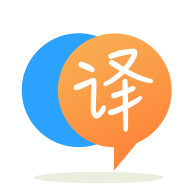
[英]Assigning a 2D array (of pointers) to a variable in an object for access in C++?
[英]2D Array of Object pointers in C++
如何為對象分配2D數組指針?
目前我有:
file.h
extern ClassName **c;
file.cpp
ClassName **c;
int main(){
// some stuff
c = new ClassName*[sizex];
for(int i = 0; i < sizex; ++i){
c[i] = new ClassName[sizey];
}
for(int i = 0; i < sizex; ++i){
for(int j = 0; j < sizey; ++j){
c[i][j] = new ClassName();
}
}
哪個沒有編譯錯誤,說明運算符=使用ClassName和ClassName *沒有匹配,查看錯誤是有意義的。 但是,如果我要將c [i] [j]的賦值更改為
ClassName cn();
c[i][j] = cn;
它給出了許多其他錯誤。 在運行時(從stdin讀取)之前無法知道數組的大小,它也必須是extern。 在這種情況下聲明數組的正確方法是什么?
你必須聲明指針
extern ClassName ***c;
分配看起來像
c = new ClassName**[sizex];
for(int i = 0; i < sizex; ++i){
c[i] = new ClassName*[sizey];
for(int j = 0; j < sizey; ++j){
c[i][j] = new ClassName();
}
}
如果要聲明某個抽象類型T
的數組,您可以自己正確定義2D數組。 然后你需要的是將T
改為ClassName *
至於這個宣言
ClassName cn();
然后它聲明一個返回類型ClassName且沒有參數的函數。
ClassName *p1;
p1
可以指向一個ClassName
或陣列ClassName
秒。
ClassName **p2;
p2
可以指向一個ClassName*
或ClassName*
s的數組。
*p2
可以指向一個ClassName
或陣列ClassName
秒。
當你使用:
c[i] = new ClassName[sizey];
你正在分配內存,以便c[i][j]
可以擁有ClassName
但不能擁有ClassName*
。
如果c[i][j] = ClassName();
失敗,你想使用c[i][j] = new ClassName();
,然后c
必須聲明為:
ClassName*** c;
但是,我強烈建議使用std::vector
和智能指針。
std::vector<std::vector<std::unique_ptr<ClassName>>> c;
以前的海報已正確回答了使用tripple指針的問題,但為了您的理智和代碼的清晰度,您可以使用幾個簡單的typedef:
typedef ClassName* Row;
typedef Row* Matrix;
Matrix *M; //equivalent to : ClassName ***M,
//check by substiting Matrix with Row* and Row with ClassName*
int main()
{
M = new Matrix[numRows];
for(int row = 0; row < numRows; ++row)
{
M[row] = new Row[numCols];
for(int col = 0; col < numCols; ++j)
{
M[row][col] = new ClassName();
}
}
}
這可以更好地傳達您的意圖,並且更容易推理。
您需要將類型更改為:ClassName *** c; 正如莫斯科的弗拉德所說。
ClassName **c;
int main(){
// some stuff
c = new ClassName**[sizex];
for(int i = 0; i < sizex; ++i){
c[i] = new ClassName*[sizey]
}
for(int i = 0; i < sizex; ++i){
for(int j = 0; j < sizey; ++j){
c[i][j] = new ClassName();
}
}
您的用法也必須改變:
ClassName cn;
c[i][j] = new ClassName( cn ); // <-- this copy constructor would cause a memory leak (old pointer not deleted)
*(c[i][j]) = cn; // <-- this would use the assignment operator. May be weak performance.
ClassName & cn = *(c[i][]); // <-- Allows you to work directly on the cell.
ClassName **c
是一個2D數組,即ClassName
對象的c[n][m]
。
您試圖將ClassName
類型的c[n][m]
指定為new ClassName()
類型的new ClassName()
ClassName*
for(int i = 0; i < sizex; ++i){
for(int j = 0; j < sizey; ++j){
c[i][j] = *(new ClassName);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.