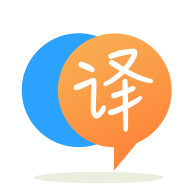
[英]How can I print out words from a list that contains a particular letter? I know what to do with words starting with specific letter but not in BETWEEN
[英]how to print words excluding particular letter string?
我需要編寫一個程序來提示用戶輸入一串禁止的字母,然后打印不包含任何字母的單詞數
這就是我所擁有的:
fin=open("demo.txt",'r')
letters=str(raw_input("Enter the key you want to exclude"))
def avoid ():
for line in fin:
word = line.strip()
for l in letters:
if l not in word:
print word
avoid()
如果我使用in關鍵字,它將打印具有特定字符串的所有單詞。 但是當我使用not in時,它的工作方式不同。
這樣的事情應該起作用:
for l in letters:
if l in word:
break # we found the letter in the word, so stop looping
else:
# This will execute only when the loop above didn't find
# any of the letters.
print(word)
最簡單的方法是將字母組合在一起,將每一行拆分成單詞,然后求和多少次沒有禁止字母的單詞:
with open("demo.txt", 'r') as f:
letters = set(raw_input("Enter the key you want to exclude"))
print(sum(not letters.intersection(w) for line in f for w in line.split()))
您還可以使用str.translate ,檢查翻譯后字長是否已更改:
with open("demo.txt", 'r') as f:
letters = raw_input("Enter the key you want to exclude")
print(sum(len(w.translate(None, letters)) == len(w) for line in f for w in line.split()))
如果嘗試刪除任何letters
后該單詞的長度相同,則該單詞不包含字母中的任何字母。
或使用any
:
with open("in.txt", 'r') as f:
letters = raw_input("Enter the key you want to exclude")
print(sum(not any(let in w for let in letters) for line in f for w in line.split()))
any(let in w for let in letters)
將檢查字母中的每個字母,看每個單詞中是否有字母,如果找到一個禁止的字母,它將短路並返回True
,否則,如果單詞中沒有字母,則返回False
然后繼續下一個詞。
除非每行只有一個單詞,否則您不能使用if l in word
,您需要將其拆分為單個單詞。
使用您自己的代碼,當您在單詞中找到字母時,您只需要中斷即可;否則,如果我們遍歷所有字母但未找到匹配項,請打印該單詞:
for line in fin:
word = line.strip()
for l in letters:
if l in word:
break
else:
print word
在循環中執行所需操作的pythonic方法是使用any:
for line in fin:
word = line.strip()
if not any(l not in word for l in letters):
print word
這等效於break / else好得多。
如果您想要總和,則需要隨時進行跟蹤:
total = 0
for line in fin:
word = line.strip()
if not any(l not in word for l in letters):
total += 1
print(total)
這是一種效率較低的方法:
print(sum(not any(let in w.rstrip() for let in letters) for word in f))
只是另一種方式。
exclude = set(raw_input("Enter the key you want to exclude"))
with open("demo.txt") as f:
print len(filter(exclude.isdisjoint, f.read().split()))
這個更好:
forbidden='ab'
listofstring=['a','ab','acc','aaabb']
for i in listofstring:
if not any((c in i) for c in forbidden):
print i
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.