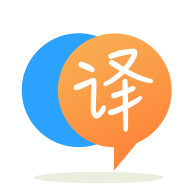
[英]How do i take user input and store it successfully in an ArrayList? Then how do i get my program to show me all the elements in the ArrayList?
[英]How do I take user input and show it on my array?
我正在創建飛機座位表。 我能夠顯示圖表,並可以要求用戶輸入。 我正在嘗試接受他們的回答,並在其輸入狀態處輸入“ X”。 我不知道如何接受他們的輸入並將其顯示在圖表上,然后將其替換為X。
import java.util.Scanner;
class AirplaneSeating {
public static void main(String[] args) {
String[][] seatingChart = new String[10][4];
int rows = 10;
int columns = 4;
Scanner inStr = new Scanner(System.in);
seatingChart = new String[rows][columns];
seatingChart[0][0] = "A1";
seatingChart[0][1] = "A2";
seatingChart[0][2] = "A3";
seatingChart[0][3] = "A4";
seatingChart[1][0] = "B1";
seatingChart[1][1] = "B2";
seatingChart[1][2] = "B3";
seatingChart[1][3] = "B4";
seatingChart[2][0] = "C1";
seatingChart[2][1] = "C2";
seatingChart[2][2] = "C3";
seatingChart[2][3] = "C4";
seatingChart[3][0] = "D1";
seatingChart[3][1] = "D2";
seatingChart[3][2] = "D3";
seatingChart[3][3] = "D4";
seatingChart[4][0] = "E1";
seatingChart[4][1] = "E2";
seatingChart[4][2] = "E3";
seatingChart[4][3] = "E4";
seatingChart[5][0] = "F1";
seatingChart[5][1] = "F2";
seatingChart[5][2] = "F3";
seatingChart[5][3] = "F4";
seatingChart[6][0] = "G1";
seatingChart[6][1] = "G2";
seatingChart[6][2] = "G3";
seatingChart[6][3] = "G4";
seatingChart[7][0] = "H1";
seatingChart[7][1] = "H2";
seatingChart[7][2] = "H3";
seatingChart[7][3] = "H4";
seatingChart[8][0] = "I1";
seatingChart[8][1] = "I2";
seatingChart[8][2] = "I3";
seatingChart[8][3] = "I4";
seatingChart[9][0] = "J1";
seatingChart[9][1] = "J2";
seatingChart[9][2] = "J3";
seatingChart[9][3] = "J4";
for(int i = 0; i < rows ; i++) {
for(int j = 0; j < columns ; j++) {
System.out.print(seatingChart[i][j] + " ");
}
System.out.println("");
}
System.out.println("What seat would you like to reserve? ");
String str = inStr.nextLine();
System.out.println("You chose: " + str);
}
}
您只想顯示任何方式? 您想如何輸入? 可以嗎
您只需解析掃描器輸入,並告訴用戶將行,列放在您的代碼中即可。
//Example: Input: 6,2
//Split the user input
String[] input = str.split(",");
//parse first number to make it the row
int rowuser = Integer.parseInt(input[0].trim());
//parse second number to make it the column
int columnuser = Integer.parseInt(input[1].trim());
//replace with "X" value the seatingChart[row][column]
seatingChart[rowuser][columnuser] = "X"
但是您仍然需要循環才能再次顯示座位表。
查看您的程序和座位的結構方式,您可以通過簡單地解析和存儲輸入來實現。
char firstChar = str.toUpperCase().charAt(0);
char secondChar = str.charAt(1);
int first = ((int) firstChar) - 101; // ascii value - 101 for upper case A
int second = ((int) secondChar) - 1; // cast the character to an int, then -1 because it's 0 indexed.
那么您要做的就是將值設置為
seatingChart[first][second] = "X";
然后,您可以再次打印出來。
確保str是兩個字符。 取第一個字符(我猜它將是字母字符),將其轉換為數字,並將其存儲為變量(例如x)。 對第二個字符(例如,y)執行相同的操作。 設置SeatingChart [x] [y] =“ X”,然后再次運行顯示循環。
我希望這不是一個很具體的答案,因為這顯然是家庭作業。
考慮創建HashMap<Character, Integer>
將行字母映射到相應的數字。 給定用戶輸入,獲取第一個字符並從哈希映射中獲取適當的行索引。 使用庫函數將第二個字符轉換為int
。 現在,您已經在數組中添加了索引,並且可以更改相關值。
該代碼段可能會有所幫助
假設在定義座位時給出輸入。 例如:H4
Scanner sc = new Scanner(System.in);
String input = sc.nextLine();
int x = (int)input.charAt(0)-65; //since 'A' has ASCII value of 65
int y = (int)input.charAt(1)-49; //since '0' has ASCII value of 49
// update seatingChart[x][y]
你可以初始化這個
seatingChart = new String[rows][columns];
seatingChart[0][0] = "A1";
seatingChart[0][1] = "A2";
seatingChart[0][2] = "A3";
seatingChart[0][3] = "A4";
seatingChart[1][0] = "B1";
seatingChart[1][1] = "B2";
seatingChart[1][2] = "B3";
seatingChart[1][3] = "B4";
seatingChart[2][0] = "C1";
seatingChart[2][1] = "C2";
seatingChart[2][2] = "C3";
seatingChart[2][3] = "C4";
seatingChart[3][0] = "D1";
seatingChart[3][1] = "D2";
seatingChart[3][2] = "D3";
seatingChart[3][3] = "D4";
seatingChart[4][0] = "E1";
seatingChart[4][1] = "E2";
seatingChart[4][2] = "E3";
seatingChart[4][3] = "E4";
seatingChart[5][0] = "F1";
seatingChart[5][1] = "F2";
seatingChart[5][2] = "F3";
seatingChart[5][3] = "F4";
seatingChart[6][0] = "G1";
seatingChart[6][1] = "G2";
seatingChart[6][2] = "G3";
seatingChart[6][3] = "G4";
seatingChart[7][0] = "H1";
seatingChart[7][1] = "H2";
seatingChart[7][2] = "H3";
seatingChart[7][3] = "H4";
seatingChart[8][0] = "I1";
seatingChart[8][1] = "I2";
seatingChart[8][2] = "I3";
seatingChart[8][3] = "I4";
seatingChart[9][0] = "J1";
seatingChart[9][1] = "J2";
seatingChart[9][2] = "J3";
seatingChart[9][3] = "J4";
像這樣:
String[][] seatingChart = new String[rows][columns];
for(int i = 0; i < rows; i++) {
for(int j = 0; j < columns; j++) {
seatingChart[i][j] = "" + ((char)('A' + i)) + ((char)('1' + j));
}
}
問題的答案是,一旦有了他們的輸入行:
System.out.println("What seat would you like to reserve? ");
String str = inStr.nextLine();
然后,您只需要遍歷數組,並找到一個匹配項:
for(int i = 0; i < rows ; i++) {
for(int j = 0; j < columns ; j++) {
if(seatingChart[i][j].equals(str)) {
System.out.print("XX" + " ");
} else {
System.out.print(seatingChart[i][j] + " ");
}
}
System.out.println("");
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.