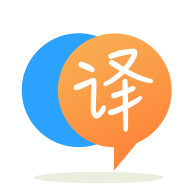
[英]how to get customer id from mysql and then to store it in a session variable to use the session variable in another file to retrieve information
[英]Get the ID of a button on click and store it as a session variable
我正在嘗試制作博客的小版本,但是在單擊附加到該按鈕的按鈕來從數據庫編輯某個帖子時,我遇到了麻煩。 我已經為每個與數據庫中的ID匹配的按鈕設置了ID,但是我現在要做的是將其存儲在會話變量中,這樣我就可以使用該ID從數據庫中獲取信息,從而可以進行更新數據庫中的信息。
這是帖子的樣子:
$result = $db->query("SELECT * FROM posts");
while($row = $result->fetch()) {
echo "<li><h1>" . $row['headline'] . "</h1></li>";
echo "<li><b>" . $row['content'] . "</b></li>";
echo "<li><h4>" . $row['author'] . "</h4></li>";
echo "<li><h5>" . $row['date'] . "</h5></li>";
echo "<input type='submit' name='edit' value='Edit' id='" . $row['id'] . "'>";
echo "<br>";
echo "<input type='submit' name='delete' value='delete' id='" . $row['id'] . "'>";
}
if (isset($_POST['edit'])) {
//Here is where I need to get the ID of the clicked edit button
//But I can't figure out how to do that in PHP
}
HTML表單元素的id
屬性在提交時不隨表單一起發送。 您想要做的是在表單中使用<input type="hidden" name="post_id" value="" />
元素來檢索帖子的ID。
將一個隱藏字段添加到您的窗體,稱為postId。
然后,將一個類添加到您的按鈕,然后在給定類中按下該按鈕中的任何一個時,使用jQuery您可以更新隱藏字段的值。
...
<input type="submit" name="edit" value="Edit" class="postButton" id="<?php echo $row["id"]; ?>" />
...
<input type="submit" name="edit" value="Edit" class="postButton" id="<?php echo $row["id"]; ?>" />
...
<input type="submit" name="edit" value="Edit" class="postButton" id="<?php echo $row["id"]; ?>" />
...
<input type="hidden" name="postId" value="" />
然后,您可以使用jQuery將ID傳遞給hiddenfield:
$('.postButton').click(function() {
$('#postId').val($(this).attr('id'));
});
或者使用鏈接來實現這一點,然后將其從css格式化為一個按鈕。
我建議使用帶有鏈接的編輯頁面:
<a href="/posts/12941/edit">edit post</a>
我不認為您只能在PHP中做到這一點。 您可能會為每個按鈕使用不同的name
屬性,並以此方式進行標識,盡管我不確定如何在不使用可怕的if/else
語句的情況下有效地檢測單擊了哪個按鈕。
做到這一點的另一種方法是使用帶有AJAX請求的jQuery
(或純Javascript,盡管我會建議jQuery)將單擊按鈕的id
發送到PHP腳本。
像這樣的東西:
jQuery的
$('.button').on('click', function(e){
e.preventDefault();
$.post("php_page.php", {id: $(this).attr('id')}, function(data){
//This is the success function, you can display a message or something
});
});
然后,在PHP中
if(!empty($_POST['id']))
$_SESSION['button_clicked'] = $_POST['id']
這是非常基本的,您需要更多的代碼才能使所有功能正常工作(例如:session_start(),jQuery中的文檔就緒函數),但這就是我要做的。
您也可以使用AJAX進行GET
請求,具體取決於您的代碼。
您可以使用JQUERY
獲取id
並可以在php
中將其設置為會話。
while($row = $result->fetch()) {
echo "<li><h1>" . $row['headline'] . "</h1></li>";
echo "<li><b>" . $row['content'] . "</b></li>";
echo "<li><h4>" . $row['author'] . "</h4></li>";
echo "<li><h5>" . $row['date'] . "</h5></li>";
echo "<input type='submit' class='edit' name='edit' value='Edit' data-id='your id goes here' id='" . $row['id'] . "'>";
echo "<br>";
echo "<input type='submit' name='delete' value='delete' id='" . $row['id'] . "'>";
}
JQUERY
$('.edit').on('click', function(){
var id = $(this).attr('data-id);
$.ajax({
type: 'post',
url: '/path/where /you want to set session',
data: {id : id},
success: function (res){
alert(res);
});
});
您可以通過以下方式實現
$result = $db->query("SELECT * FROM posts");
?>
<?php
echo "<li><h1>" . $row['headline'] . "</h1></li>";
echo "<li><b>" . $row['content'] . "</b></li>";
echo "<li><h4>" . $row['author'] . "</h4></li>";
echo "<li><h5>" . $row['date'] . "</h5></li>";
echo "<form action='".$_SERVER['PHP_SELF']."' method='post'><input type='hidden' name='id' value='".$row['id']."'>
<input type='submit' name='edit' value='Edit' id='" . $row['id'] . "'></form>";
echo "<br>";
echo "<form action='".$_SERVER['PHP_SELF']."' method='post'><input type='hidden' name='id' value='".$row['id']."'><input type='submit' name='delete' value='delete' id='" . $row['id'] . "'></form>";
}
if (isset($_POST['edit'])) {
$id=$_POST['id'];
$_SESSION['id']=$id;
}
<script>
function submitForm(idButton) {
document.getElementById('storedClickedButtonId').value = idButton;
document.forms["MyForm"].submit();
}
</script>
<form name="MyForm" action="tt.php" method="post">
<?php
while($row = $result->fetch()) {
echo "<li><h1>" . $row['headline'] . "</h1></li>";
echo "<li><b>" . $row['content'] . "</b></li>";
echo "<li><h4>" . $row['author'] . "</h4></li>";
echo "<li><h5>" . $row['date'] . "</h5></li>";
echo "<input type='button' name='edit' onClick='submitForm(this.id)' value='Edit' id='" . $row['id'] . "'>";
echo "<br>";
echo "<input type='button' name='delete' onClick='submitForm(this.id)' value='delete' id='" . $row['id'] . "'>";
echo "<input type='hidden' id='storedClickedButtonId' name='clickedButtonId' value='' />";
}
?>
</form>
您將需要將每個部分包裝在自己的表單標簽中。 然后將ID設置為隱藏字段,然后將您的提交按鈕更改為具有相同的ame但值不同(在本例中為操作-編輯/刪除)。
然后,您可以從隱藏字段中檢索值,並根據所按下的提交按鈕的值來忽略該值:
while($row = $result->fetch()) {
echo "<li><h1>" . $row['headline'] . "</h1></li>";
echo "<li><b>" . $row['content'] . "</b></li>";
echo "<li><h4>" . $row['author'] . "</h4></li>";
echo "<li><h5>" . $row['date'] . "</h5></li>";
echo "<form method='post'>"; //form tag
echo "<input type='hidden' name='deleteid' value=" . $row['id'] . ">"; //get id
echo "<input type='submit' name='action' value='edit' id='" . $row['id'] . "'>";//pick action
echo "<br>";
echo "<input type='submit' name='action' value='delete' id='" . $row['id'] . "'>";//pick action
echo "/form>"
}
if (isset($_POST['action'])) {
$id=$_POST['deleteid'];//get id
if($_POST['action']=='edit'){//do edit}
else if($_POST['action']=='delete'){//do delete}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.