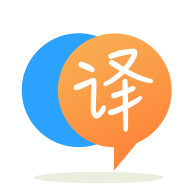
[英]Java: Match tokens between two strings and return the number of matched tokens
[英]Count number of strings that match in two arrays in Java
給定兩個按字母順序排列的字符串數組a和b,我想返回兩個數組中匹配的字符串數的計數。
public int countMatch(String[] a, String[] b) {
}
我的目標是能夠按以下方式調用該方法;
countMatch({"apple", "banana", "xylophone"}, {"banana", "carrot", "dog", "xray", "xylophone"});
它應該返回int值2,因為每個數組都有兩個匹配的字符串(“香蕉”和“木琴”)。
重要的是要注意,如果這有助於解決方案,則數組都將始終按字母順序排列。
對這些數組進行排序后,您可以執行以下操作:
您將有兩個變量: i
和j
。 第一個將遍歷第一個數組,第二個至第二個。
然后比較a[i]
和b[j]
。 如果它們相等,則匹配,並且您將兩個索引都提前。 如果它們不等於提前索引,則該索引在較小的單詞處(如果a[i] < b[j]
提前i
else j
)。
您可以創建一個簡單的方法,例如:
public int countMatch(String[] a, String[] b) {
List<String> list1 = new ArrayList(Arrays.asList(a));
List<String> list2 = Arrays.asList(b);
list1.retainAll(list2);
return list1.size();
}
我的代碼如下:
public class Test {
public static int findMatchCount(final String [] a,final String [] b){
int matchCount = 0;
for(int i = 0, j = 0;i < a.length && j < b.length;){
int res = a[i].compareTo(b[j]);
if(res == 0){
matchCount++;
i++;
j++;
}else if(res < 0){
i++;
}else{
j++;
}
}
return matchCount;
}
public static void main(String[] args){
String[] a = {"apple", "apple"};
String[] b = {"apple", "apple"};
System.out.println(findMatchCount(a,b));
}
}
您可以使用for
循環比較這些數組,並將第一個數組的第一個索引與所有第二個數組索引進行比較,然后繼續到其他第一個數組索引。 像這樣:
public class Ber {
public static void main( String[] arg){
String[] abc1 = {"1", "7", "j", "kolo", "7", "1"};
String[] abc2 = {"2", "n", "m", "2", "n", "kolo"};
for (int i = 0, j = 0; j < abc2.length; i++) {
if (abc1[i].equals(abc2[j])){
System.out.println("found first index: "+i+" second index: "+j);
break;
} else if (i == abc1.length-1) {
i = 0;
j++;
}
System.out.println("searching... "+i+" "+j);
}
}
}
您會看到類似以下內容:
searching... 0 0
searching... 1 0
searching... 2 0
searching... 3 0
searching... 4 0
searching... 0 1
searching... 1 1
searching... 2 1
searching... 3 1
searching... 4 1
searching... 0 2
searching... 1 2
searching... 2 2
searching... 3 2
searching... 4 2
searching... 0 3
searching... 1 3
searching... 2 3
searching... 3 3
searching... 4 3
searching... 0 4
searching... 1 4
searching... 2 4
searching... 3 4
searching... 4 4
searching... 0 5
searching... 1 5
searching... 2 5
found first index: 3 second index: 5
嘗試這個
public final class StringArrayMatcher{
public int findMatchCount(final String [] a,final String [] b){
/*
If a has more than one entries that matches to a string in b, it's also considered as accountable.
*/
int matchCount = 0;
for(String x_element : a){
for(String y_element : b){
if(x_element.equals(y_element)) ++matchCount;
}
}
return matchCount;
}
public static void main(String[] args) {
System.out.format("Total matches = %d",new StringArrayMatcher().findMatchCount(new String[]{"apple", "banana", "xylophone"}, new String[]{"banana", "carrot", "dog", "xray", "xylophone"}));
}
}
除非最終性能成為問題,否則請忽略它們已排序的事實。 將a []中的所有值放入HashSet中。 循環遍歷b []並計算集合中包含的數量。
如您所說,如果您想只對兩個匹配的單詞進行精確計數一次,而不管它們是否出現; 試試這個代碼
import java.util.Set;
import java.util.HashSet;
import java.util.Arrays;
import java.util.List;
public final class StringArrayMatcher{
public int findMatchCount(final String [] a,final String [] b){
int matchCount = 0;
Set<String> setA = new HashSet<>(Arrays.asList(a));
List<String> setB = Arrays.asList(b);
for(String value1 : setA){
for(String value2 : setB){
if(value1.equals(value2)){ ++matchCount; break; }
}
}
return matchCount;
}
public static void main(String[] args) {
System.out.format("Total matches = %d",new StringArrayMatcher().findMatchCount(new String[]{"apple"}, new String[]{"apple","apple"}));
}
}
*使用Set會自動修剪數組中重復的單詞。
如果您完全不關心字母順序。 這很簡單,您需要兩個循環來完成工作,即,您需要比較所有可能的對。 在您的示例中,您需要進行3 * 5比較才能得出結論。
public int countMatch(String[] a, String[] b) {
int count = 0;
for(int i=0; i<a.length; i++){
for(int j=0; j<b.length; i++){
if(a[i].equals(b[i])){
count++;
break;
}
}
}
return count;
}
但是,如果考慮字母順序,則可以簡單地對其進行改進。
public int countMatch1(String[] a, String[] b) {
int count = 0;
int cursor = 0;
for(int i=0; i<a.length; i++){
for(int j=cursor; j<b.length; i++){
if(a[i].equals(b[i])){
count++;
cursor = j;
break;
}
}
}
return count;
}
在這種情況下,您可以使用可變光標記住上一次搜索數組a [i]的停止位置,然后對於下一次搜索a [i + 1]可以簡單地從光標開始而不是從頭開始,因為所有光標之前的前一個肯定不能匹配a [i + 1]。
使用集和列表執行此操作的一種好方法。 如果您遲早使用Java,那么無論如何都要開始使用它們,不妨早日開始研究它們的工作方式。
import java.util.Set;
import java.util.HashSet;
import java.util.Arrays;
public final class Match{
public int countMatch( String [] a, String [] b){
Set<String> set = new HashSet<>(Arrays.asList(a));
set.retainAll(Arrays.asList(b));
System.out.println("The number of matches is " + set.size());
return set.hashCode();
}
public static void main(String[] args) {
String[] a = {"apple", "banana", "xylophone"};
String[] b = {"banana", "carrot", "dog", "xray", "xylophone"};
Match countMatch = new Match();
countMatch.countMatch(a, b);
}
}
此功能為您完成工作,在這種情況下,它的工作是對兩個匹配的單詞進行精確計數一次 ,而不管它們是否出現。
讓我們看看它是如何工作的:
它遍歷2個數組,並且僅比較每個數組中最后一次出現的迭代項,以不計算重復項。 最后,為了進行一些優化,如果第二個數組的項比較小,我們將跳過對第一個數組項的檢查。
僅當2個數組按字母順序排序時,此功能才起作用 。
public int commonTwo(String[] a, String[] b) {
int c = 0;
for(int i = 0; i < a.length; i++){
for(int j = 0; j < b.length; j++){
if(a.length - i >= 2 && a[i].equals(a[i+1])) break;
if(b.length - j >= 2 && b[j].equals(b[j+1])) continue;
int cmp = a[i].compareTo(b[j]);
if(cmp == 0) {
c++;
} else if(cmp < 0){
break;
}
}
}
return c;
}
樣品:
public class Main {
public static void main(String[] args) {
String[] a = {"a", "c", "x"};
String[] b = {"a", "b", "c", "x", "z"};
int count = commonTwo(a, b);
System.out.println(count); // prints 3
String[] a2 = {"a", "a", "b", "b", "c"};
String[] b2 = {"a", "b", "b", "b", "c"};
int count = commonTwo(a2, b2);
System.out.println(count); // prints 3
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.