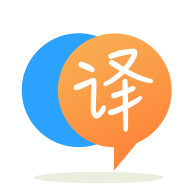
[英]Getting the Current Location Lat Long as 0.0 in Android google maps
[英]Android | Google Maps Getting Current Location and Update
我想在地圖上獲取當前位置並在移動時更新它。 每當更新發生時,我想獲得當前的longtidude和latitute值以用於其他方法。
private LocationRequest request = LocationRequest.create().setInterval(50000);
我想我必須使用LocationRequest
。 我創建了一個每5分鍾更新一次位置的對象。 但現在我不知道如何使用它。 我在互聯網上查了教程,但對於初學者來說它們是如此復雜。 有人有簡單的解決方案嗎?
編輯
這就是我的代碼現在的樣子:
public class MainActivity extends Activity implements LocationListener {
private GoogleMap map;
public double latitude;
public double longitude;
private LocationManager locationManager;
private Context mContext;
private android.location.LocationListener locationListener;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
;
map = ((MapFragment) getFragmentManager().findFragmentById(R.id.map)).getMap();
map.setMyLocationEnabled(true);
getLocation();
Toast.makeText(getApplicationContext(), "Your Location is - \nLat: " + latitude + "\nLong: " + longitude, Toast.LENGTH_LONG).show();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
@Override
public void onLocationChanged(Location location) {
if (location != null) {
longitude = location.getLongitude();
latitude = location.getLatitude();
locationManager.requestLocationUpdates(LocationManager.NETWORK_PROVIDER, 0, 0, locationListener);
}
}
public void getLocation()
{
locationManager = (LocationManager) mContext.getSystemService(LOCATION_SERVICE);
}
}
當我嘗試測試時,我的應用程序停止了工作。 由於這是我的第一個Android應用程序,我不是很擅長,我找不到什么錯。
對於位置使用這個
locationManager = (LocationManager) getSystemService(LOCATION_SERVICE);
// Define a listener that responds to location updates
locationListener = new LocationListener() {
public void onLocationChanged(Location location) {
// Called when a new location is found by the network location provider.
longitude = String.valueOf(location.getLongitude());
latitude = String.valueOf(location.getLatitude());
Log.d(TAG, "changed Loc : " + longitude + ":" + latitude);
}
public void onStatusChanged(String provider, int status, Bundle extras) {
}
public void onProviderEnabled(String provider) {
}
public void onProviderDisabled(String provider) {
}
};
// getting GPS status
isGPSEnabled = locationManager.isProviderEnabled(LocationManager.GPS_PROVIDER);
// check if GPS enabled
if (isGPSEnabled) {
Location location = locationManager.getLastKnownLocation(LocationManager.GPS_PROVIDER);
if (location != null) {
longitude = String.valueOf(location.getLongitude());
latitude = String.valueOf(location.getLatitude());
locationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER, 0, 0, locationListener);
} else {
location = locationManager.getLastKnownLocation(LocationManager.NETWORK_PROVIDER);
if (location != null) {
longitude = String.valueOf(location.getLongitude());
latitude = String.valueOf(location.getLatitude());
locationManager.requestLocationUpdates(LocationManager.NETWORK_PROVIDER, 0, 0, locationListener);
} else {
longitude = "0.00";
latitude = "0.00";
}
}
}
來自http://androidadvance.com/android_snippets.php#h.r43fot3suy6h
使用gps確定位置(longtidude和latitute值)並將其傳遞給地圖
this mainactivity.java for gps
public class GpsBasicsAndroidExample extends Activity implements LocationListener {
private LocationManager locationManager;
TextView text;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_gps_basics_android_example);
text=(TextView)findViewById(R.id.tv1);
/********** get Gps location service LocationManager object ***********/
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
/*
Parameters :
First(provider) : the name of the provider with which to register
Second(minTime) : the minimum time interval for notifications, in milliseconds. This field is only used as a hint to conserve power, and actual time between location updates may be greater or lesser than this value.
Third(minDistance) : the minimum distance interval for notifications, in meters
Fourth(listener) : a {#link LocationListener} whose onLocationChanged(Location) method will be called for each location update
*/
locationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER,1,10, this);
/********* After registration onLocationChanged method called periodically after each 3 sec ***********/
}
/************* Called after each 3 sec **********/
@Override
public void onLocationChanged(Location location) {
String str = "Latitude: "+location.getLatitude()+" \nLongitude: "+location.getLongitude();
//Toast.makeText(getBaseContext(), str, Toast.LENGTH_LONG).show();
text.setText(str);
}
@Override
public void onProviderDisabled(String provider) {
/******** Called when User off Gps *********/
Toast.makeText(getBaseContext(), "Gps turned off ", Toast.LENGTH_LONG).show();
}
@Override
public void onProviderEnabled(String provider) {
/******** Called when User on Gps *********/
Toast.makeText(getBaseContext(), "Gps turned on ", Toast.LENGTH_LONG).show();
}
@Override
public void onStatusChanged(String provider, int status, Bundle extras) {
// TODO Auto-generated method stub
}
}
這對於地圖
main.java
enter code here
公共類MainActivity擴展FragmentActivity {
GoogleMap mMap;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mMap = ((MapFragment) getFragmentManager().findFragmentById(R.id.map)).getMap();
mMap.setMapType(GoogleMap.MAP_TYPE_SATELLITE);
final LatLng CIU = new LatLng(35.21843892856462, 33.41662287712097);
Marker ciu = mMap.addMarker(new MarkerOptions()
.position(CIU).title("My Office"));
final LatLng CIU1 = new LatLng(30.21843892856462, 33.41662287712097);
Marker ciu1 = mMap.addMarker(new MarkerOptions()
.position(CIU1).title("My Second Office"));
final LatLng CIU2 = new LatLng(30.21843892856462, 30.41662287712097);
Marker ciu2 = mMap.addMarker(new MarkerOptions()
.position(CIU2).title("My thired Office"));
}
}
這是你可以做的。 我會盡量讓你變得簡單:
使用implements關鍵字將LocationListener接口添加到擴展活動類。 這將強制您覆蓋查找當前位置所需的一些方法。
public class A extends Activity implements LocationListener {}
創建一個Location Manager類的實例,它將作為一個鈎子來調用Location包中的各種服務和方法。
private LocationManager locationManager;
創建一個類似getLocation()的方法,並從Location Manger實例調用預定義的服務方法。
locationManager = (LocationManager) mContext .getSystemService(LOCATION_SERVICE);
在onLocationChanged()方法中,您可以使用getLongitude()使用getLongitude()使用這兩個方法(位置管理器類的一部分)請求緯度。
將這些方法獲得的兩個值存儲在兩個單獨的變量中,確保它們是Double類型,稍后您可以將它們轉換為String類型,然后在應用程序活動的Text視圖中顯示它們。
if (location != null) { longitude = String.valueOf(location.getLongitude()); latitude = String.valueOf(location.getLatitude()); locationManager.requestLocationUpdates(LocationManager.NETWORK_PROVIDER, 0, 0, locationListener); }
調用onCreate()中的getLocation()方法,並通過TextView或Toast在應用程序屏幕上顯示它們。
Toast.makeText(getApplicationContext(), "Your Location is - \\nLat: " + latitude + "\\nLong: " + longitude, Toast.LENGTH_LONG).show();
最后但並非最不要忘記在Manifest文件中添加權限。
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> <uses-permission android:name="android.permission.INTERNET" />
更多詳情請參閱此鏈接 。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.