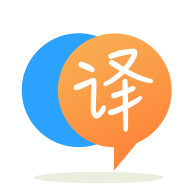
[英]How do I filter out an iterator's values where calling a function returns a Result::Err?
[英]How can I filter an iterator when the predicate returns a Result<bool, _>?
我希望迭代迭代器,但我的謂詞有可能失敗。 當謂詞失敗時,我想使整個函數失敗。 在這個例子中,我想work
返回maybe
生成的Result
:
fn maybe(v: u32) -> Result<bool, u8> {
match v % 3 {
0 => Ok(true),
1 => Ok(false),
2 => Err(42),
}
}
fn work() -> Result<Vec<u32>, u8> {
[1, 2, 3, 4, 5].iter().filter(|&&x| maybe(x)).collect()
}
fn main() {
println!("{:?}", work())
}
error[E0308]: mismatched types
--> src/main.rs:10:45
|
10 | [1, 2, 3, 4, 5].iter().filter(|&&x| maybe(x)).collect()
| ^^^^^^^^ expected bool, found enum `std::result::Result`
|
= note: expected type `bool`
found type `std::result::Result<bool, u8>`
error[E0277]: the trait bound `std::result::Result<std::vec::Vec<u32>, u8>: std::iter::FromIterator<&u32>` is not satisfied
--> src/main.rs:10:55
|
10 | [1, 2, 3, 4, 5].iter().filter(|&&x| maybe(x)).collect()
| ^^^^^^^ a collection of type `std::result::Result<std::vec::Vec<u32>, u8>` cannot be built from an iterator over elements of type `&u32`
|
= help: the trait `std::iter::FromIterator<&u32>` is not implemented for `std::result::Result<std::vec::Vec<u32>, u8>`
您可以將Result<bool, u8>
轉換為Option<Result<u32, u8>>
,即將bool
拉出到Option
並將值放入其中,然后使用filter_map
:
fn maybe(v: u32) -> Result<bool, u8> {
match v % 3 {
0 => Ok(true),
1 => Ok(false),
_ => Err(42),
}
}
fn work() -> Result<Vec<u32>, u8> {
[1, 2, 3, 4, 5]
.iter()
.filter_map(|&x| match maybe(x) {
Ok(true) => Some(Ok(x)),
Ok(false) => None,
Err(e) => Some(Err(e)),
})
.collect()
}
fn main() {
println!("{:?}", work())
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.