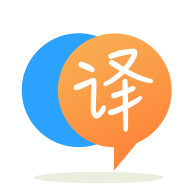
[英]How to convert ArrayList<String[]> to multidimensional array String[][]?
[英]How to convert an ArrayList to String array with ordered elements?
我正在嘗試編寫一種方法,該方法將采用Student對象的ArrayList並返回一個String數組,其中包含按分數順序排列的學生姓名(得分最高的學生姓名將在索引0處)。
public static void orderStudent(List<Student> ls) {
for (Student stu : ls) {
System.out.println("Name: " + stu.getName() + ", Score: "
+ stu.getScore());
}
}
上面的代碼片段在執行時將打印如下內容
Name: Alex, Score: 10.35
Name: Bob, Score: 11.2
Name: Charles, Score: 8.22
我希望orderStudent方法返回一個String數組,該數組的內容為[Bob,Alex,Charles] Bob是頭號得分手,其次是Alex和Charles。
如果Student
實現了Comparable
接口並按得分排序,則只需對列表進行排序,並構建名稱數組。
如果您無法編輯Student
類,則需要編寫一個實現Comparator<Student>
的類,並使用該類對列表進行排序,然后構建名稱數組。
簡化@PrasadKhode的答案:
public static String[] orderStudent(List<Student> list) {
String[] students = new String[list.size()];
Collections.sort(list, new Comparator<Student>() {
@Override
public int compare(Student object1, Student object2) {
return Integer.compare(object2.getScore(), object1.getScore());
}
});
for (int index = 0; index < list.size(); index++) {
Student student = list.get(index);
students[index] = student.getName();
System.out.println("Name: " + student.getName());
}
return students;
}
無需為每個得分比較創建一個CompareToBuilder
實例。 效率低下。
首先,您需要對列表進行排序,然后構造String []
您可以使用CompareToBuilder
類, 而無需對現有的POJO類進行任何更改 。
在CompareToBuilder上,您需要添加用於對集合進行排序的屬性。 您可以看到以下代碼:
import org.apache.commons.lang3.builder.CompareToBuilder;
...
public static String[] orderStudent(List<Student> list) {
String[] students = new String[list.size()];
Collections.sort(list, new Comparator<Student>() {
@Override
public int compare(Student object1, Student object2) {
return new CompareToBuilder().append(object2.getScore(), object1.getScore()).toComparison();
}
});
for (int index = 0; index < list.size(); index++) {
Student student = list.get(index);
students[index] = student.getName();
System.out.println("Name: " + student.getName());
}
return students;
}
您需要使用按分數排序的自定義Comporator對對象進行排序(必需)
Collections.sort(列表列表,比較器c)
遍歷排序的集合並打印
您需要在Student
類中實現兩件事: Comparable
和.toString()
方法的重寫。 首先,定義您的學生班級,例如:
public class Student implements Comparable<Student>
然后,您必須按以下方式定義.compareTo()
方法:
//used for sorting later
public int compareTo(Student other) {
return this.getScore().compareTo(other.getScore());//Assuming Student.score is not a primitive type
}
還要重寫.toString()
方法,如下所示:
//used for printing later
public string toString() {
return "Name: " + this.getName() + ", Score: " + this.getScore().toString();
}
現在,您只需將orderStudents
函數更改為以下內容:
public static void orderStudent(List<Student> ls) {
// this will sort your collection based on the logic in the `Student.compareTo()` method.
ls = Collections.sort(ls);
for (Student stu : ls) {
//this will print the student object based on the `Student.toString()` method
System.out.println(stu);
}
}
用列表替換數組:
public static List<String> orderStudent(List<Student> ls) {
Collections.sort(ls, new Comparator<Student>() {
@Override
public int compare(Student o1, Student o2) {
return o2.getScore().compareTo(o1.getScore());
}
});
List<String> result = new ArrayList<String>();
for(Student s : ls) {
result.add(s.getName());
}
return result;
}
如果使用Java 8,則少於兩行...
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.