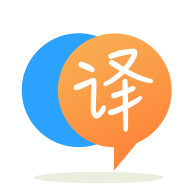
[英]In C/C++, is char* arrayName[][] a pointer to a pointer to a pointer OR a pointer to a pointer?
[英]C++ Pointer Char
我是C ++的新手,現在正在學習指針。 我正在嘗試了解該程序:
#include <iostream>
int main() {
char *text = "hello world";
int i = 0;
while (*text) {
i++;
text++;
}
printf("Char num of <%s> = %d", text, i);
}
它輸出:
Char num of <> = 11
但是為什么不這樣:
Char num of <hello world> = 11
當您增加text
直到它指向'\\0'
,打印的字符串為空。
您在打印出來之前更改了text
的值:
#include <iostream>
int main() {
// text starts pointing at the beginnin of "hello world"
char *text = "hello world"; // this should be const char*
int i = 0;
while (*text) {
i++;
// text moves forward one character each time in the loop
text++;
}
// now text is pointing to the end of the "hello world" text so nothing to print
printf("Char num of <%s> = %d", text, i);
}
char指針(如text
)的值是它指向的字符的位置。 如果將text
加一(使用text++
),則它指向下一個字符的位置。
不知道為什么#include <iostream>
但使用printf()
。 通常,在C++
代碼中,您可以這樣做:
std::cout << "Char num of <" << text << "> = " << i << '\n';
您嘗試做的工作示例:
int main()
{
const char* text = "hello world"; // should be const char*
int length = 0;
for(const char* p = text; *p; ++p)
{
++length;
}
std::cout << "Char num of <" << text << "> = " << length << '\n';
}
首先-不要在C ++中使用char-string! 使用std :: string。
您的while循環將繼續直至達到零(這是字符串終止),因此%s只是一個空字符串。 即使字符串為空,仍會打印“ <”和“>”。
您的文本指針從以下字符開始:
'h','e','l','l','o',' ','w','o','r','l','d','\0'
在第一個循環之后,文本指向:
'e','l','l','o',' ','w','o','r','l','d','\0'
第二次循環后,文本指向:
'l','l','o',' ','w','o','r','l','d','\0'
等等。
while循環繼續進行,直到文本指向“ \\ 0”,這只是一個空字符串,即“”。
因此,%s不會打印任何內容。
在C ++中執行以下操作:
int main() {
std::string text = "hello world";
cout << "Char num of <" << text << "> = " << text.size() << std::endl;
}
text
是指向char
的指針。 因此,當您增加指針時,便使其指向下一個元素。 C中字符串的結尾由空字符定界。 因此,您要增加指針直到它指向空字符。 此后,當您調用printf
它不會打印任何內容,因為它會打印字符直到到達空字符,但已經在空字符處。
這可能是一個快速解決方案:
#include <stdio.h>
int main() {
char *text = "hello world";
int i = 0;
while (*text) {
i++;
text++;
}
printf("Char num of <%s> = %d", text-i, i);
}
循環指針之后的text
指向字符串文字的終止零,因為它在循環中增加了。
另外,在頭文件<cstdio>
聲明了printf
,並且C ++中的字符串常量具有常量數組的類型(盡管在C中,它們具有非常量數組的類型。但是,在兩種語言中,您都不能更改字符串常量)
您可以像這樣重寫程序
#include <cstdio>
int main() {
const char *text = "hello world";
int i = 0;
while ( text[i] ) ++i;
std::printf("Char num of <%s> = %d\n", text, i);
}
或喜歡
#include <cstdio>
int main() {
const char *text = "hello world";
int i = 0;
for ( const char *p = text; *p; ++p ) ++i;
std::printf("Char num of <%s> = %d\n", text, i);
}
另外,最好使用標准C ++流運算符代替C函數printf。
例如
#include <iostream>
int main() {
const char *text = "hello world";
size_t i = 0;
for ( const char *p = text; *p; ++p ) ++i;
std::cout << "Char num of <" << text << "> = " << i << std::endl;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.