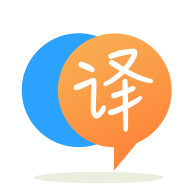
[英]Hybrid QuickSort + Insertion sort java.lang.StackOverflowError
[英]Java - How to implement a hybrid QuickSort and Insertion sort method
當分區大小低於某個閾值(使用下面的10)時,嘗試在quickSort時使用插入排序對混合的quickSort進行編碼。 我似乎無法正常工作。 數組將總是返回一些亂序的數字。
這是我的部分代碼:
public static void quickSort(int[] list) {
quickSort(list, 0, list.length - 1);
}
private static void quickSort(int[] list, int first, int last) {
int size = (last+1) - first;
if (size <= 10){ // insertion sort if 10 or smaller
insertionSort(list, first, size);
}
else // quicksort if large
{
int pivotIndex = partition(list, first, last);
quickSort(list, first, pivotIndex - 1);
quickSort(list, pivotIndex + 1, last);
}
}
public static void insertionSort(int[] list, int first, int size) {
for (int i = first+1; i < size; i++) {
int currentElement = list[i];
int k;
for (k = i - 1; k >= 0 && list[k] > currentElement; k--) {
list[k + 1] = list[k];
}
// Insert the current element into list[k+1]
list[k + 1] = currentElement;
}
}
預期輸出:以升序排列的隨機數組。
樣本輸出包含錯誤:9 18 34 36 53 61 87 89 117 115 109 120 129 154 163 136 131 164 175 193 206 182 182 243 243 181 165 216 261 274 276 281 320 338 341 322 322 379 372 382 392 397 419 401 401 479 479 508 512 494 518 558 578 588 606 660 657 665 617 674 698 728 683 692 684 685 737 738 741 745 753 777 799 816 824 791 791 823 823 762 761 825 845 833 854 854 860 934 886 933 938 880 890 989 935 939 970 948 953945968977995
問題是當您調用插入排序時
int size = (last+1) - first;
if (size <= 10){ // insertion sort if 10 or smaller
insertionSort(list, first, size);
}
假設在某個位置上first = 5,last = 7,所以size =2。您最終將調用insertSort(list,5,2)
因此,在您的insertSort()方法中,初始的for循環將如下所示:
for (int i = 5+1; i < 2; i++) {
什么時候應該看起來像:
for (int i = 5+1; i < 7; i++) {
我沒有對其進行測試,但是看起來這就是問題所在。
通過更改以下內容使其生效:
private static void quickSort(int[] list, int first, int last) {
int size = (last +1) - first;
if (first < last){
if (last < 11){ // insertion sort if 10 or smaller
insertionSort(list, first, size);
}
else // quicksort if large
{
int pivotIndex = partition(list, first, last);
quickSort(list, first, pivotIndex - 1);
quickSort(list, pivotIndex + 1, last);
}
}
}
我沒有評論,因為我還沒有50名代表。 我認為您的解決方案不會像您認為的那樣起作用。 您將最終僅對前10個元素運行插入排序。
返回您的初始代碼,並將您的調用更改為insertSort(list,first,size); 到:insertSort(list,first,last);
好吧,我做了這項工作...
第一種更改方法quickSort:
private static void quickSort(int[] list, int first, int last) {
int size = (last+1) - first;
if (first < last){
if (size <= 10){
insertionSort(list, first, last); //Changed this line
}
else{
int pivotIndex = partition(list, first, last);
quickSort(list, first, pivotIndex); //Changed this line just because i used the partition method from Hoare and not Lomuto
quickSort(list, pivotIndex + 1, last);
}
}
}
然后在方法insertSort中:
public static void insertionSort(int[] list, int first, int last) {
for (int i = first+1; i <= last; i++) { // Change i <= last
int currentElement = list[i];
int j = i-1;
while (j>=0 && list[j]>currentElement) {
list[j+1] = list[j];
j--;
}
list[j+1] = currentElement;
}
}
希望將是您想要的答案。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.