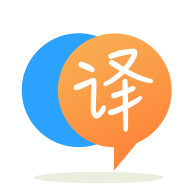
[英]Converting number of days(int) into days,months and years in java (including leap year)
[英]Converting a float number to years, months, weeks, days
我一直在嘗試將浮點數轉換為年,月,周,天,小時,分鍾和秒,但我不明白。
例如,如果用戶輸入768.96,則總計為2年,1個月,1周,1天,23小時,0分鍾和2秒。
這就是我所擁有的。
import javax.swing.JOptionPane;
public class timePartition {
public static void main(String[] args) {
float totalTime;
float userInput;
int years = 0, months = 0, weeks = 0, days = 0, hours = 0, minutes = 0, seconds = 0;
do{
userInput = Float.parseFloat(JOptionPane.showInputDialog("Enter a positive number to decompose"));
totalTime = userInput;
years = (int) userInput / 365;
userInput = userInput % 10;
months = (int) userInput / 12;
userInput = userInput % 10;
weeks = (int) userInput / 4;
userInput = userInput % 10;
days = (int) userInput / 30;
userInput = userInput % 10;
hours = (int) userInput / 24;
userInput = userInput % 10;
minutes = (int) userInput / 60;
userInput = userInput % 10;
seconds = (int) userInput / 60;
userInput = userInput % 10;
}while (userInput >=1);
System.out.print("The number " +totalTime+ " is " +years+ " years, " +months+ " months, " +weeks+ " weeks, " +days+ " days, " +hours+ " hours, " +minutes+ " minutes, " +seconds+ " seconds.");
}
我認為拔出每種面額后不能使用模10減少輸入。 另外,您根本不需要while循環。
你必須做類似的事情
years = (int) (userInput / 365);
userInput = userInput - years*365;
等等。 另外,由於輸入的天數是天數,因此除數時,您必須繼續考慮數天,因此,除以12來獲得月數是沒有意義的。 您可以將其除以30、31或28。類似地,對於小時,則必須將剩余的天數乘以24,然后取小時的小數部分,然后類似地分解為分鍾和秒。
我想輸入的是一天。
您的代碼中很少有奇怪的事情:
while
循環不是必需的 (int) userInput
將在除法之前將userInput強制轉換為int
,在這里不是很重要,但是要小心;) userInput % 10
無處不在 這是解決方案的框架:
float userInput /* = ... */ ;
int years = (int)(userInput/365) ;
userInput = userInput - years*365 ; // or userInput%365 ;
int month = (int)(userInput/30);
userInput = userInput - month*30 ; // or userInput%30 ;
int day = (int) userInput ;
userInput = userInput - day ;
userInput = userInput * 24 ; //transform in hours
int hours = (int)hours ;
userInput = userInput - hours ;
userInput = userInput * 60 ; // transform in minute
int minutes = (int)userInput ;
userInput = userInput - minutes ;
userInput = userInput * 60 ; // transform in second
int seconds = (int) userInput ;
好的,這里有幾件事:
%=
。 這是工作代碼:
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class TimePartition {
public static void main(String[] args) throws Exception {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
while (true) {
System.out.println("Enter a positive number to decompose");
String input = br.readLine();
if (input.equals("")) break;
float inputAsFloat = Float.parseFloat(input);
if (inputAsFloat == 0.0) break;
// the input is an integral day count, with a possible fractional part representing time as a fraction of one day
int totalDays = (int)inputAsFloat;
int totalSeconds = (int)((inputAsFloat-totalDays)*60.0*60.0*24.0);
// decompose totalDays into date fields
int years = 0;
int months = 0;
int weeks = 0;
int days = 0;
// ignores leap years
years = (int)totalDays/365;
totalDays %= 365;
// assumes all months have 30 days
months = (int)totalDays/30;
totalDays %= 30;
weeks = (int)totalDays/7;
totalDays %= 7;
days = (int)totalDays;
// decompose totalSeconds into time fields
int hours = 0;
int minutes = 0;
int seconds = 0;
hours = (int)totalSeconds/3600;
totalSeconds %= 3600;
// ignores leap seconds
minutes = (int)totalSeconds/60;
totalSeconds %= 60;
seconds = (int)totalSeconds;
System.out.println("The number "+inputAsFloat+" is "+years+" years, "+months+" months, "+weeks+" weeks, "+days+" days, "+hours+" hours, "+minutes+" minutes, "+seconds+" seconds.");
} // end while
} // end main()
} // end class TimePartition
演示:
bash> ls
TimePartition.java
bash> javac TimePartition.java
bash> ls
TimePartition.class* TimePartition.java
bash> CLASSPATH=. java TimePartition
Enter a positive number to decompose
768.96
The number 768.96 is 2 years, 1 months, 1 weeks, 1 days, 23 hours, 2 minutes, 25 seconds.
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.