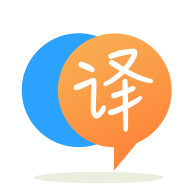
[英]How do I store positive and negative value (may be separately?) into an array, and print them out?
[英]How do I store multiple values (with different types) in an array and print them out?
我的程序要求用戶創建一個銀行帳戶。 目前,它只能創建一個帳戶並打印出來。 我想擴展我的程序,以便它可以一次又一次選擇菜單選項來創建多個帳戶,並最終打印所有帳戶詳細信息。
package bankapp_assignment;
import java.util.Scanner;
public class BankApp_Assignment {
public static void main(String[] args) {
BankAccount[] accounts = new BankAccount[10];
Scanner sc = new Scanner(System.in);
int userChoose;
String name = null;
int accNum = 0;
double initiateAmount = 0;
double newAmm = 0;
double depOrWith = 0;
System.out.println("WELCOME TO OUR BANK!\n\n"
+ "...................\n"
+ "...................\n\n"
+ "Choose your optiin:\n"
+ "1. Create new account\n"
+ "2. View all the accounts property\n"
+ "3. Quit\n\n");
System.out.println("*************\n"
+ "************");
while (true) {
userChoose = sc.nextInt();
sc.nextLine();
if (userChoose == 1) {
/*the user must be able to create multiple accounts, let's say 10 accounts for instance
To open another new account the user should choose the menu option "1" again and continue...
*/
System.out.println("Enter your full name:");
name = sc.nextLine();
System.out.println("Choose an account number:");
accNum = sc.nextInt();
System.out.println("Enter an initiating amount:");
initiateAmount = sc.nextDouble();
System.out.println("\n-----------\n"
+ "------------");
} else if (userChoose == 2) {//view all the accounts property (including account number and initial balance)
} else if (userChoose == 3) {
System.exit(0);
}
}
}
}
銀行賬戶:
package bankapp_assignment;
public class BankAccount {
public void createAcc(){
}
}
納曼回答后編輯:
public static void main(String[] args) {
BankAccount[] accounts = new BankAccount[10];
Scanner sc = new Scanner(System.in);
int userChoose;
String name = null;
int accNum = 0;
double initiateAmount = 0;
double newAmm = 0;
double depOrWith = 0;
System.out.println("WELCOME TO OUR BANK!\n\n"
+ "...................\n"
+ "...................\n\n"
+ "Choose your optiin:\n"
+ "1. Create new account\n"
+ "2. View all the accounts property\n"
+ "3. Quit\n\n");
System.out.println("*************\n"
+ "************");
while (true) {
userChoose = sc.nextInt();
sc.nextLine();
if (userChoose == 1) {
/*the user must be able to create multiple accounts, let's say 10 accounts for instance
To open another new account the user should choose the menu option "1" again and continue...
*/
System.out.println("Enter your full name:");
name = sc.nextLine();
System.out.println("Choose an account number:");
accNum = sc.nextInt();
System.out.println("Enter an initiating amount:");
initiateAmount = sc.nextDouble();
System.out.println("\n-----------\n"
+ "------------");
accounts[numOfAcc]=bankAcc;
numOfAcc++;
} else if (userChoose == 2) {//view all the accounts property (including account number and initial balance)
for(BankAccount bankAccount: accounts){
System.out.println("Your name: " + name);
System.out.println("Your account number: " + accNum);
System.out.println("Your current balance: " + initiateAmount);
System.out.println("\n-----------\n"
+ "------------");
}
} else if (userChoose == 3) {
System.exit(0);
}
}
}
}
如果要繼續使用數組,則可以像先初始化靜態變量那樣進行操作:
static int number = 0;
如下更改您的塊:
if (userChoose == 1) {
BankAccount ac = new BankAccount();
//set account properties;
accounts[number] = ac;
number++;
}
您可以像下面這樣檢索數組:
for(BankAccount bankAccount: accounts){
//bankAccount.getProperty();
}
您的代碼應該像
BankAccount bankAcc = null;
if (userChoose == 1) {
bankAcc = new BankAccount();
/*the user must be able to create multiple accounts, let's say 10 accounts for instance
To open another new account the user should choose the menu option "1" again and continue...
*/
System.out.println("Enter your full name:");
name = sc.nextLine();
bankAcc.setName(name);//setter method of your bankAccount bean
System.out.println("Choose an account number:");
accNum = sc.nextInt();
bankAcc.setAccountNum(accNum);
System.out.println("Enter an initiating amount:");
initiateAmount = sc.nextDouble();
bankAcc.setInitialAmount(initialAmount);
System.out.println("\n-----------\n"
+ "------------");
accounts[numOfAcc]=bankAcc;
numOfAcc++;
}
檢索如下:
else if (userChoose == 2) {//view all the accounts property (including account number and initial balance)
for(BankAccount bankAccount: accounts){
System.out.println("Your name: " + bankAccount.getName());
System.out.println("Your account number: " + bankAccount.getAccountNum());
System.out.println("Your current balance: " + bankAccount.getInitialAmount());
System.out.println("\n-----------\n"
+ "------------");
}
1)創建一個對象類:例如
class BankAccount{
private String userName;
private String userSurname;
private String birthDay;
private int accountNumber;
private int ibanNo;
private int balance;
public BankAccount(String userName, String userSurname, String birthDay, int accountNumber, int ibanNo, int balance) {
super();
this.userName = userName;
this.userSurname = userSurname;
this.birthDay = birthDay;
this.accountNumber = accountNumber;
this.ibanNo = ibanNo;
this.balance = balance;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getUserSurname() {
return userSurname;
}
public void setUserSurname(String userSurname) {
this.userSurname = userSurname;
}
public String getBirthDay() {
return birthDay;
}
public void setBirthDay(String birthDay) {
this.birthDay = birthDay;
}
public int getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(int accountNumber) {
this.accountNumber = accountNumber;
}
public int getIbanNo() {
return ibanNo;
}
public void setIbanNo(int ibanNo) {
this.ibanNo = ibanNo;
}
public int getBalance() {
return balance;
}
public void setBalance(int balance) {
this.balance = balance;
}
}
2)在您的主類中使用此對象創建一個全局列表;
List<BankAccount> accounts = new ArrayList<BankAccount>();
3)現在您可以將新帳戶添加到列表中;
accounts.add(new BankAccount(String userName, String userSurname, String birthDay, int accountNumber, int ibanNo, int balance));
4)現在您可以使用帳戶列表添加/獲取/刪除帳戶。
創建銀行帳戶詳細信息的對象,然后簡單地添加到ArrayList中。使用java.util包中的ArrayList數據類型創建一個包含銀行帳戶詳細信息的類,只需將其導入到此類中並編寫以下代碼
List <BankAccount> accounts = new <BankAccount> ArrayList();
BankAccount object = new BankAccount();
//Set field here like
System.out.println("Enter your full name:");
name = sc.nextLine();
System.out.println("Choose an account number:");
accNum = sc.nextInt();
System.out.println("Enter an initiating amount:");
initiateAmount = sc.nextDouble();
經過簡單的使用
accounts.add(object);
在這種情況下,存儲通用數據類型或對象是完美的選擇,也可以在if -then- else情況下使用switch情況,以防止其易讀性和便利性
也使用增強的for循環查看帳戶
for(BankAccount account:accounts){
System.out.println("ALL DETAILS FROM OBJECT");
}
完整的代碼以及有效的答案
package tester;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class BankApp {
static class BankAccount {
private String name;
private int accNum;
private double initiateAmount;
public BankAccount() {
this.name = null;
this.accNum = 0;
this.initiateAmount = 0;
}
public void setAccNum(int accNum) {
this.accNum = accNum;
}
public void setInitiateAmount(double initiateAmount) {
this.initiateAmount = initiateAmount;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return name + "\t" + accNum + "\t" + initiateAmount;
}
}
public static void main(String[] args) {
List<BankAccount> bankAccounts = new ArrayList<BankAccount>();
Scanner sc = new Scanner(System.in);
while(true){
System.out.println("WELCOME TO OUR BANK!\n\n"
+ "Choose your option:\n"
+ "1. Create new account\n"
+ "2. View all the accounts property\n"
+ "3. Quit\n");
int option = sc.nextInt();
sc.nextLine();
switch(option){
case 1:
BankAccount account = new BankAccount();
System.out.println("Enter your full name:");
account.setName(sc.nextLine());
System.out.println("Choose an account number:");
account.setAccNum(sc.nextInt());
System.out.println("Enter an initiating amount:");
account.setInitiateAmount(sc.nextDouble());
bankAccounts.add(account);
break;
case 2:
System.out.println("Name\tAcc No\tAmount");
for (BankAccount bankAccount : bankAccounts) {
System.out.println(bankAccount);
}
System.out.println("\n\n");
break;
case 3:
return;
}
}
}
}
如果要將BankAccount
放在單獨的文件中,請執行以下操作。
文件BankAccount.java
public class BankAccount {
...
...
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.