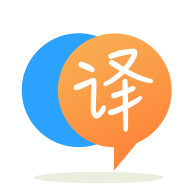
[英]Count the number of times a letter occurs in a word(string) in c using recursion
[英]Specified letter count with recursion
我正在嘗試編寫一種遞歸算法,該算法可對用戶指定的字母進行計數。 但是,我陷入了兩種情況。 首先,我認為我必須得到2
,我不能。 其次,如果沒有限制鍵,例如用戶指定為z
的限制字符,那么在g
如何掃描字符直到結束字符? 這個問題對我來說有點復雜。 我需要您的建議和想法。 謝謝大家贊賞的答案。
示例字符串是: how are you i am testing
另一個例子:
#include <stdio.h>
int lettercount(char* str, char key, char limit);
int main(){
char test[]="how are you i am testing";
int num;
num=lettercount(test,'a','t');
printf("%d",num);
return 0;
}
int lettercount(char* str, char key, char limit)
{
int count = 0;
if(str[0] == limit)
{
return 0;
}
else if(str[0] == key)
{
lettercount(&str[1], key, limit);
count++;
}
else
lettercount(&str[1], key, limit);
return count;
}
as the code is unwinding from the recursion(s)
it needs to accumulate the count
the following code should work for your needs.
Note: this returns 0 if key and limit are the same char
int lettercount(char* str, char key, char limit)
{
int count = 0;
if(str[0] == limit)
{
return 0;
}
// implied else, more char in string to check
if(str[0] == key)
{
count++;
}
count += lettercount(&str[1], key, limit);
return count;
} // end function: lettercount
使用遞歸函數,您需要三件事。 (1)在准備下一次調用的功能中設置 ; (2) 遞歸調用 ; (3) 一種終止遞歸的方法。 這是一種方法。 注意:以下代碼中的版本是長版本,以提高可讀性,最后包含一個簡短版本:
#include <stdio.h>
/* recursively find the number of occurrences
of 'c' in 's' (n is provided as '0')
*/
int countchar (char *s, char c, int n)
{
char *p = s;
if (!*p)
return n;
if (*p == c)
n = countchar (p+1, c, n+1);
else
n = countchar (p+1, c, n);
return n;
}
int main (int argc, char **argv) {
if (argc < 3) {
fprintf (stderr, "\n error: insufficient input. Usage: %s <string> <char>\n\n", argv[0]);
return 1;
}
int count = countchar (argv[1], *argv[2], 0);
printf ("\n There are '%d' '%c's in: %s\n\n", count, *argv[2], argv[1]);
return 0;
}
輸出:
$ ./bin/rec_c_in_s "strings of s'es for summing" s
There are '5' 's's in: strings of s'es for summing
您可以使用以下方法使函數更短,但可讀性稍差:
int countchar (char *s, char c, int n)
{
char *p = s;
if (!*p) return n;
return countchar (p+1, c, (*p == c) ? n+1 : n);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.