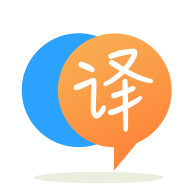
[英]How to store JSON data from two sources in javascript objects when the data is pulled in at different rates?
[英]How to pull JSON data from two different sources?
我想知道是否有一種方法可以從兩個不同的來源提取和使用JSON數據。 當前,代碼如下所示:
//JSON1
$.getJSON('url1',function(data){
$.each(data,function(key,val){
//code
});
});
//JSON2
$.getJSON('url2',function(data){
$.each(data,function(key,val){
//code
});
});
當我這樣做時,我似乎發現從一個JSON函數創建的變量在另一函數中不可用,這使得它們很難一起使用。 是否有更好的方法將這兩個工具一起使用?
此函數將網址數組和回調作為參數:
function getMultiJSON(urlList,callback) {
var respList = {};
var doneCount = 0;
for(var x = 0; x < urlList.length; x++) {
(function(url){
$.getJSON(url,function(data){
respList[url] = data;
doneCount++;
if(doneCount === urlList.length) {
callback(respList);
}
});
})(urlList[x]);
}
}
您可以這樣使用它:
getMultiJSON(['url1','url2'],function(response) {
// in this case response would have 2 properties,
//
// response.url1 data for url1
// response.url2 data for url2
// continue logic here
});
您可能要添加超時,因為如果任何URL加載失敗,該函數將永遠不會調用處理程序
輕松使用開源項目jinqJs( http://www.jinqJs.com )
var data1 = jinqJs().from('http://....').select();
var data2 = jinqJs().from('http://....').select();
var result = jinqJs().from(data1, data2).select();
該示例進行同步調用,您可以通過執行以下操作來進行異步調用:
var data1 = null;
jinqJs().from('http://....', function(self){ data1 = self.select(); });
結果將同時包含兩個結果。
如果控制端點,則可以使它一次返回所有想要的數據。 然后您的數據將如下所示:
{
"url1_data": url1_json_data,
"url2_data": url2_json_data
}
如果仍然有2個端點需要命中,則可以將第一個ajax調用的結果傳遞給第二個函數(但這會使您的2個ajax調用同步):
function getJson1(){
$.getJSON('url1',function(data){
getJson2(data);
});
}
function getJson2(json1Data){
$.getJSON('url2',function(data){
//Do stuff with json1 and json2 data
});
}
getJson1();
在函數內使用var
(或塊,使用let
)聲明的變量在函數(或塊)之外不可用。
$.getJSON('url1',function(data){
$.each(data,function(key,val){
var only_accessible_here = key;
});
});
因此,如果希望在聲明它們的函數范圍之外訪問變量,則需要在使用它們的函數之外聲明它們。
var combined_stuff = ''
$.getJSON('url1',function(data){
$.each(data,function(key,val){
combined_stuff += val;
});
});
//JSON2
$.getJSON('url2',function(data){
$.each(data,function(key,val){
combined_stuff += val;
});
});
正如Marc B所說的那樣,如果getJSON
調用之一失敗,或者如果兩個都失敗,則無法知道首先更新JSON1
或首先更新JSON2
或僅更新一個順序,無法知道combined_stuff
變量的更新順序。
如果更新順序很重要,請在要先調用的功能中調用要使用的第二個。
var combined_stuff = ''
$.getJSON('url1',function(data){
$.each(data,function(key,val){
combined_stuff += val;
//JSON2
$.getJSON('url2',function(data){
$.each(data,function(key,val){
combined_stuff += val;
});
});
});
});
我建議您使用$ .when函數在jquery中可用,以並行執行兩個方法,然后執行操作。 請參閱下面的代碼片段,
var json1 = [], json2 = []; $.when(GetJson1(), GetJson2()).always(function () { //this code will execute only after getjson1 and getjson2 methods are run executed if (json1.length > 0) { $.each(json1,function(key,val){ //code }); } if (json2.length > 0) { $.each(json2,function(key,val){ //code }); } }); function GetJson1() { return $.ajax({ url: 'url1', type: 'GET', dataType: 'json', success: function (data, textStatus, xhr) { if (data != null) { json1 = data; } }, error: function (xhr, textStatus, errorThrown) { json1 = [];//just initialize to avoid js error } } function GetJson2() { return $.ajax({ url: 'url2', type: 'GET', dataType: 'json', success: function (data, textStatus, xhr) { if (data != null) { json2 = data; } }, error: function (xhr, textStatus, errorThrown) { json2 = [];//just initialize to avoid js error } }
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
每個AJAX調用返回的數據都無法在其自己的回調函數之外使用。 我敢肯定還有更優雅的(復雜的)解決方案,但是有兩個簡單的Occamic解決方案包括全局變量,或將接收到的數據存儲在隱藏的輸入元素中。
在每個回調函數中,只需循環直到出現另一個調用的數據:
function getJson1(){
$.getJSON('url1',function(data){
var d2 = '';
$('#hidden1').val(data);
while ( d2 == '' ){
//you should use a time delay here
d2 = $('#hidden2').val();
}
getJson2();
});
}
function getJson2(){
$.getJSON('url2',function(d2){
var d1 = '';
$('#hidden2').val(d2);
while ( d1 == '' ){
//you should use a time delay here
d1 = $('#hidden1').val();
}
//Do stuff with json1 and json2 data
});
}
getJson1();
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.