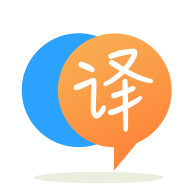
[英]In java I want to jump from a particular block of Statements to another block of statements. How can I achieve it?
[英]How to use, check and compare 'enter' key as an input from user, in my if statements. Using Java
我必須:更新帳戶信息:該程序應首先要求管理員輸入要更新的帳戶號:輸入帳戶號:15然后,應顯示一個菜單,其中顯示管理員所有的舊信息,然后執行以下步驟:分步查詢以更改特定字段。 如果他將字段留空,則傳播舊信息,即先前的信息保持不變。
問題:將字段保留為空白意味着按下回車鍵時。 我應該如何實現呢? 我嘗試使用char'10'/'13'以及'\\ n',但是它們不能與不同類型進行比較,例如string到char以及int到char。
Heres my code:
import java.util.*;
class Customers
{
private String name;
private int accountno;
private String status;
private String type;
private int currbal;
private String Login;
private int Pin;
public Customers()
{
this.name = " ";
this.accountno = accountno;
this.currbal = currbal;
}
public Customers( String name1, int A, int Bal)
{
name = name1;
accountno = A;
currbal = Bal;
}
public Customers ( String name1,int accountno1, String status1, String type1, int curr, String Login1, int Pin1)
{
name = name1;
accountno = accountno1;
status = status1;
type = type1;
currbal = curr;
Login = Login1;
Pin = Pin1;
}
public String getHolderName()
{
return name;
}
public int getaccountno()
{
return accountno;
}
public String getStatus()
{
return status;
}
public String getType()
{
return type;
}
public int getCurrentBalance()
{
return currbal;
}
public String getLogin()
{
return Login;
}
public int getPin()
{
return Pin;
}
public void setName( String N)
{
name = N;
}
public void setAccountno(int account )
{
accountno = account;
}
public void setStatus( String S)
{
status = S;
}
public void setType( String T)
{
type = T;
}
public void setCurrentBalance(int Bal )
{
currbal = Bal;
}
public void setLogin( String Log)
{
Login = log;
}
public void setPin( int Pinn )
{
Pin = Pinn;
}
}
class ATMmain
{
public static void main( String [] args)
{
Customers [] Carray = new Customers [100];
int count = 0;
System.out.println ("Begin session (Y/N)? ");
char ch = 'Y';
Scanner S = new Scanner(System.in);
ch = S.next().charAt(0);
while ( ch == 'Y' || ch == 'y' )
{
System.out.println(" 1----Create New Account.");
System.out.println("2----Delete Existing Account");
System.out.println("3----Update Account Information. ");
System.out.println(" 4----Search for Account.");
System.out.println(" 5----View Reports");
System.out.println(" 6----Exit");
//Scanner S = new Scanner(System.in);
int input = S.nextInt();
if ( input == 1)
{
//Customers [] C1 = new Customers[1];
//Customers [] C2 = new Customers[1];
System.out.println(createNewAccount(count, Carray));
count++;
}
if (input == 2)
{
System.out.println(DeleteExistingAcc(count, Carray));
count--;
}
System.out.println("Do you wish to do some action" );
ch = S.next().charAt(0);
if (ch != 'Y' || ch != 'y' )
{
System.out.println ("Thank you for your time");
}
}
}
public static int createNewAccount( int count, Customers [] Carray)
{
//Customers [] Carray = new Customers[10];
System.out.println("Enter account information");
Scanner S = new Scanner (System.in);
System.out.println("Login:");
String log = S.next();
System.out.println("Pin Code:");
int Pin = S.nextInt();
System.out.println("Holder's name: ");
String holderN = S.next();
System.out.println("Type:" );
String type = S.next();
if (!type.equals("Savings") && !type.equals("Current"))
{
System.out.println("error type! please Re-enter");
System.out.println("Type:" );
type = S.next();
}
System.out.println("Starting Balance:");
int SBal = S.nextInt();
System.out.println("Status:");
String Stat = S.next();
Customers ACC = new Customers(holderN, count,Stat, type,SBal,log, Pin );
Carray[count] = ACC;
System.out.println("Account Successfully created!");
System.out.println("account number is" +count);
//count++;
return 1;
}
public static int DeleteExistingAcc(int count, Customers [] Carray )
{
System.out.println ("Enter Account Number: ");
Scanner S = new Scanner(System.in);
int acc = S.nextInt();
System.out.println("Are you sure you want to delete this Account:" +acc);
String x = Carray[acc].getHolderName();
System.out.println("You wish to delete the account held by: " + x);
System.out.println("If this information is correct, please re-enter account number: " );
acc = S.nextInt();
int accnew = acc + 1;
Carray[acc] = Carray[accnew];
System.out.println ("Account successfully deleted");
//Carray.splice(acc,1, 0);
return 0;
}
public static void display( int acc, Customer [] arr)
{
arr[acc].getHolderName();
arr[acc].getLogin();
arr[acc].getCurrentBalance();
arr[acc].getPin();
arr[acc].getStatus();
arr[acc].getType();
arr[acc].getaccountno();
}
public static int UpdateAccountInfo( Customers [] Carray)
{
System.out.println("Enter Account Number:");
Scanner S = new Scanner(System.in);
int acc = S.nextInt();
display( acc, Carray);
char ent = '/n';
int ent1 = '/n';
Carray[acc].getHolderName();
String Str = S.next();
if ( Str != '\n') //See here
{
Carray[acc].setName( Str);
}
Carray[acc].getaccountno();
int account = S.nextInt();
if( account != '10') //here
{
Carray[acc].setAccountno(account);
}
Carray[acc].getType();
String type1 = S.next();
if ( type1 != '/n' ) //here
{
Carray[acc].setType( type1);
}
Carray[acc].getCurrentBalance();
int bal = S.nextInt();
if (bal != '13') //here
{
Carray[acc].setCurrentBalance( bal);
}
Carray[acc].getStatus();
String stat = S.next();
if ( stat != '13') //here
{
Carray[acc].setStatus( stat);
}
System.out.println("Your account has been successfully updated");
}
}
您可以將S.next()
替換為S.nextLine()
,並使用以下命令檢測空行(ENTER鍵):
String str = S.nextLine();
if (str.equals("") {
// empty line
}
我在您的代碼中看到的是,在UpdateAccountInfo()方法下,您已將String對象和int數據與char文字進行了比較。
在這里,您已將String與char進行了比較。 因此,該表達式將結果為false。
Carray[acc].getHolderName();
String Str = S.next();
if ( Str != '\n') //See here
{
Carray[acc].setName( Str);
}
在這里,int對抗char。
Carray[acc].getaccountno();
int account = S.nextInt();
if( account != '10') //here
{
Carray[acc].setAccountno(account);
}
在這里,再次是針對char的String。
Carray[acc].getType();
String type1 = S.next();
if ( type1 != '/n' ) //here
{
Carray[acc].setType( type1);
}
在這里,int對抗char。
Carray[acc].getCurrentBalance();
int bal = S.nextInt();
if (bal != '13') //here
{
Carray[acc].setCurrentBalance( bal);
}
在這里,再次是針對char的String。
Carray[acc].getStatus();
String stat = S.next();
if ( stat != '13') //here
{
Carray[acc].setStatus( stat);
}
嘗試以與獲取“是/否”和菜單選項選擇相同的方式進行數據比較。
好了,更好的選擇是讀取整行並檢查其是否為空(長度為零)。
Scanner S = new Scanner(System.in);
String input = S.nextLine();
if (input != null || input.trim().length() > 0) {
//do the update task
}
//otherwise you need not update the value
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.