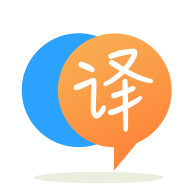
[英]Javascript?:How to dynamically add text inputs/form fields to a HTML form?
[英]Dynamically add set of inputs to html form
我當前正在創建HTML表單,我有一個部分包含8個文本輸入。 這是到目前為止的部分:
<div class="form-title"><h3>Clinical Information</h3></div>
<div class="form-title">Time Assesed:</div>
<input class="form-field" type="time" name="timeassessd" /><br />
<div class="form-title">Blood Pressure:</div>
<input class="form-field" type="text" name="bp" /><br />
<div class="form-title">Pulse:</div>
<input class="form-field" type="date" name="pulse" /><br />
<div class="form-title">Resp. Rate:</div>
<input class="form-field" type="text" name="breathing" /><br />
<div class="form-title">Temp:</div>
<input class="form-field" type="text" name="temp" /><br />
<div class="form-title">SPO2:</div>
<input class="form-field" type="text" name="spo2" /><br />
<div class="form-title">GCS:</div>
<input class="form-field" type="text" name="gcs" /><br />
<div class="form-title">AVPU:</div>
<input class="form-field" type="text" name="avpu" /><br />
我需要的是一個按鈕,當用戶按下該按鈕時,它將創建與上面相同的另一部分,將字段添加到表單中。 每個表格的名稱末尾也需要一個數字。 我瀏覽過不同的論壇,但找不到完整的部分來添加單獨的輸入,這對我沒有幫助。 謝謝。
您需要制作一個JS函數來添加該部分。 該函數將如下所示:
function add_section() {
new_row = "";
new_row += "";
new_row += '<div class="form-title"><h3>Clinical Information</h3></div>';
new_row += '<div class="form-title">Time Assesed:</div>';
new_row += '<input class="form-field" type="time" name="timeassessd" /><br />';
new_row += '<div class="form-title">Blood Pressure:</div>';
new_row += '<input class="form-field" type="text" name="bp" /><br />';
new_row += '<div class="form-title">Pulse:</div>';
new_row += '<input class="form-field" type="date" name="pulse" /><br />';
new_row += '<div class="form-title">Resp. Rate:</div>';
new_row += '<input class="form-field" type="text" name="breathing" /><br />';
new_row += '<div class="form-title">Temp:</div>';
new_row += '<input class="form-field" type="text" name="temp" /><br />';
new_row += '<div class="form-title">SPO2:</div>';
new_row += '<input class="form-field" type="text" name="spo2" /><br />';
new_row += '<div class="form-title">GCS:</div>';
new_row += '<input class="form-field" type="text" name="gcs" /><br />';
new_row += '<div class="form-title">AVPU:</div>';
new_row += '<input class="form-field" type="text" name="avpu" /><br />';
var pos = $("selecter"); //element selecter after which you need to add the section
$(pos).after(new_row);
}
然后單擊按鈕,調用此函數。 它會工作。
輸入字段的名稱也應為數組ex: name="avpu[]"
如果不使用數組,則post方法將僅獲取具有相同名稱的最后一個輸入元素的值。
您能張貼整個表格嗎?
如果使用的是jQuery,您可以做的就是克隆表單的jQuery節點,然后操作輸入名稱,然后將內容附加到表單中。
像這樣:
var num = 1;
function add_form_elements(num) {
var clonedForm = $('#id_of_the_form').clone();
clonedForm.find('input').each(function(id, elm) {
elm.attr("name",elm.attr("name") + num);
});
$('#id_of_the_form').append(clonedForm.innerHTML);
num++;
});
比您需要將EventListener添加到您的按鈕並將add_form_elements函數綁定到它。
新建一個div,其中所有要復制的內容都是不可見的。
<div class="copyable" style="display: none;">
<div class="form-title"><h3>Clinical Information</h3></div>
<div class="form-title">Time Assesed:</div>
<input class="form-field" type="time" name="timeassessd" /><br />
<div class="form-title">Blood Pressure:</div>
<input class="form-field" type="text" name="bp" /><br />
<div class="form-title">Pulse:</div>
<input class="form-field" type="date" name="pulse" /><br />
<div class="form-title">Resp. Rate:</div>
<input class="form-field" type="text" name="breathing" /><br />
<div class="form-title">Temp:</div>
<input class="form-field" type="text" name="temp" /><br />
<div class="form-title">SPO2:</div>
<input class="form-field" type="text" name="spo2" /><br />
<div class="form-title">GCS:</div>
<input class="form-field" type="text" name="gcs" /><br />
<div class="form-title">AVPU:</div>
<input class="form-field" type="text" name="avpu" /><br />
</div>
在JS文件中:
function add_elm(){
var content = $('.copyable').html(),
$elm = $('.elm'); //element where you want to add copyable content
$elm.append(content);
}
注意:append用於在父節點內附加html。 如果要在其他Elm之后添加html,只需在上述代碼末尾使用after(content)
。
以下是使用普通JavaScript解決問題的一種方法(請記住,這確實需要對HTML進行更改,因為包含要復制的<input>
元素的部分需要一個類名(此處為"clinicalInformation"
,但要根據自己的要求進行調整-記住更改<button>
元素的data-duplicates
屬性中保留的選擇器):
// the event is passed automagically from the addEventListener()
// method:
function duplicate(event) {
// preventing the clicked-element's default behaviour
// (which in many cases could cause a page reload):
event.preventDefault();
// using Array.prototype.slice, with Function.prototype.call,
// on the NodeList returned by document.querySelectorAll(),
// to create an array of element nodes;
// 'this.dataset.duplicates' retrieves the attribute-value from
// the 'data-duplicates' attribute of the clicked element:
var allCurrent = Array.prototype.slice.call(document.querySelectorAll(this.dataset.duplicates), 0),
// getting the last element from the array of nodes:
toClone = allCurrent[allCurrent.length - 1],
// cloning that node, including its child elements:
clone = toClone.cloneNode(true),
// creating a RegExp (regular expression) literal,
// to match a sequence of one, or more, numbers (\d+)
// followed by the end of the string ($):
reg = /\d+$/,
// creating an 'empty'/unitialised variable for use
// within the (later) loop:
childElems;
// adding the created clone to the allCurrent array:
allCurrent.push(clone);
// using Array.prototype.forEach() to iterate over the
// array, the arguments (names are user-defined):
// - first (here 'fieldset') is the current array element
// over which we're iterating,
// - second (here 'index') is the index of the current
// array element in the array:
allCurrent.forEach(function(fieldset, index) {
// finding all descendant elements contained within
// the current array-element that have a 'name' attribute,
// using a CSS attribute-selector within
// document.querySelectorAll():
childElems = fieldset.querySelectorAll('[name]');
// iterating over those descendant elements in the
// array-like NodeList:
Array.prototype.forEach.call(childElems, function(elem) {
// if the regular expression matches the name (
// RegExp.prototype.test() returning a Boolean true/false
// based on the string matching, or not matching, the
// regular expression) we replace that match with the index
// (from the outer loop), or if not we simply append the
// index to the current name property-value:
elem.name = reg.test(elem.name) ? elem.name.replace(reg, index) : elem.name + index;
});
// navigating from the cloned node to its parent and, using
// Node.insertBefore(), we insert the created clone before
// the nextSibling of that cloned node:
toClone.parentNode.insertBefore(clone, toClone.nextSibling);
});
}
// getting a reference to the element that should trigger
// the duplication:
var addMore = document.getElementById('duplicate');
// adding an event-handler for the 'click' event
// (note the lack of parentheses):
addMore.addEventListener('click', duplicate)
function duplicate(event) { event.preventDefault(); var allCurrent = Array.prototype.slice.call(document.querySelectorAll(this.dataset.duplicates), 0), toClone = allCurrent[allCurrent.length - 1], clone = toClone.cloneNode(true), reg = /\\d+$/, childElems; allCurrent.push(clone); allCurrent.forEach(function(fieldset, index) { childElems = fieldset.querySelectorAll('[name]'); Array.prototype.forEach.call(childElems, function(elem) { elem.name = reg.test(elem.name) ? elem.name.replace(reg, index) : elem.name + index; }); toClone.parentNode.insertBefore(clone, toClone.nextSibling); }); } var addMore = document.getElementById('duplicate'); addMore.addEventListener('click', duplicate)
label { display: block; }
<!-- here we're using an actual <form> element --> <form action="#" method="post"> <!-- using a fieldset to group the related fields together --> <fieldset class="clinicalInformation"> <!-- the <legend> element semantically titles that group of related fields --> <legend class="form-title"> Clinical Information </legend> <!-- the <label> semantically associates a text-label with a specific form-element; that form-element (<input />, <textarea>, <select>) can appear within the <label>, or the <label> can have a 'for' attribute equal to the 'id' of the associated element. --> <label class="form-title">Time Assesed: <input class="form-field" type="time" name="timeassessd" /> </label> <label class="form-title">Blood Pressure: <input class="form-field" type="text" name="bp" /> </label> <label class="form-title">Pulse: <input class="form-field" type="date" name="pulse" /> </label> <label class="form-title">Resp. Rate: <input class="form-field" type="text" name="breathing" /> </label> <label class="form-title">Temp: <input class="form-field" type="text" name="temp" /> </label> <label class="form-title">SPO<sub>2</sub>: <input class="form-field" type="text" name="spo2" /> </label> <label class="form-title">GCS: <input class="form-field" type="text" name="gcs" /> </label> <label class="form-title">AVPU: <input class="form-field" type="text" name="avpu" /> </label> </fieldset> <fieldset> <button id="duplicate" data-duplicates=".clinicalInformation">Add more</button> </fieldset> </form>
但是,由於使用了jQuery標簽,因此使用了jQuery:
// binding an anonymous click event-handler, using on():
$('#duplicate').on('click', function(event) {
// preventing the default action:
event.preventDefault();
// finding all elements that match the selector from
// the clicked-element's 'data-duplicates' attribute:
var allCurrent = $(this.dataset.duplicates),
// finding the last of those elements:
toClone = allCurrent.last(),
// creating the clone, including the child elements:
clone = toClone.clone(true),
// the regular expression (as above):
reg = /\d+$/;
// adding the clone to the 'allCurrent' collection,
// then iterating over them with each(), getting a
// reference to the index of each element in the collection:
allCurrent.add(clone).each(function(index) {
// finding all descendant elements that have a name attribute,
// updating the 'name' property of each of those found
// elements using prop():
$(this).find('[name]').prop('name', function(i, n) {
// the first argument (here: 'i') is the index of the
// current element in the collection,
// the second (here: 'n') is the current value of the
// current element's property.
// exactly the same as above:
return reg.test(n) ? n.replace(reg, index) : n + index;
});
});
// inserting the clone into the document
// after the toClone element:
clone.insertAfter(toClone);
});
$('#duplicate').on('click', function(event) { event.preventDefault(); var allCurrent = $(this.dataset.duplicates), toClone = allCurrent.last(), clone = toClone.clone(true), reg = /\\d+$/; allCurrent.add(clone).each(function(index) { $(this).find('[name]').prop('name', function(i, n) { return reg.test(n) ? n.replace(reg, index) : n + index; }); }); clone.insertAfter(toClone); });
label { display: block; }
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <form action="#" method="post"> <fieldset class="clinicalInformation"> <legend class="form-title"> Clinical Information </legend> <label class="form-title">Time Assesed: <input class="form-field" type="time" name="timeassessd" /> </label> <label class="form-title">Blood Pressure: <input class="form-field" type="text" name="bp" /> </label> <label class="form-title">Pulse: <input class="form-field" type="date" name="pulse" /> </label> <label class="form-title">Resp. Rate: <input class="form-field" type="text" name="breathing" /> </label> <label class="form-title">Temp: <input class="form-field" type="text" name="temp" /> </label> <label class="form-title">SPO<sub>2</sub>: <input class="form-field" type="text" name="spo2" /> </label> <label class="form-title">GCS: <input class="form-field" type="text" name="gcs" /> </label> <label class="form-title">AVPU: <input class="form-field" type="text" name="avpu" /> </label> </fieldset> <fieldset> <button id="duplicate" data-duplicates=".clinicalInformation">Add more</button> </fieldset> </form>
參考文獻:
data-*
屬性嵌入自定義非可見數據 。 <fieldset>
。 <label>
。 <legend>
。 data
屬性 。 Array.prototype.forEach()
。 Array.prototype.slice()
。 document.querySelectorAll()
。 Event.preventDefault()
。 EventTarget.addEventListener()
。 Function.prototype.call()
。 HTMLElement.dataset
。 Node.cloneNode
。 Node.insertBefore
。 Node.parentNode
。 RegExp
RegExp.prototype.test()
。 String.prototype.replace()
。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.