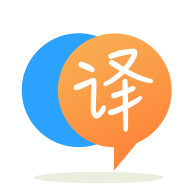
[英]C Programming: Reading data from a file, dynamically allocating memory, placing contents in struct array
[英]Dynamically allocating array of struct from file C
我正在嘗試編寫一個程序,該程序將打開一個名為hw3.data的文件,該文件將在每行中包含一個字符串,一個浮點數,一個int和一個字符串。 示例:someword 5.4 200000 someword我不知道文件的大小或文件中字符串的大小。 我需要動態分配結構數組以存儲信息。 我是C語言的新手,曾經研究過其他各種問題和文章,但是這些問題和文章都沒有真正幫助我掌握如何解決這個問題。 我認為解決此問題的最佳方法是首先靜態聲明一個結構。 讀取文件的長度,然后從靜態結構中獲取信息,然后動態分配。 到目前為止,這是我的代碼:
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
//Static Array of Struct
struct record{
char name[100];
float hp;
int size;
char color[30];
};
//getData from file
int getData(FILE*, struct record[], int currSize){
fp = fopen("hw3.data","r");
if (fp !=NULL){
printf("file Open");
while(3==fscanf(fp, "[^,],%f,%d,[^,]", records[i].name &records[i].hp, &records[i].size, records[i].color)){
currSizef++;
}
} else {
printf("failed");
}
return 0;
}
//First sorting function to sort by FLOAT value
//Second sorting function to sort by INT value
int main()
{
int currSizef=0;
struct record *records;
FILE* fp = NULL;
int choice;
//menu
do
{
printf("\t\tMenu\n");
printf("Options:\n");
printf("Input a number to select option:\n");
printf("1-Sort floats high to low\n 2-Sort floats low to high\n");
printf("3-Sort intergers high to low\n 4-Sort intergers low to high\n");
printf("5-Exit\n");
scanf("%d", &choice);
switch (choice)
{
case 1: /*Print high to low floats*/
break;
case 2: /*print low to high floats*/
break;
case 3: /*print high to low ints*/
break;
case 4: /*print low to high ints*/
break;
}
}while(choice !=5);
return 0;
}
您可以嘗試將數據加載到臨時記錄中,並使用realloc
動態擴展data
大小。
注意:如果將NULL
傳遞給realloc
則realloc
行為類似於指定大小的malloc
函數。
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
//Static Array of Struct
typedef struct record{
char name[100];
float hp;
int size;
char color[30];
}RECORD;
void record_copy(RECORD *dest, RECORD* src) {
strcpy(dest->name,src->name);
dest->hp = src->hp;
dest->size = src->size;
strcpy(dest->color,src->color);
}
//LoadData from file
RECORD* LoadData(FILE* fp,int *size){
int i = 0;
RECORD temp;
RECORD *data= NULL,*tempptr = NULL;
while( fscanf(fp, "%[^,],%f,%d,%[^,],", temp.name,&temp.hp,&temp.size,temp.color)==4 ) {
if( (tempptr = (RECORD*) realloc( data, sizeof(RECORD) * (i+1) )) )
data = tempptr;
else
return (NULL);
record_copy(&data[i],&temp);
i++;
}
*size = i;
return (data);
}
void record_sort(RECORD *data, int size) {
int i,j;
RECORD temp;
for (i = 0 ; i < ( size - 1 ); ++i)
for (j = 0 ; j < (size - i - 1); ++j )
if( data[j].hp > data[j+1].hp ) {
record_copy(&temp,&data[i]);
record_copy(&data[i],&data[i+1]);
record_copy(&data[i+1],&temp);
}
}
int main() {
FILE *fp = fopen("<fileName>","r");
RECORD *data = NULL;
int i;
int size;
if( fp != NULL ) {
data = LoadData(fp,&size);
}
record_sort(data,size);
for(i = 0;i<size;i++) {
printf("%s:%f:%d:%s\n",data[i].name,data[i].hp,data[i].size,data[i].color);
}
free(data);
return 0;
}
關於排序,您可以使用任何排序算法對記錄進行排序。 我給出了一個例子。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.