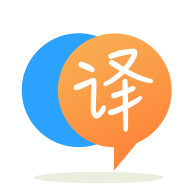
[英]Need to create a program that in PYTHON that takes the average of test scores and determines a letter grade on them
[英]Python program that takes test scores and outputs the following:
我必須編寫一個程序,該程序接受用戶輸入的測試分數並輸出,所有輸入的分數,平均分數,最大和最低分數,所有超過50的分數以及所有超過平均分數的分數。 我在未識別分數的地方出現錯誤,我不確定在調用列表時我做錯了什么? 我對編程還是很陌生,並且在調用函數方面苦苦掙扎。 謝謝。
def main():
test = getscores()
avg = average(test)
top = large(test)
over = passed(test)
greater = above(test, avg)
print("The test scores are,", test)
print("The average test score is,", avg)
print("The largest and lowest score are," top)
print("All the PASS scores are,", over)
print("All the scores above average are,", greater)
def getscores():
scores = []
xStr = input("Enter a test score in the range 0-100: (<Enter> to quit)")
while xStr != "":
x = eval(xStr)
scores.append(x)
xStr = input("Enter a test score int he range 0-100: (<Enter> to quit)")
return scores
def average(scores):
sum = 0.0
for i in scores:
sum = sum + scores
avg = sum / i
return avg
def large(scores):
largest = max(scores)
lowest = min(scores)
return largest, lowest
def passed(scores):
for i in scores:
PASS = i > 50
return PASS
def above(scores, avg):
high = scores > avg
return high
main()
這里有一個列表:
你可能不希望調用eval()
輸入值,當int()
是一個更好的選擇。 前者用於評估任意表達式,例如1 + 2
或variable * 7
。
另外,如果您使用的是Python 2,則需要raw_input()
而不是input()
。
在您的average
函數中,無需計算平均值,直到獲得完整的總和。 最重要的是,您試圖添加一個帶有列表scores
的整數sum
,而您應該添加scores
的元素i
。 重寫為:
def average(scores):
sum = 0.0
for i in scores:
sum = sum + i
avg = sum / len(scores)
return avg
由於large()
返回兩個值,並且您要打印兩個值,因此應將其調用為:
(top, bottom) = large(test)
並使用類似以下內容進行打印:
print("The largest and lowest score are ", top, " and ", bottom)
您的passed()
函數將返回單個真值,而不是通過的人數。 它可以寫成:
def passed(scores):
quant = 0
for i in scores:
if i > 50:
quant = quant + 1
return quant
與above()
函數相同,這將更好:
def above(scores,avg):
quant = 0
for i in scores:
if i > avg:
quant = quant + 1
return quant
完成所有這些更改后,您將看到以下內容:
Enter a test score in the range 0-100: (<Enter> to quit)52
Enter a test score int he range 0-100: (<Enter> to quit)45
Enter a test score int he range 0-100: (<Enter> to quit)8
Enter a test score int he range 0-100: (<Enter> to quit)
('The test scores are,', [52, 45, 8])
('The average test score is,', 35.0)
('The largest and lowest score are', 52, 'and', 8)
('All the PASS scores are,', 1)
('All the scores above average are,', 2)
如果要返回通過和高於平均分數的實際列表 ,則可以像輸入分數時一樣,構造一個列表並將其追加到列表中:
def passed(scores):
slist = []
for i in scores:
if i > 50:
slist.append(i)
return slist
def above(scores,avg):
slist = []
for i in scores:
if i > avg:
slist.append(i)
return slist
或者更好的是,重構,因此您只需要一個:
def above(scores,threshold):
slist = []
for i in scores:
if i > threshold:
slist.append(i)
return slist
over = above(test,50)
greater = above(test, avg)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.