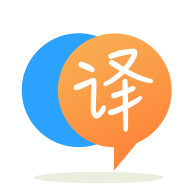
[英]Why i get [Getter/Setter] on first object in console.log?
[英]How can I get console.log to output the getter result instead of the string "[Getter/Setter]"?
在這段代碼中:
function Cls() {
this._id = 0;
Object.defineProperty(this, 'id', {
get: function() {
return this._id;
},
set: function(id) {
this._id = id;
},
enumerable: true
});
};
var obj = new Cls();
obj.id = 123;
console.log(obj);
console.log(obj.id);
我想得到 { _id: 123, id: 123 } 但我得到的是 { _id: 123, id: [Getter/Setter] }
有沒有辦法讓 console.log 函數使用 getter 值?
您可以使用console.log(Object.assign({}, obj));
使用console.log(JSON.stringify(obj));
您可以在您的對象上定義一個inspect
方法,並導出您感興趣的屬性。請參閱此處的文檔: https : //nodejs.org/api/util.html#util_custom_inspection_functions_on_objects
我想它看起來像:
function Cls() {
this._id = 0;
Object.defineProperty(this, 'id', {
get: function() {
return this._id;
},
set: function(id) {
this._id = id;
},
enumerable: true
});
};
Cls.prototype.inspect = function(depth, options) {
return `{ 'id': ${this._id} }`
}
var obj = new Cls();
obj.id = 123;
console.log(obj);
console.log(obj.id);
從 Nodejs v11.5.0 開始,您可以在util.inspect
選項中設置getters: true
。 有關文檔,請參見此處。
getters <boolean> | <string> 如果設置為 true,則檢查 getter。 如果設置為 'get',則只檢查沒有相應設置器的 getter。 如果設置為“set”,則僅檢查具有相應設置器的 getter。 這可能會導致副作用,具體取決於 getter 函數。 默認值:假。
我需要一個漂亮的打印對象,沒有 getter 和 setter,但純 JSON 會產生垃圾。 對我來說,在將JSON.stringify()
給一個特別大的嵌套對象后,JSON 字符串太長了。 我希望它在控制台中看起來和行為都像一個普通的字符串化對象。 所以我只是再次解析它:
JSON.parse(JSON.stringify(largeObject))
那里。 如果你有更簡單的方法,請告訴我。
在 Node.js 上,我建議使用util.inspect.custom ,這將允許您將 getter 漂亮地打印為值,同時保持其他屬性輸出不變。
它僅適用於您的特定對象,不會弄亂一般的 console.log 輸出。
與Object.assign
的主要好處是它發生在您的對象上,因此您可以保留常規的通用console.log(object)
語法。 您不必用console.log(Object.assign({}, object))
包裝它。
將以下方法添加到您的對象中:
[util.inspect.custom](depth, options) {
const getters = Object.keys(this);
/*
for getters set on prototype, use instead:
const prototype = Object.getPrototypeOf(this);
const getters = Object.keys(prototype);
*/
const properties = getters.map((getter) => [getter, this[getter]]);
const defined = properties.filter(([, value]) => value !== undefined);
const plain = Object.fromEntries(defined);
const object = Object.create(this, Object.getOwnPropertyDescriptors(plain));
// disable custom after the object has been processed once to avoid infinite looping
Object.defineProperty(object, util.inspect.custom, {});
return util.inspect(object, {
...options,
depth: options.depth === null ? null : options.depth - 1,
});
}
這是您的上下文中的一個工作示例:
const util = require('util');
function Cls() {
this._id = 0;
Object.defineProperty(this, 'id', {
get: function() {
return this._id;
},
set: function(id) {
this._id = id;
},
enumerable: true
});
this[util.inspect.custom] = function(depth, options) {
const getters = Object.keys(this);
/*
for getters set on prototype, use instead:
const prototype = Object.getPrototypeOf(this);
const getters = Object.keys(prototype);
*/
const properties = getters.map((getter) => [getter, this[getter]]);
const defined = properties.filter(([, value]) => value !== undefined);
const plain = Object.fromEntries(defined);
const object = Object.create(this, Object.getOwnPropertyDescriptors(plain));
// disable custom after the object has been processed once to avoid infinite looping
Object.defineProperty(object, util.inspect.custom, {});
return util.inspect(object, {
...options,
depth: options.depth === null ? null : options.depth - 1,
});
}
};
var obj = new Cls();
obj.id = 123;
console.log(obj);
console.log(obj.id);
輸出:
Cls { _id: 123, id: 123 }
123
使用傳播運算符 console.log({... obj });
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.