Server/3.2/bin");
//這一行將在給定目錄中打開您的外殼,導入命令與您在命令提升中使用 mongoimport 完全相同
p = r.exec("c:/windows/system32/cmd.exe /c mongoimport --db mydb --collection student --type csv --file student.csv --headerline",null,dir);
我今天剛剛面對這個問題,並以另一種不同的方式解決了它,但這里沒有一個讓我滿意,所以請享受我的額外貢獻。 性能足以導出 30k 文檔並將它們導入我的 Springboot 應用程序以進行集成測試用例(需要幾秒鍾)。
首先,首先導出數據的方式很重要。 我想要一個文件,其中每行包含 1 個可以在我的 java 應用程序中解析的文檔。
mongo db --eval 'db.data.find({}).limit(30000).forEach(function(f){print(tojson(f, "", true))})' --quiet > dataset.json
然后我從我的資源文件夾中獲取文件,解析它,提取行,並使用 mongoTemplate 處理它們。 可以使用緩沖區。
@Autowired
private MongoTemplate mongoTemplate;
public void createDataSet(){
mongoTemplate.dropCollection("data");
try {
InputStream inputStream = Thread.currentThread().getContextClassLoader().getResourceAsStream(DATASET_JSON);
List<Document> documents = new ArrayList<>();
String line;
InputStreamReader isr = new InputStreamReader(inputStream, Charset.forName("UTF-8"));
BufferedReader br = new BufferedReader(isr);
while ((line = br.readLine()) != null) {
documents.add(Document.parse(line));
}
mongoTemplate.insert(documents,"data");
} catch (Exception e) {
throw new RuntimeException(e);
}
}
List<Document> jsonList = new ArrayList<Document>();
net.sf.json.JSONArray array = net.sf.json.JSONArray.fromObject(json);
for (Object object : array) {
net.sf.json.JSONObject jsonStr = (net.sf.json.JSONObject)JSONSerializer.toJSON(object);
Document jsnObject = Document.parse(jsonStr.toString());
jsonList.add(jsnObject);
}
collection.insertMany(jsonList);
public static void importCSV(String path) {
try {
List<Document> list = new ArrayList<>();
MongoDatabase db = DbConnection.getDbConnection();
db.createCollection("newCollection");
MongoCollection<Document> collection = db.getCollection("newCollection");
BufferedReader reader = new BufferedReader(new FileReader(path));
String line;
while ((line = reader.readLine()) != null) {
String[] item = line.split(","); // csv file is "" separated
String id = item[0]; // get the value in the csv assign keywords
String first_name = item[1];
String last_name = item[2];
String address = item[3];
String gender = item[4];
String dob = item[5];
Document document = new Document(); // create a document
document.put("id", id); // data into the database
document.put("first_name", first_name);
document.put("last_name", last_name);
document.put("address", address);
document.put("gender", gender);
document.put("dob", dob);
list.add(document);
}
collection.insertMany(list);
}catch (Exception e){
System.out.println(e);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.