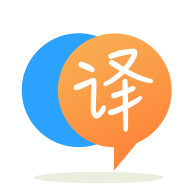
[英]Passing array as a parameter to an external ASM (Assembly) x86 and accessing it
[英]Assembly asm x86 Encryption/Decryption Program
我已經創建了這個程序已有一段時間了,我不知道如何解決解密程序,對您的幫助將不勝感激。
到目前為止,代碼的加密部分可以正常工作。
#include <conio.h> // for kbhit
#include <iostream> // for cin >> and cout <<
#include <iomanip> // for fancy output
using namespace std;
#define MAXCHARS 6 // feel free to alter this, but 6 is the minimum
#define dollarchar '$' // string terminator
char OChars[MAXCHARS],
EChars[MAXCHARS],
DChars[MAXCHARS] = "Soon!"; // Global Original, Encrypted, Decrypted character strings
//----------------------------- C++ Functions ----------------------------------------------------------
void get_char (char& a_character)
{
cin >> a_character;
while (((a_character < '0') | (a_character > 'z')) && (a_character != dollarchar))
{ cout << "Alphanumeric characters only, please try again > ";
cin >> a_character;
}
}
//-------------------------------------------------------------------------------------------------------------
void get_original_chars (int& length)
{ char next_char;
length = 0;
get_char (next_char);
while ((length < MAXCHARS) && (next_char != dollarchar))
{
OChars [length++] = next_char;
get_char (next_char);
}
}
//---------------------------------------------------------------------------------------------------------------
//----------------- ENCRYPTION ROUTINES -------------------------------------------------------------------------
void encrypt_chars (int length, char EKey)
{ char temp_char; // char temporary store
for (int i = 0; i < length; i++) // encrypt characters one at a time
{
temp_char = OChars [i]; //
__asm { //
push eax // save register values on stack to be safe
push ecx // Push last parameter first
lea eax,EKey
push temp_char
push eax
call encrypt3 // encrypt the character
mov temp_char, al
add esp, 8 // Clean parameters from stack
pop ecx // restore original register values from stack
pop eax //
}
EChars [i] = temp_char; // Store encrypted char in the encrypted chars array
}
return;
__asm {
encrypt3:
push ebp // Save the old base pointer value
mov ebp, esp // Set the new base pointer value
push edx // Save EDX to the first unused empty stack
push ecx //ecx register containing the temp_char is pushed to the stack
push eax // Save EAX to the first unused empty stack
mov edx, [ebp + 8] // Accessing the last value of ebp
movzx eax, byte ptr[eax] // Move 4 bytes to the EAX register
rol al, 1 // Rotate AL register one position to the left
rol al, 1 // Rotate AL register one position to the left
rol al, 1 // Rotate AL register one position to the left
mov edx, eax // Move 4 bytes from EAX into edx
pop eax // Restore original EAX
mov byte ptr[eax], dl //moves the Ekey value into the EAX register as an 8-bit value
pop ecx //stores the current letter being encrypted within the ECX register (it was pushed to the stack earlier in the assembly code).
xor ecx, edx //clears the EDX register of all values
mov eax, ecx // Move 4 bytes from ECX into EAX
ror al, 1 // Rotate AL register one position to the left
ror al, 1 // Rotate AL register one position to the left
ror al, 1 // Rotate AL register one position to the left
pop edx // Restore the value of EDX
pop ebx // Restore original EBX
mov esp, ebp // Dellocate local variables
pop ebp // Restore the original value of EBP
ret // Return EAX value
}
//--- End of Assembly code
}
// end of encrypt_chars function
//---------------------------------------------------------------------------------------------------------------
//---------------------------------------------------------------------------------------------------------------
//----------------- DECRYPTION ROUTINES -------------------------------------------------------------------------
//
void decrypt_chars(int length, char EKey)
{
return;
}
// end of decrypt_chars function
//---------------------------------------------------------------------------------------------------------------
當然,在放入匯編語言之前,請先用高級語言編寫代碼。
原因如下:
edx
寄存器 mov edx, [ebp + 8] // Accessing the last value of ebp
movzx eax, byte ptr[eax] // Move 4 bytes to the EAX register
rol al, 1 // Rotate AL register one position to the left
rol al, 1 // Rotate AL register one position to the left
rol al, 1 // Rotate AL register one position to the left
mov edx, eax // Move 4 bytes from EAX into edx
在上面的代碼中,將[ebp + 8]
移到edx
。 然后,您稍后將eax
復制到edx
四個指令。 為什么要打擾這里的第一條指令?
使用匯編語言進行編碼的常見原因之一是效率。 據說您可以比編譯器更好地編碼,或者可以使用比編譯器更好的特殊指令。 如以下示例所示,您不需要:
rol al, 1 // Rotate AL register one position to the left
rol al, 1 // Rotate AL register one position to the left
rol al, 1 // Rotate AL register one position to the left
上面的代碼應編碼為rol al, 3
。
另外,您是否有理由使用al
register代替eax
?
清除edx
寄存器錯誤操作與注釋不匹配。
xor ecx, edx //clears the EDX register of all values
edx
寄存器位於錯誤的一側。
語句xor edx, edx
實際上清除edx
寄存器。
我建議您破壞匯編語言,並以高級語言重寫功能。 首先使它工作。 檢查編譯器的匯編語言。 如果您可以比編譯器更有效地編碼算法,則可以這樣做。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.