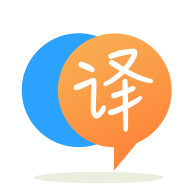
[英]How GoLang's typecast to interface implemented by a struct and embedded struct works
[英]In golang, How can I override the embedded struct's method
代碼在這里
package main
import "fmt"
func main() {
t16()
}
type Base struct {
val int
}
func (b *Base)Set(i int) {
b.val = i
}
type Sub struct {
Base
changed bool
}
func (b *Sub)Set(i int) {
b.val = i
b.changed = true
}
func t16() {
s := &Sub{}
s.Base.Set(1)
var b *Base = &s.Base
fmt.Printf("%+v\n", b)
fmt.Printf("%+v\n", s)
}
我想讓Sub充當Base,但是當我調用Set時,對於Sub它會標記已更改。我知道golang中沒有多態性或代理,但是有沒有辦法做到這一點,並且不影響Base?
更新
我希望當我調用 Base.Set 時它會標記更改,對於用戶來說,他們不知道他們實際上使用了 Sub,所以我可以監控 Base 的行為。
func t16() {
s := &Sub{}
var b *Base = &s.Base
b.Set(10)
fmt.Printf("%+v\n", b)
fmt.Printf("%+v\n", s)
}
通過讓Sub
嵌入Base
,它會自動將Base
的所有字段和函數作為Sub
的頂級成員提供。 這意味着您可以直接調用s.val
,並且您可以調用s.Set
來調用基本函數,除了Sub
實現了自己的Set
方法,它隱藏了基本函數。
當您在示例中調用s.Base.Set()
時,您將繞過Sub.Set()
並直接調用Base.Set()
。
在您的情況下修復它就像調用s.Set()
而不是s.Base.Set()
一樣簡單。
這對我有用:
func (b *Sub)Set(i int) {
b.Base.Set(i)
b.changed = true
}
func t16() {
s := &Sub{}
s.Set(1)
var b *Base = &s.Base
fmt.Printf("%+v\n", b)
fmt.Printf("%+v\n", s)
}
注意Sub.Set()
也可以調用嵌入的 structs 方法,這感覺很像其他 oo 語言提供的super()
類型繼承。
我想我應該在這里使用接口,它實現了我想要的,但是影響了基礎
func main() {
t16()
}
type Base interface {
Set(int)
}
type base struct {
val int
}
func (b *base)Set(i int) {
b.val = i
}
type Sub struct {
base
changed bool
}
func (b *Sub)Set(i int) {
b.val = i
b.changed = true
}
func t16() {
s := &Sub{}
s.Set(1)
var b Base = s
fmt.Printf("%+v\n", b)
fmt.Printf("%+v\n", s)
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.