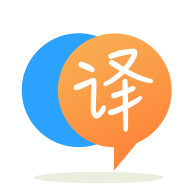
[英]Is there a way of adjusting the width of a <select> according to the length of the selected <option> within?
[英]Adjust width of select element according to selected option's width
我希望 select 元素具有更短的默認選項值的寬度。 當用戶選擇另一個選項時,select 元素應調整自身大小,以便整個文本始終在元素中可見。
我在Auto resizing the SELECT element under selected OPTION's width問題中找到了解決方案,但它不適用於 bootstrap,我不知道為什么。
這是我的嘗試:
$(document).ready(function() { $('select').change(function(){ $("#width_tmp").html($('select option:selected').text()); // 30 : the size of the down arrow of the select box $(this).width($("#width_tmp").width()+30); }); });
.btn-select { color: #333; background-color: #f5f5f5; border-color: #ccc; padding:7px; } select { width: 60px; } #width_tmp{ display : none; }
<link href="//cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.2/css/bootstrap.css" rel="stylesheet"/> <link href="//cdnjs.cloudflare.com/ajax/libs/font-awesome/4.3.0/css/font-awesome.css" rel="stylesheet"/> <script src="//cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.js"></script> <script src="//cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.2/js/bootstrap.js"></script> <div class="form-group"> <div class="input-group"> <span class="input-group-btn"> <select class="btn btn-select align-left"> <option>All</option> <option>Longer</option> <option>A very very long string...</option> </select> <span id="width_tmp"></span> </span> <input type="text" class="form-control" placeholder="Search by..."> <span class="input-group-btn"> <button class="btn btn-default" type="button"> <i class="fa fa-search"></i> </button> </span> </div> </div>
出現此問題是因為#width_tmp
嵌套在.input-group-btn
因此它繼承了font-size: 0;
來自引導程序的屬性。 因此,元素的計算寬度始終為零:
要解決此問題,只需移動<span id="width_tmp"></span>
使其不在輸入組內。
// resize on initial load
document.querySelectorAll("select").forEach(resizeSelect)
// delegated listener on change
document.body.addEventListener("change", (e) => {
if (e.target.matches("select")) {
resizeSelect(e.target)
}
})
function resizeSelect(sel) {
let tempOption = document.createElement('option');
tempOption.textContent = sel.selectedOptions[0].textContent;
let tempSelect = document.createElement('select');
tempSelect.style.visibility = "hidden";
tempSelect.style.position = "fixed"
tempSelect.appendChild(tempOption);
sel.after(tempSelect);
sel.style.width = `${+tempSelect.clientWidth + 4}px`;
tempSelect.remove();
}
// resize on initial load document.querySelectorAll("select").forEach(resizeSelect) // delegated listener on change document.body.addEventListener("change", (e) => { if (e.target.matches("select")) { resizeSelect(e.target) } }) function resizeSelect(sel) { let tempOption = document.createElement('option'); tempOption.textContent = sel.selectedOptions[0].textContent; let tempSelect = document.createElement('select'); tempSelect.style.visibility = "hidden"; tempSelect.style.position = "fixed" tempSelect.appendChild(tempOption); sel.after(tempSelect); sel.style.width = `${+tempSelect.clientWidth + 4}px`; tempSelect.remove(); }
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <select> <option value="2">Some longer option</option> <option value="1">Short option</option> <option value="3">An very long option with a lot of text</option> </select> <select> <option>Short option 2</option> <option>Some longer option 2</option> <option selected>An very long option with a lot of text 2</option> </select>
另一種選擇是使用插件來處理從頭到尾的整個操作。 這是我剛剛提出的一個。
該插件會動態創建和銷毀span
因此它不會弄亂您的 html。 這有助於分離關注點,並讓您將該功能委托給另一個文件,並輕松地跨多個頁面重復使用多個元素。
(function($, window){
$(function() {
let arrowWidth = 30;
$.fn.resizeselect = function(settings) {
return this.each(function() {
$(this).change(function(){
let $this = $(this);
// get font-weight, font-size, and font-family
let style = window.getComputedStyle(this)
let { fontWeight, fontSize, fontFamily } = style
// create test element
let text = $this.find("option:selected").text();
let $test = $("<span>").html(text).css({
"font-size": fontSize,
"font-weight": fontWeight,
"font-family": fontFamily,
"visibility": "hidden" // prevents FOUC
});
// add to body, get width, and get out
$test.appendTo($this.parent());
let width = $test.width();
$test.remove();
// set select width
$this.width(width + arrowWidth);
// run on start
}).change();
});
};
// run by default
$("select.resizeselect").resizeselect();
})
})(jQuery, window);
您可以通過以下兩種方式之一初始化插件:
HTML - 將類.resizeselect
添加到任何選擇元素:
<select class="btn btn-select resizeselect "> <option>All</option> <option>Longer</option> <option>A very very long string...</option> </select>
JavaScript - 在任何 jQuery 對象上調用.resizeselect()
:
$("select"). resizeselect ()
(function($, window){ $(function() { let arrowWidth = 30; $.fn.resizeselect = function(settings) { return this.each(function() { $(this).change(function(){ let $this = $(this); // get font-weight, font-size, and font-family let style = window.getComputedStyle(this) let { fontWeight, fontSize, fontFamily } = style // create test element let text = $this.find("option:selected").text(); let $test = $("<span>").html(text).css({ "font-size": fontSize, "font-weight": fontWeight, "font-family": fontFamily, "visibility": "hidden" // prevents FOUC }); // add to body, get width, and get out $test.appendTo($this.parent()); let width = $test.width(); $test.remove(); // set select width $this.width(width + arrowWidth); // run on start }).change(); }); }; // run by default $("select.resizeselect").resizeselect(); }) })(jQuery, window);
.btn-select { color: #333; background-color: #f5f5f5; border-color: #ccc; padding:7px; }
<link href="//cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.2/css/bootstrap.css" rel="stylesheet"/> <link href="//cdnjs.cloudflare.com/ajax/libs/font-awesome/4.3.0/css/font-awesome.css" rel="stylesheet"/> <script src="//cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.js"></script> <script src="//cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.2/js/bootstrap.js"></script> <div class="form-group"> <div class="input-group"> <span class="input-group-btn"> <select class="btn btn-select resizeselect"> <option>All</option> <option>Longer</option> <option>A very very long string...</option> </select> </span> <input type="text" class="form-control" placeholder="Search by..."> <span class="input-group-btn"> <button class="btn btn-default" type="button"> <i class="fa fa-search"></i> </button> </span> </div> </div>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.