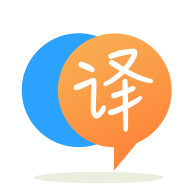
[英]retrieve multiple values from sqlite database and populate it in listview in android
[英]Android Retrieve Values from SQLite Database
我是Android和SQLite數據庫的新手。 我正在完成一個教程,但是在適應我的應用程序時遇到了麻煩。
我想為每個級別建立一個高分數據庫。 因此,我的表將被稱為HighScore,字段ID,Level和HighScore。 Level和HighScore是整數。
這是我的課程:
public class HighScore { private int id; private Integer Level; private Integer highscore; public HighScore(){} public HighScore(Integer Level, Integer highscore) { super(); this.Level = Level; this.highscore = highscore; } //getters & setters public Integer getHighScore() { return highscore; } public Integer getLevel() { return Level; } public int getId() { return id; } public void setId(int id) { this.id = id; } public void setLevel(Integer Level) { this.Level = Level; } public void setHighScore(Integer highscore) { this.highscore = highscore; } }
import java.util.LinkedList; import java.util.List; import android.content.ContentValues; import android.content.Context; import android.database.Cursor; import android.database.sqlite.SQLiteDatabase; import android.database.sqlite.SQLiteOpenHelper; import android.util.Log; public class MySQLiteHelper extends SQLiteOpenHelper { // Database Version private static final int DATABASE_VERSION = 1; // Database Name private static final String DATABASE_NAME = "HighScore"; public MySQLiteHelper(Context context) { super(context, DATABASE_NAME, null, DATABASE_VERSION); } @Override public void onCreate(SQLiteDatabase db) { // SQL statement to create HighScores table String CREATE_HighScore_TABLE = "CREATE TABLE HighScores ( " + "id INTEGER PRIMARY KEY AUTOINCREMENT, " + "Level INTEGER, "+ "HIGHSCORE INTEGER )"; // create HighScores table db.execSQL(CREATE_HighScore_TABLE); } @Override public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) { // Drop older HighScores table if existed db.execSQL("DROP TABLE IF EXISTS HighScores"); // create fresh books table this.onCreate(db); } //--------------------------------------------------------------------- /** * CRUD operations (create "add", read "get", update, delete) book + get all books + delete all books */ // HighScores table name private static final String TABLE_HIGHSCORES = "HighScores"; // HighScores Table Columns names private static final String KEY_ID = "id"; private static final String KEY_LEVEL = "Level"; private static final String KEY_HIGHSCORE = "HIGHSCORE"; private static final String[] COLUMNS = {KEY_ID,KEY_LEVEL,KEY_HIGHSCORE}; public void addHighScore(HighScore highScore){ Log.d("addHighScore", highScore.toString()); // 1. get reference to writable DB SQLiteDatabase db = this.getWritableDatabase(); // 2. create ContentValues to add key "column"/value ContentValues values = new ContentValues(); values.put(KEY_LEVEL, highScore.getLevel()); // get title values.put(KEY_HIGHSCORE, highScore.getHighScore()); // get author // 3. insert db.insert(TABLE_HIGHSCORES, // table null, //nullColumnHack values); // key/value -> keys = column names/ values = column values // 4. close db.close(); } public HighScore getHighScore(Integer Level){ // 1. get reference to readable DB SQLiteDatabase db = this.getReadableDatabase(); // 2. build query Cursor cursor = db.query(TABLE_HIGHSCORES, // a. table COLUMNS, // b. column names " Level = ?", // c. selections new String[] { String.valueOf(Level) }, // d. selections args null, // e. group by null, // f. having null, // g. order by null); // h. limit // 3. if we got results get the first one if (cursor != null) cursor.moveToFirst(); // 4. build highScore object HighScore highScore = new HighScore(); //highScore.setId(Integer.parseInt(cursor.getString(0))); //highScore.setLevel(cursor.getInt(1)); highScore.setHighScore(cursor.getInt(2)); Log.d("getHighScore("+Level+")", highScore.toString()); // 5. return highScore return highScore; } // Get All HighScores public List<HighScore> getAllHighScores() { List<HighScore> highScores = new LinkedList<HighScore>(); // 1. build the query String query = "SELECT * FROM " + TABLE_HIGHSCORES; // 2. get reference to writable DB SQLiteDatabase db = this.getWritableDatabase(); Cursor cursor = db.rawQuery(query, null); // 3. go over each row, build highScore and add it to list HighScore highScore = null; if (cursor.moveToFirst()) { do { highScore = new HighScore(); highScore.setId(Integer.parseInt(cursor.getString(0))); highScore.setLevel(cursor.getInt(1)); highScore.setHighScore(cursor.getInt(2)); // Add highScore to highScores highScores.add(highScore); } while (cursor.moveToNext()); } Log.d("getAllHighScores()", highScores.toString()); // return highScores return highScores; } // Updating single highScore public int updateHighScore(HighScore highScore) { // 1. get reference to writable DB SQLiteDatabase db = this.getWritableDatabase(); // 2. create ContentValues to add key "column"/value ContentValues values = new ContentValues(); values.put("Level", highScore.getLevel()); // get title values.put("HighScore", highScore.getHighScore()); // get author // 3. updating row int i = db.update(TABLE_HIGHSCORES, //table values, // column/value KEY_ID+" = ?", // selections new String[] { String.valueOf(highScore.getId()) }); //selection args // 4. close db.close(); return i; } // Deleting single highScore public void deleteHighScore(HighScore highScore) { // 1. get reference to writable DB SQLiteDatabase db = this.getWritableDatabase(); // 2. delete db.delete(TABLE_HIGHSCORES, KEY_ID+" = ?", new String[] { String.valueOf(highScore.getId()) }); // 3. close db.close(); Log.d("deleteHighScore", highScore.toString()); } }
然后在我的主類的SetStartupValues中,我有以下代碼:
MySQLiteHelper db = new MySQLiteHelper(this); intHighScore = db.getHighScore(intLevel);
我想做的是當SetStartUpValues方法運行時,我可以從表中為用戶intLevel獲得高分。 但是intHighScore是一個整數,並且getHighScore方法返回一個HighScore對象。 如何從HighScore對象獲取1個字段值?
只需將您的getHighScore更改為以下內容
public int getHighScore(Integer Level){
// 1. get reference to readable DB
SQLiteDatabase db = this.getReadableDatabase();
// 2. build query
Cursor cursor =
db.query(TABLE_HIGHSCORES, // a. table
COLUMNS, // b. column names
" Level = ?", // c. selections
new String[] { String.valueOf(Level) }, // d. selections args
null, // e. group by
null, // f. having
null, // g. order by
null); // h. limit
// 3. if we got results get the first one
if (cursor != null)
cursor.moveToFirst();
// 4. return highScore
return cursor.getInt(2);
}
而不是創建一個的HighScore
對象,只返回Integer
的價值HighScore
。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.