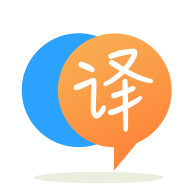
[英]I am trying to write a quickSort method as part of an assignment, but I keep getting a StackOverflow error in Java
[英]I am trying to implement QuickSort in java but Could not get output?
我正在嘗試通過將last Element作為樞軸來實現quickSort。 但是我無法產生有效的輸出。
為了使其隨機化,還需要進行哪些更改? 我正在用Java編寫代碼。
public class QuickSortDemo {
public static void quicksort(int A[], int temp[], int left, int right) {
int q, i;
//why u need to check this base case always
if (left < right) {
q = partition(A, temp, left, right);
quicksort(A, temp, left, q - 1);
quicksort(A, temp, q + 1, right);
for (i = 0; i < A.length; i++) {
System.out.println(A[i]);
}
}
}
public static int partition(int A[], int temp[], int left, int right) {
int x, i, j;
x = A[right];
i = left - 1;
for (j = left; j <= right - 1; j++) {
if (A[j] <= x) {
i = i + 1;
A[i] = A[j];
}
}
A[i + 1] = A[right];
return i + 1;
}
public static void main(String s[]) {
int ar[] = {6, 3, 2, 5, 4};
int p[] = new int[ar.length];
quicksort(ar, p, 0, ar.length - 1);
}
} //end class QuickSortDemo
輸出顯示
2
2
4
5
4
2
2
4
4
4
2
2
4
4
4
如注釋中所述,分區方法中存在一些錯誤。 在實現快速排序時,您希望交換數組中的元素,而不僅僅是覆蓋前面的元素。 他們會迷路的。
此外,代碼從左側開始,並且始終交換每個小於或等於樞軸的元素。 如果元素已經在數組的正確部分中,則這是不必要的操作。
最好搜索一個大於左側樞軸的元素。 然后,搜索一個小於右側樞軸的元素,以僅交換它們,然后繼續直到所有必需的元素都被交換為止。
標准實現(我知道)看起來像這樣
public static int partition(int A[], int left, int right) {
int pivot, i, j;
pivot = A[right];
i = left;
j = right - 1;
do {
// Search for element greater than pivot from left, remember position
while ( A[i] <= pivot && i < right ) i++;
// Search for element less than pivot from right, remember positon
while ( A[j] >= pivot && j > left ) j--;
// If elements are in the wrong part of the array => swap
if ( i < j ) swap( A, i, j );
// Continue until the whole (sub)array has been checked
} while ( j > i );
// Put the pivot element at its final position if possible
if ( A[i] > pivot )
swap ( A, i, right );
// Return the border / position of the pivot element
return i;
}
交換與往常一樣
public static void swap(int[] A, int first, int second) {
int temp = A[first];
A[first] = A[second];
A[second] = temp;
}
另請注意,在此代碼內無需使用temp
。 您的代碼在其簽名中聲明了它,但從未使用過它。
這是我想到的代碼。 在這里,我將最右邊的值作為關鍵值和結轉額。 通過遞歸進行進一步。 希望您能諒解。
public class QuickSortDemo {
public static void main(String[] args) {
int[] input = {6, 3, 2, 5, 4};
int[] output = quickSort(input, 0, input.length-1);
for(int a : output) {
System.out.println(a);
}}
public static int[] quickSort(int[] array, int lowerIndex, int higherIndex) {
int i = lowerIndex;
int j = higherIndex;
// calculate pivot number, rightmost value
int pivot = array[higherIndex];
// Divide into two arrays
while (i <= j) {
while (array[i] < pivot) {
i++;
}
while (array[j] > pivot) {
j--;
}
if (i <= j) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
//move index to next position on both sides
i++;
j--;
}
}
// call quickSort() method recursively
if (lowerIndex < j)
quickSort(array,lowerIndex, j);
if (i < higherIndex)
quickSort(array, i, higherIndex);
return array;
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.