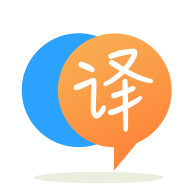
[英]How to change instance variable when another related instance variable is changed in a class?
[英]How to change an instance variable of a class when that variable is inside a list
我正在用Python編寫井字游戲,有一部分,我在一個類中有很多實例變量,它們都在列表中。 我試圖更改一個實例變量,但是當它在列表中時,我只能更改列表元素。
這里有一些代碼:
# only a piece of my code
class Board(object):
def __init__(self):
self.one = "1"
self.two = "2"
self.three = "3"
board = Board()
configs = [board.one, board.two, board.three]
configs[2] = "X"
print board.three
print configs
預期結果:
X
['1', '2', 'X']
實際結果:
3
['1', '2', 'X']
有沒有辦法獲得我的預期結果?
字符串是不可變的對象,因此當您使用給定索引更改列表中的項目時,列表現在指向完全獨立的字符串X
同樣的情況在這里適用;
>>> configs[2] += "X"
>>> print configs[2]
'3X'
>>> print board.three
'3'
一種替代方法是每當列表中的項目被更新時執行回調函數。 (但是,我個人不建議這樣做,因為這似乎是一個棘手的解決方案。)
class interactive_list(list):
def __init__(self, *args, **kwargs):
self.callback = kwargs.pop('callback', None)
super(interactive_list, self).__init__(*args, **kwargs)
def __setitem__(self, index, value):
super(interactive_list, self).__setitem__(index, value)
if self.callback:
self.callback(index, value)
>>> board = Board()
>>> def my_callback(index, value):
... if index == 2:
... board.three = value
>>> configs = interactive_list([board.one, board.two, board.three], callback=my_callback)
>>> configs[2] = 'X'
>>> print board.three
'X'
您是否考慮過使用更好的數據結構? 像字典一樣
class Board(object):
def __init__(self):
self.dict = {"one":"1", "two":"2", "three":"3"}
然后您可以執行以下操作:
>>> a = Board()
>>> a.dict
{'three': '3', 'two': '2', 'one': '1'}
>>> for element in a.dict:
a.dict[element] = element+"x"
>>> a.dict
{'three': 'threex', 'two': 'twox', 'one': 'onex'}
>>> a.dict["one"] = "1"
>>> a.dict
{'three': 'threex', 'two': 'twox', 'one': '1'}
您正在尋找的解決方案也是可能的(最有可能帶有一些非常奇怪的getattrs
等...,我真的不推薦使用。
Edit1結果(檢查后)表明您的類屬性將存儲在object.__dict__
。 所以為什么不使用自己的。
還要澄清一下,可以通過定義諸如以下的__getitem__
和__setitem__
方法來使用您自己的類來模擬容器對象:
class Board(object):
def __init__(self):
self.dict = {"one":"1", "two":"2", "three":"3"}
def __getitem__(self,key):
return self.dict[key]
def __setitem__(self, key, value):
self.dict[key] = value
這意味着您不必到處都寫a.dict
,並且可以假裝您的類是像下面這樣的容器(dict):
>>> a = Board()
>>> a.dict
{'three': '3', 'two': '2', 'one': '1'}
>>> a["one"]
'1'
>>> a["one"] = "x"
>>> a.dict
{'three': '3', 'two': '2', 'one': 'x'}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.