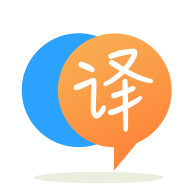
[英]How can I modify my program to make the histogram vertical? I'm having trouble making it vertical. Code is below
[英]I'm having trouble with my conversion program
對於我的課程,我將用C ++編寫一個程序,將句子中的每個字符轉換為相反的情況(從上到下,從下到上)。 我們應該使用數組和用戶定義的方法,這就是我想出的:
#include <iostream>
using namespace std;
// declare variables
int count = 0; // array counter
int i = 0; // loop control
char ch[100]; // each character entered will be stored in this array
char newCh[100]; // this will hold each character after its case has been changed
main()
{
cout << "Enter a sentence." << endl; // prompts user
while ( ch[count] != '\n' ) // loop continues until "enter" is pressed
{
cin >> ch[count]; // store each character in an array
count += 1; // increment counter
}
int convert(); // call user-defined function
}
// even though it isn't necessary, we are using a user-defined function to perform the conversion
int convert()
{
for ( i = 0; i >= 0; i++ )
{
if ( (ch[i] > 64) and (ch[i] < 91)
)
{
newCh[i] = tolower(ch[i]);
}
else
{
newCh[i] = toupper(ch[i]);
}
cout << newCh[i];
}
}
我不知道為什么,但它不起作用。 我不相信我的while循環正在終止並執行程序的其余部分。 任何建議將不勝感激。
while ( ch[count] != '\\n' )
的循環條件是錯誤的,因為ch
所有條目都將被編譯器初始化為零,並且當你在循環內增加count
,條件永遠不會為false
而你有一個無限循環,導致你寫超出數組的限制。
超出數組限制的寫入會導致未定義的行為 ,並導致整個程序非法。
我建議你學習std::string
和std::getline
。
你的for循環有問題 - 你想要(i = 0; i <count; i ++)。 你的函數也可以是void,你需要將count值傳遞給它(你只需要使用convert()調用它,而不需要在前面使用int或void。
我通過一些修改重寫了你的代碼。 以下代碼在我的機器中完美運行 -
#include <iostream>
#include<cstdio>
#include<string>
using namespace std;
void convert(char *, int);
string line;
char input[1024];
char output[1024];
main()
{
cout << "Enter a sentence." << endl;
while (getline(cin, line)) { // POINT 1
cout<< line<<endl;
//converting to char array since you need char array
//POINT 2
for(int i=0; i< line.length(); i++){
input[i]=line[i];
}
convert(input, line.length());
cout<<output<<endl;
input[1024] = {0}; //POINT 3
output[1024] = {0};
}
}
//Custom Convert Method
void convert(char input[], int size){
for(int i = 0; i < size; i++){
if(input[i] >= 'a' && input[i] <= 'z'){
output[i] = toupper(input[i]);
} else {
output[i] = tolower(input[i]);
}
}
}
注意這里的一些觀點(在我的評論中) -
要點1:使用getline()
方法讀取整行。 這里的line
是一個string
要點2:既然你需要char數組,我將字符串line
轉換為char數組輸入[1024]
要點3:正在重置input
和output
數組以使用下一個值;
輸出代碼:
“Ctrl + C”將終止程序
希望它會對你有所幫助。
非常感謝。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.