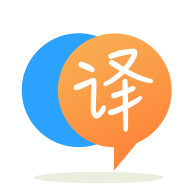
[英]How to Import Google doc text into Email draft using Google Apps Script
[英]How to send a draft email using google apps script
我正在使用谷歌應用程序腳本,並希望創建一個腳本,從草稿中提取郵件,如果標簽為“明天發送”,則發送它們。 查找帶有特定標簽的草稿非常簡單:
var threads = GmailApp.search('in:draft label:send-tomorrow');
但是我沒有看到用於發送消息的API! 我看到的唯一選擇是: - 打開消息 - 提取正文/附件/ title / from / to / cc / bcc - 發送帶有上述參數的新消息 - 銷毀之前的草稿
這看起來很煩人,我不確定它是否適用於嵌入式圖像,多個附件等...
任何提示?
我看到的唯一選擇是: - 打開消息 - 提取正文/附件/ title / from / to / cc / bcc - 發送帶有上述參數的新消息 - 銷毀之前的草稿
這是Amit Agarawal撰寫的這篇博客的主題。 他的腳本完全符合您的描述,但不處理內聯圖像。 對於那些,您可以調整本文中的代碼。
但是你是對的 - 如果你不能發送愚蠢的東西,甚至有一條草稿信息的重點是什么?!
我們可以使用GMail API Users.drafts:從Google Apps腳本發送以發送草稿。 以下獨立腳本執行此操作,並處理必要的授權。
完整的腳本可以在這個要點中找到 。
/*
* Send all drafts labeled "send-tomorrow".
*/
function sendDayOldDrafts() {
var threads = GmailApp.search('in:draft label:send-tomorrow');
for (var i=0; i<threads.length; i++) {
var msgId = threads[0].getMessages()[0].getId();
sendDraftMsg( msgId );
}
}
/**
* Sends a draft message that matches the given message ID.
* Throws if unsuccessful.
* See https://developers.google.com/gmail/api/v1/reference/users/drafts/send.
*
* @param {String} messageId Immutable Gmail Message ID to send
*
* @returns {Object} Response object if successful, see
* https://developers.google.com/gmail/api/v1/reference/users/drafts/send#response
*/
function sendDraftMsg( msgId ) {
// Get draft message.
var draftMsg = getDraftMsg(msgId,"json");
if (!getDraftMsg(msgId)) throw new Error( "Unable to get draft with msgId '"+msgId+"'" );
// see https://developers.google.com/gmail/api/v1/reference/users/drafts/send
var url = 'https://www.googleapis.com/gmail/v1/users/me/drafts/send'
var headers = {
Authorization: 'Bearer ' + ScriptApp.getOAuthToken()
};
var params = {
method: "post",
contentType: "application/json",
headers: headers,
muteHttpExceptions: true,
payload: JSON.stringify(draftMsg)
};
var check = UrlFetchApp.getRequest(url, params)
var response = UrlFetchApp.fetch(url, params);
var result = response.getResponseCode();
if (result == '200') { // OK
return JSON.parse(response.getContentText());
}
else {
// This is only needed when muteHttpExceptions == true
var err = JSON.parse(response.getContentText());
throw new Error( 'Error (' + result + ") " + err.error.message );
}
}
/**
* Gets the current user's draft messages.
* Throws if unsuccessful.
* See https://developers.google.com/gmail/api/v1/reference/users/drafts/list.
*
* @returns {Object[]} If successful, returns an array of
* Users.drafts resources.
*/
function getDrafts() {
var url = 'https://www.googleapis.com/gmail/v1/users/me/drafts';
var headers = {
Authorization: 'Bearer ' + ScriptApp.getOAuthToken()
};
var params = {
headers: headers,
muteHttpExceptions: true
};
var check = UrlFetchApp.getRequest(url, params)
var response = UrlFetchApp.fetch(url, params);
var result = response.getResponseCode();
if (result == '200') { // OK
return JSON.parse(response.getContentText()).drafts;
}
else {
// This is only needed when muteHttpExceptions == true
var error = JSON.parse(response.getContentText());
throw new Error( 'Error (' + result + ") " + error.message );
}
}
/**
* Gets the draft message ID that corresponds to a given Gmail Message ID.
*
* @param {String} messageId Immutable Gmail Message ID to search for
*
* @returns {String} Immutable Gmail Draft ID, or null if not found
*/
function getDraftId( messageId ) {
if (messageId) {
var drafts = getDrafts();
for (var i=0; i<drafts.length; i++) {
if (drafts[i].message.id === messageId) {
return drafts[i].id;
}
}
}
// Didn't find the requested message
return null;
}
/**
* Gets the draft message content that corresponds to a given Gmail Message ID.
* Throws if unsuccessful.
* See https://developers.google.com/gmail/api/v1/reference/users/drafts/get.
*
* @param {String} messageId Immutable Gmail Message ID to search for
* @param {String} optFormat Optional format; "object" (default) or "json"
*
* @returns {Object or String} If successful, returns a Users.drafts resource.
*/
function getDraftMsg( messageId, optFormat ) {
var draftId = getDraftId( messageId );
var url = 'https://www.googleapis.com/gmail/v1/users/me/drafts'+"/"+draftId;
var headers = {
Authorization: 'Bearer ' + ScriptApp.getOAuthToken()
};
var params = {
headers: headers,
muteHttpExceptions: true
};
var check = UrlFetchApp.getRequest(url, params)
var response = UrlFetchApp.fetch(url, params);
var result = response.getResponseCode();
if (result == '200') { // OK
if (optFormat && optFormat == "JSON") {
return response.getContentText();
}
else {
return JSON.parse(response.getContentText());
}
}
else {
// This is only needed when muteHttpExceptions == true
var error = JSON.parse(response.getContentText());
throw new Error( 'Error (' + result + ") " + error.message );
}
}
要使用Google的API,我們需要為當前用戶提供OAuth2令牌 - 就像我們為高級服務所做的那樣。 這是使用ScriptApp.getOAuthToken()
。
將代碼復制到您自己的腳本后,打開資源 - >高級Google服務,打開Google Developers Console的鏈接,然后為您的項目啟用Gmail API。
只要腳本包含至少一個需要用戶權限的GMailApp方法,就會為OAuthToken正確設置身份驗證范圍。 在此示例中,由GmailApp.search()
中的sendDayOldDrafts()
; 但是對於保險,您可以使用API直接在函數中包含不可訪問的函數調用。
我是使用GmailMessage.forward方法完成的。
它適用於上傳圖像和附件,但我必須設置主題以避免前綴“Fwd:”和用戶名,因為它只向收件人顯示用戶電子郵件。
我沒有找到處理草稿的方法,所以我只是刪除標簽以防止再次發送它。
腳本:
function getUserFullName(){
var email = Session.getActiveUser().getEmail();
var contact = ContactsApp.getContact(email);
return contact.getFullName();
}
function testSendTomorrow(){
var threads = GmailApp.search('in:draft label:send-tomorrow');
if(threads.length == 0){
return;
}
var labelSendTomorrow = GmailApp.getUserLabelByName("send-tomorrow");
for(var i = 0; i < threads.length; i++){
var messages = threads[i].getMessages();
for(var j = 0; j < messages.length; j++){
var mssg = messages[j];
if(mssg.isDraft()){
mssg.forward(mssg.getTo(), {
cc: mssg.getCc(),
bcc: mssg.getBcc(),
subject: mssg.getSubject(),
name: getUserFullName()
});
}
}
threads[i].removeLabel(labelSendTomorrow);
}
}
您可以搜索所有草稿,然后發送該特定草稿沒問題。
function sendMessage(id){
GmailApp.getDrafts().forEach(function (draft) {
mes = draft.getMessage()
if (mes.getId() == id) {
draft.send()
}
})
}
更簡單的替代方法是使用gmail api而不是gmailApp:
function sendtestDraft(draftId){
var request = Gmail.Users.Drafts.send({id : draftId},'me');
Logger.log(request);
}
上面的函數示例在https://script.google.com的gs腳本中使用。 它需要draftId(而不是消息Id),草稿將被發送。 圖像和附件都可以! 信息: https : //developers.google.com/gmail/api/v1/reference/users/drafts/send
我是新來的,沒有足夠的“聲譽”來評論,所以無法對Mogsdad的原始答案發表評論,所以我不得不創建一個新的答案:
我已經改編了Mogsdad的解決方案,也支持回復/轉發現有線程,而不僅僅是全新的消息。
要在現有線程上使用它,首先應創建回復/轉發,然后僅標記線程。 我的代碼還支持多個標簽並設置這些標簽。
我為它創建了一個新的要點,在這里分享Mogsdad's: https ://gist.github.com/hadasfester/81bfc5668cb7b666b4fd6eeb6db804c3
我仍然需要在文檔中添加一些屏幕截圖鏈接,否則這已經可以使用了,我自己一直在使用它。 希望你覺得它有用。
同樣在這里內聯:
/** * This script allows you to mark threads/drafts with a predetermined label and have them get sent the next time your trigger * sets off. * * Setup instructions: * 1. Make a copy of this script (File -> Make a copy) * 2. Follow the "Authorization" instructions on https://stackoverflow.com/a/27215474. (If later during setup/testing you get * another permissions approval dialog, approve there as well). * 2. I created two default labels, you can edit/add your own. See "TODO(user):" below. After that, to create them in gmail, * choose "setUpLabel" function above and click the play button (TODO: screenshot). Refresh your gmail tab, you should see * the new labels. * 3. Click the clock icon above (TODO: screenshot) and set time triggers, eg like so: (TODO: screenshot) * 4. I recommend also setting up error notifications: (TODO: screenshot). * * Testing setup: * When you're first setting this up, if you want to test it, create a couple * of drafts and label them. Then, in this * script, select "sendWeekStartDrafts" or "sendTomorrowDrafts" in the function dropdown * and press play. This manually triggers the script (instead of relying on the * timer) so you can see how it works. * * Usage instructions: * 1. To get a draft sent out on the next trigger, mark your draft with the label you chose. * NOTE: If your draft is a reply to a thread, make sure you first create the draft and only then set the label on the * thread, not the other way around. * That's it! Upon trigger your draft will be sent and the label will get removed from the thread. * * Some credits and explanation of differences/improvements from other existing solutions: * 1. This script was adapted from https://stackoverflow.com/a/27215474 to also support replying existing threads, not only * sending brand new messages. * 2. Other solutions I've run into are based on creating a new message, copying it field-by-field, and sending the new one, * but those have many issues, some of which are that they also don't handle replies and forwards very elegantly. * * Enjoy! **/ var TOMORROW_LABEL = '!send-tomorrow'; var WEEK_START_LABEL = '!send-week-start'; // TODO(user): add more labels here. /** * Set up the label for delayed send! **/ function setUpLabels() { GmailApp.createLabel(TOMORROW_LABEL); GmailApp.createLabel(WEEK_START_LABEL); // TODO(user): add more labels here. } function sendTomorrowDrafts() { sendLabeledDrafts(TOMORROW_LABEL); } function sendWeekStartDrafts() { sendLabeledDrafts(WEEK_START_LABEL); } // TODO(user): add more sendXDrafts() functions for your additional labels here. /* * Send all drafts labeled $MY_LABEL. * @param {String} label The label for which to send drafts. */ function sendLabeledDrafts(label) { var threads = GmailApp.search('in:draft label:' + label); for (var i=0; i<threads.length; i++) { var thread = threads[i]; var messages = thread.getMessages(); var success = false; for (var j=messages.length-1; j>=0; j--) { var msgId = messages[j].getId(); if (sendDraftMsg( msgId )) { success = true; } } if (!success) { throw Error( "Failed sending msg" ) }; if (success) { var myLabel = GmailApp.getUserLabelByName(label); thread.removeLabel(myLabel); } } } /** * Sends a draft message that matches the given message ID. * See https://developers.google.com/gmail/api/v1/reference/users/drafts/send. * * @param {String} messageId Immutable Gmail Message ID to send * * @returns {Object} Response object if successful, see * https://developers.google.com/gmail/api/v1/reference/users/drafts/send#response */ function sendDraftMsg( msgId ) { // Get draft message. var draftMsg = getDraftMsg(msgId,"json"); if (!getDraftMsg(msgId)) return null; // see https://developers.google.com/gmail/api/v1/reference/users/drafts/send var url = 'https://www.googleapis.com/gmail/v1/users/me/drafts/send' var headers = { Authorization: 'Bearer ' + ScriptApp.getOAuthToken() }; var params = { method: "post", contentType: "application/json", headers: headers, muteHttpExceptions: true, payload: JSON.stringify(draftMsg) }; var check = UrlFetchApp.getRequest(url, params) var response = UrlFetchApp.fetch(url, params); var result = response.getResponseCode(); if (result == '200') { // OK return JSON.parse(response.getContentText()); } else { // This is only needed when muteHttpExceptions == true return null; } } /** * Gets the current user's draft messages. * Throws if unsuccessful. * See https://developers.google.com/gmail/api/v1/reference/users/drafts/list. * * @returns {Object[]} If successful, returns an array of * Users.drafts resources. */ function getDrafts() { var url = 'https://www.googleapis.com/gmail/v1/users/me/drafts'; var headers = { Authorization: 'Bearer ' + ScriptApp.getOAuthToken() }; var params = { headers: headers, muteHttpExceptions: true }; var check = UrlFetchApp.getRequest(url, params) var response = UrlFetchApp.fetch(url, params); var result = response.getResponseCode(); if (result == '200') { // OK return JSON.parse(response.getContentText()).drafts; } else { // This is only needed when muteHttpExceptions == true var error = JSON.parse(response.getContentText()); throw new Error( 'Error (' + result + ") " + error.message ); } } /** * Gets the draft message ID that corresponds to a given Gmail Message ID. * * @param {String} messageId Immutable Gmail Message ID to search for * * @returns {String} Immutable Gmail Draft ID, or null if not found */ function getDraftId( messageId ) { if (messageId) { var drafts = getDrafts(); for (var i=0; i<drafts.length; i++) { if (drafts[i].message.id === messageId) { return drafts[i].id; } } } // Didn't find the requested message return null; } /** * Gets the draft message content that corresponds to a given Gmail Message ID. * See https://developers.google.com/gmail/api/v1/reference/users/drafts/get. * * @param {String} messageId Immutable Gmail Message ID to search for * @param {String} optFormat Optional format; "object" (default) or "json" * * @returns {Object or String} If successful, returns a Users.drafts resource. */ function getDraftMsg( messageId, optFormat ) { var draftId = getDraftId( messageId ); var url = 'https://www.googleapis.com/gmail/v1/users/me/drafts'+"/"+draftId; var headers = { Authorization: 'Bearer ' + ScriptApp.getOAuthToken() }; var params = { headers: headers, muteHttpExceptions: true }; var check = UrlFetchApp.getRequest(url, params) var response = UrlFetchApp.fetch(url, params); var result = response.getResponseCode(); if (result == '200') { // OK if (optFormat && optFormat == "JSON") { return response.getContentText(); } else { return JSON.parse(response.getContentText()); } } else { // This is only needed when muteHttpExceptions == true return null; } }
首先, GmailDraft
現在有一個你可以直接調用的send()
函數。 請參閱: https : //developers.google.com/apps-script/reference/gmail/gmail-draft#send()
他們的代碼示例:
var draft = GmailApp.getDrafts()[0]; // The first draft message in the drafts folder
var msg = draft.send(); // Send it
Logger.log(msg.getDate()); // Should be approximately the current timestamp
其次,谷歌已經發布預定發送,甚至可能都不需要它。
Send
旁邊的箭頭
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.