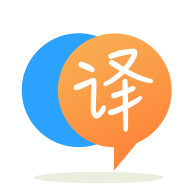
[英]How to return the largest integer in an Array that has 10 random integers in it?
[英]How do I write a random array of 5 integers but have it not count 0 as an integer?
我正在嘗試編寫一個程序,該程序會隨機選擇1到6之間的5個整數。然后,我需要該程序顯示缺少的整數。 我不知道如何不將“ 0”顯示為整數。 這是我到目前為止所擁有的...
import java.util.Random;
public class Task6
{
public static void main(String[] args)
{
int[] numbers = new int[5];
Random random = new Random();
for(int n = 1; n < 5; n++)
{
int select = random.nextInt(n + 1); //shuffle generator so it will not duplicate numbers
numbers[n] = numbers[select];
numbers[select] = n;
}//end for statement
for(int number : numbers)
{
System.out.println("Numbers selected : " + number);
}//end for
}
}
我也必須在其中進行O(n ^ 2)操作。
如果我理解正確,您是否希望您的隨機數僅在1到6(含)之間? 如果是這種情況,則需要使用類似於以下的代碼來限制RNG實際吐出的范圍:
/**
* Returns a pseudo-random number between min and max, inclusive.
* The difference between min and max can be at most
* <code>Integer.MAX_VALUE - 1</code>.
*
* @param min Minimum value
* @param max Maximum value. Must be greater than min.
* @return Integer between min and max, inclusive.
* @see java.util.Random#nextInt(int)
*/
public static int randInt(int min, int max) {
// NOTE: Usually this should be a field rather than a method
// variable so that it is not re-seeded every call.
Random rand = new Random();
// nextInt is normally exclusive of the top value,
// so add 1 to make it inclusive
int randomNum = rand.nextInt((max - min) + 1) + min;
return randomNum;
}
另外,您的for循環:
for(int n = 1; n < 5; n++) { ... }
不會生成5個數字,而是會生成4個數字。考慮一下循環的約束; 它會以n = 1、2、3、4運行一次,然后停止(5不小於5)。
如果要進行5次迭代,可以執行以下操作:
for (int n = 0; n < 5; n++) { ... }.
或這個:
for (int n = 1; n <= 5; n++) { ... }
您的迭代次數和隨機數范圍不需要關聯。
如果需要,請查看此出色的答案以獲取更多詳細信息。
創建將您的約束應用於隨機數的方法。 這是一個例子。
// this assumes that only 0 is unacceptable.
private static int myRandomBlammy()
{
int returnValue;
do
{
returnValue = blam; // replace blam with some way of generating a random integer.
} while (returnValue == 0);
return returnValue;
}
您應該在循環中使用if語句來遍歷數字。
for(int number : numbers)
{
if(number != 0){
System.out.println("Numbers selected : " + number);
}
}//end for
我正在嘗試編寫一個程序,該程序會隨機選擇1到6之間的5個整數。然后,我需要該程序顯示缺少的整數。 我不知道如何不將“ 0”顯示為整數。
根據您的問題,可能會出現5個隨機整數並非全部都是唯一的情況,並且可能有多個未生成的唯一數字。
我將使用一個數組來處理此問題,該數組計算每個數字生成多少個:
import java.util.Random;
public class RandomCounter
{
/**
* An example that uses array indices to count how many random
* numbers are generated in a range.
*/
public static void main(String[] args)
{
//use an array of size n + 1 (ignore the zero index)
int[] numbers = new int[7];
Random r = new Random();
//generate random numbers
for (int i = 1; i < 6; i++){
int next = r.nextInt(6) + 1;
numbers[next]++; //count each number at the index
}
//print any numbers that didn't occur at least once.
for(int i = 1; i < numbers.length; i++){
if(numbers[i] != 0){
System.out.println(i);
}
}
}
}
將此代碼段替換為最后一個for循環,以查看每個數字發生了多少:
//print how many of each number occurred.
for(int i = 1; i < numbers.length; i++){
System.out.println (i + ": " + numbers[i]); }
}
數組索引計數是一種動態計數數字出現的有用方法。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.