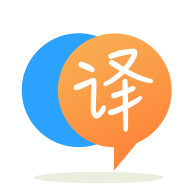
[英]Android RecyclerView.Adapter onCreateViewHolder() working
[英]What is the SortedList<T> working with RecyclerView.Adapter?
Android支持庫22.1已於昨天發布。 v4支持庫和v7中添加了許多新功能,其中android.support.v7.util.SortedList<T>
引起了我的注意。
據說, SortedList
是一個新的數據結構,工作原理RecyclerView.Adapter
,維護項目添加/刪除/移動/改變所提供的動畫RecyclerView
。 聽起來像ListView
的List<T>
,但似乎更高級,更強大。
那么, SortedList<T>
和List<T>
什么區別? 如何有效使用它? 如果是這樣,那么對List<T>
的SortedList<T>
的強制執行是什么? 有人可以張貼一些樣品嗎?
任何提示或代碼將不勝感激。 提前致謝。
SortedList
通過Callback
處理與Recycler適配器的通信。
在下面的示例的addAll
幫助器方法中可以看到SortedList
和List
之間的區別。
public void addAll(List<Page> items) {
mPages.beginBatchedUpdates();
for (Page item : items) {
mPages.add(item);
}
mPages.endBatchedUpdates();
}
假設在填充回收商列表時,我有10個緩存項目要立即加載。 同時,我在網絡中查詢相同的10個項目,因為自從將它們緩存以來,它們可能已經更改。 我可以調用相同addAll
方法和SortedList
將取代在引擎蓋下與fetchedItems的cachedItems(始終保持最后添加的項目)。
// After creating adapter
myAdapter.addAll(cachedItems)
// Network callback
myAdapter.addAll(fetchedItems)
在常規List
,我將擁有所有項目的副本(列表大小為20)。 使用SortedList
它使用Callback的areItemsTheSame
替換相同的areItemsTheSame
。
添加fetchedItems時,僅當Page
標題中的一個或多個更改時,才會調用onChange
。 您可以自定義SortedList
在回調的areContentsTheSame
查找的areContentsTheSame
。
如果你要多個項目添加到排序列表,BatchedCallback調用轉換個別onInserted(指數1)呼叫到一個onInserted(索引,N),如果項目被加入到連續的指數。 這種變化可以幫助解決RecyclerView變化更容易。
您可以在適配器上為SortedList
設置一個吸氣劑,但是我只是決定向適配器添加輔助方法。
適配器類別:
public class MyAdapter extends RecyclerView.Adapter<RecyclerView.ViewHolder> {
private SortedList<Page> mPages;
public MyAdapter() {
mPages = new SortedList<Page>(Page.class, new SortedList.Callback<Page>() {
@Override
public int compare(Page o1, Page o2) {
return o1.getTitle().compareTo(o2.getTitle());
}
@Override
public void onInserted(int position, int count) {
notifyItemRangeInserted(position, count);
}
@Override
public void onRemoved(int position, int count) {
notifyItemRangeRemoved(position, count);
}
@Override
public void onMoved(int fromPosition, int toPosition) {
notifyItemMoved(fromPosition, toPosition);
}
@Override
public void onChanged(int position, int count) {
notifyItemRangeChanged(position, count);
}
@Override
public boolean areContentsTheSame(Page oldItem, Page newItem) {
// return whether the items' visual representations are the same or not.
return oldItem.getTitle().equals(newItem.getTitle());
}
@Override
public boolean areItemsTheSame(Page item1, Page item2) {
return item1.getId() == item2.getId();
}
});
}
@Override
public RecyclerView.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext())
.inflate(R.layout.viewholder_page, parent, false);
return new PageViewHolder(view);
}
@Override
public void onBindViewHolder(RecyclerView.ViewHolder holder, int position) {
PageViewHolder pageViewHolder = (PageViewHolder) holder;
Page page = mPages.get(position);
pageViewHolder.textView.setText(page.getTitle());
}
@Override
public int getItemCount() {
return mPages.size();
}
// region PageList Helpers
public Page get(int position) {
return mPages.get(position);
}
public int add(Page item) {
return mPages.add(item);
}
public int indexOf(Page item) {
return mPages.indexOf(item);
}
public void updateItemAt(int index, Page item) {
mPages.updateItemAt(index, item);
}
public void addAll(List<Page> items) {
mPages.beginBatchedUpdates();
for (Page item : items) {
mPages.add(item);
}
mPages.endBatchedUpdates();
}
public void addAll(Page[] items) {
addAll(Arrays.asList(items));
}
public boolean remove(Page item) {
return mPages.remove(item);
}
public Page removeItemAt(int index) {
return mPages.removeItemAt(index);
}
public void clear() {
mPages.beginBatchedUpdates();
//remove items at end, to avoid unnecessary array shifting
while (mPages.size() > 0) {
mPages.removeItemAt(mPages.size() - 1);
}
mPages.endBatchedUpdates();
}
}
頁面類:
public class Page {
private String title;
private long id;
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
}
觀看者xml:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/text_view"
style="@style/TextStyle.Primary.SingleLine"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
觀看者類別:
public class PageViewHolder extends RecyclerView.ViewHolder {
public TextView textView;
public PageViewHolder(View itemView) {
super(itemView);
textView = (TextView)item.findViewById(R.id.text_view);
}
}
v7 support library
SortedList
。
一個
SortedList
實現,它可以使項目保持順序,並通知列表中的更改,以便可以將其綁定到RecyclerView.Adapter
。它使用
compare(Object, Object)
方法使項目保持有序compare(Object, Object)
並使用二進制搜索來檢索項目。 如果項目的排序標准可能會更改,請確保在編輯它們時調用適當的方法,以避免數據不一致。您可以通過
SortedList.Callback
參數控制項目的順序並更改通知。
下面是使用SortedList
的示例,我認為這是您想要的,看看並享受吧!
public class SortedListActivity extends ActionBarActivity {
private RecyclerView mRecyclerView;
private LinearLayoutManager mLinearLayoutManager;
private SortedListAdapter mAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.sorted_list_activity);
mRecyclerView = (RecyclerView) findViewById(R.id.recycler_view);
mRecyclerView.setHasFixedSize(true);
mLinearLayoutManager = new LinearLayoutManager(this);
mRecyclerView.setLayoutManager(mLinearLayoutManager);
mAdapter = new SortedListAdapter(getLayoutInflater(),
new Item("buy milk"), new Item("wash the car"),
new Item("wash the dishes"));
mRecyclerView.setAdapter(mAdapter);
mRecyclerView.setHasFixedSize(true);
final EditText newItemTextView = (EditText) findViewById(R.id.new_item_text_view);
newItemTextView.setOnEditorActionListener(new TextView.OnEditorActionListener() {
@Override
public boolean onEditorAction(TextView textView, int id, KeyEvent keyEvent) {
if (id == EditorInfo.IME_ACTION_DONE &&
(keyEvent == null || keyEvent.getAction() == KeyEvent.ACTION_DOWN)) {
final String text = textView.getText().toString().trim();
if (text.length() > 0) {
mAdapter.addItem(new Item(text));
}
textView.setText("");
return true;
}
return false;
}
});
}
private static class SortedListAdapter extends RecyclerView.Adapter<TodoViewHolder> {
SortedList<Item> mData;
final LayoutInflater mLayoutInflater;
public SortedListAdapter(LayoutInflater layoutInflater, Item... items) {
mLayoutInflater = layoutInflater;
mData = new SortedList<Item>(Item.class, new SortedListAdapterCallback<Item>(this) {
@Override
public int compare(Item t0, Item t1) {
if (t0.mIsDone != t1.mIsDone) {
return t0.mIsDone ? 1 : -1;
}
int txtComp = t0.mText.compareTo(t1.mText);
if (txtComp != 0) {
return txtComp;
}
if (t0.id < t1.id) {
return -1;
} else if (t0.id > t1.id) {
return 1;
}
return 0;
}
@Override
public boolean areContentsTheSame(Item oldItem,
Item newItem) {
return oldItem.mText.equals(newItem.mText);
}
@Override
public boolean areItemsTheSame(Item item1, Item item2) {
return item1.id == item2.id;
}
});
for (Item item : items) {
mData.add(item);
}
}
public void addItem(Item item) {
mData.add(item);
}
@Override
public TodoViewHolder onCreateViewHolder(final ViewGroup parent, int viewType) {
return new TodoViewHolder (
mLayoutInflater.inflate(R.layout.sorted_list_item_view, parent, false)) {
@Override
void onDoneChanged(boolean isDone) {
int adapterPosition = getAdapterPosition();
if (adapterPosition == RecyclerView.NO_POSITION) {
return;
}
mBoundItem.mIsDone = isDone;
mData.recalculatePositionOfItemAt(adapterPosition);
}
};
}
@Override
public void onBindViewHolder(TodoViewHolder holder, int position) {
holder.bindTo(mData.get(position));
}
@Override
public int getItemCount() {
return mData.size();
}
}
abstract private static class TodoViewHolder extends RecyclerView.ViewHolder {
final CheckBox mCheckBox;
Item mBoundItem;
public TodoViewHolder(View itemView) {
super(itemView);
mCheckBox = (CheckBox) itemView;
mCheckBox.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if (mBoundItem != null && isChecked != mBoundItem.mIsDone) {
onDoneChanged(isChecked);
}
}
});
}
public void bindTo(Item item) {
mBoundItem = item;
mCheckBox.setText(item.mText);
mCheckBox.setChecked(item.mIsDone);
}
abstract void onDoneChanged(boolean isChecked);
}
private static class Item {
String mText;
boolean mIsDone = false;
final public int id;
private static int idCounter = 0;
public Item(String text) {
id = idCounter ++;
this.mText = text;
}
}
}
支持庫源存儲庫中有一個示例SortedListActivity,該示例演示了如何在RecyclerView.Adapter中使用SortedList和SortedListAdapterCallback。 從SDK的根目錄開始,安裝了支持庫后,它應該位於extras/android/support/samples/Support7Demos/src/com/example/android/supportv7/util/SortedListActivity.java
(也在github上 )。
關於SortedList
實現,它由<T>
數組支持,默認最小容量為10個項目。 陣列裝滿后,將其大小調整為size() + 10
源代碼在這里
從文檔
Sorted list實現,可以使項目保持順序,並通知列表中的更改,以便可以將其綁定到RecyclerView.Adapter。
它使用compare(Object,Object)方法使項目保持有序,並使用二進制搜索來檢索項目。 如果項目的排序標准可能會更改,請確保在編輯它們時調用適當的方法,以避免數據不一致。
您可以通過SortedList.Callback參數控制項目的順序並更改通知。
關於性能,他們還添加了SortedList.BatchedCallback一次執行多個操作,而不是一次執行
可以批量通知SortedList調度的事件的回調實現。
如果您要對SortedList進行多個操作,但又不想一個一個地分派每個事件,則這可能會導致性能問題,該類很有用。
例如,如果要將多個項目添加到SortedList,則將項目添加到連續索引中時,BatchedCallback調用將單個onInserted(index,1)調用轉換為一個onInserted(index,N)。 這種變化可以幫助解決RecyclerView變化更容易。
如果SortedList中的連續更改不適合進行批處理,則在檢測到這種情況后,BatchingCallback會立即分派它們。 在SortedList上的編輯完成后,必須始終調用dispatchLastEvent()來刷新對回調的所有更改。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.