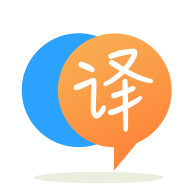
[英]How do I access a template class member field in a specialised template class in C++?
[英]In C++, how do I template a class to use another class and a member of that class?
因此,我希望能夠在不同情況下使用我們為家庭作業設計的數據結構。 具體來說,我有一個minHeap,但我想對其進行模板處理以獲取對象。 由於minHeap必須比較元素以維護結構並有效地返回最小值,因此可以模板化我的minHeap類以將任何給定對象和該對象的成員作為要在元素之間進行比較的事物嗎?
我的想法是這樣的:
template<class TYPE, class TYPE_COMPARE>
class minHeap {
...(stuff inside)...
};
但是我意識到我無法真正使用TYPE_COMPARE訪問類型為TYPE的對象。 這就是為什么我想知道是否可以模板化一個類來接受另一個類和該類的成員。
編輯:有人建議添加我的代碼以進行澄清。 這就是我想像的樣子,但是我強烈感覺它無法像這樣工作。
這是我的minHeap的頭文件。
#include <vector>
using namespace std;
template<class TYPE, TYPE::class MEMBER>
class minHeap {
private:
vector<TYPE> nodes;
int current;
int parent(int i) {
return (i-1)/2;
}
int leftChild(int i) {
return 2*i+1;
}
int rightChild(int i) {
return 2*i+2;
}
void bubbleUp(int i) {
if(i > 0) {
int p = parent(i);
if(nodes[i]::MEMBER < nodes[p]::MEMBER) {
swap(nodes[i], nodes[p]);
bubbleUp(p);
}
}
}
void bubbleDown(int i) {
if(i < current) {
int a = leftChild(i);
int b = rightChild(i);
if(b > current && a <= current) {
if(nodes[i]::MEMBER > nodes[a]::MEMBER) {
swap(nodes[i], nodes[a]);
}
}
else if(b <= current && a <= current) {
if(nodes[i]::MEMBER > nodes[a]::MEMBER || nodes[i]::MEMBER > nodes[b]::MEMBER) {
if(nodes[a] < nodes[b]) {
swap(nodes[i], nodes[a]);
bubbleDown(a);
}
else {
swap(nodes[i], nodes[b]);
bubbleDown(b);
}
}
}
}
}
public:
minHeap() {
current = -1;
}
void insert(TYPE x) {
current++;
nodes.push_back(x);
bubbleUp(current);
}
TYPE extractMin() {
TYPE min = nodes[0];
swap(nodes[0], nodes[current]);
current--;
nodes.pop_back();
bubbleDown(0);
return min;
}
bool empty() {
if(current < 0)
return true;
return false;
}
};
您當然可以訪問TYPE
。 但是由於只有類型,因此只能訪問靜態成員。
class MyType
{
static void SomeStaticFunction();
};
template<class TYPE>
class minHeap
{
void fn()
{
TYPE::SomeStaticFunction();
}
};
如果要訪問非靜態成員,則自然需要一個實例來操作:
class MyType
{
void SomeNonStaticFunction();
};
template<class TYPE>
class minHeap
{
void fn(const TYPE& inst)
{
inst.SomeNonStaticFunction();
}
};
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.