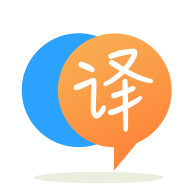
[英]How to display toolbar icons inside an inflated view toolbar android java
[英]Android toolbar doesn't display icons and titles
我正在使用工具欄制作自定義操作欄。 我有一個稱為BaseActivity的類,該類在所有其他活動中繼承。 它將工具欄合並到動作欄。 但問題是我可能看不到工具欄圖標和標題。
這是我的BaseActivity:
package com.meroanswer.classes;
import android.os.Build;
import android.support.annotation.Nullable;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.support.v7.widget.Toolbar;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.ViewGroup;
import android.view.Window;
import android.view.WindowManager;
import com.meroanswer.R;
public abstract class BaseActivity extends ActionBarActivity {
private Toolbar toolbar;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(getLayoutResource());
toolbar = (Toolbar) findViewById(R.id.toolbar);
if (toolbar != null) {
setSupportActionBar(toolbar);
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setHomeButtonEnabled(true);
getSupportActionBar().setDisplayShowHomeEnabled(true);
getSupportActionBar().setDisplayShowTitleEnabled(true);
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) {
Window window = getWindow();
window.addFlags(WindowManager.LayoutParams.FLAG_DRAWS_SYSTEM_BAR_BACKGROUNDS);
window.clearFlags(WindowManager.LayoutParams.FLAG_TRANSLUCENT_STATUS);
window.setStatusBarColor(getResources().getColor(R.color.primary_dark));
}
}
}
protected abstract int getLayoutResource();
protected void setActionBarIcon(int iconRes) {
try{
toolbar.setNavigationIcon(iconRes);
}
catch (NullPointerException e){
Log.d("Roshan", "Exception");
}
}
}
這是我的派生頁面代碼
package com.meroanswer;
import android.content.Context;
import android.content.Intent;
import android.net.Uri;
import android.os.Environment;
import android.support.v4.view.ViewPager;
import android.support.v4.widget.DrawerLayout;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.support.v7.app.ActionBarDrawerToggle;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.support.v7.widget.Toolbar;
import android.view.Gravity;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.webkit.JavascriptInterface;
import android.webkit.WebSettings;
import android.webkit.WebView;
import android.widget.LinearLayout;
import android.widget.Toast;
import com.meroanswer.DrawerAdapter;
import com.meroanswer.MainActivity;
import com.meroanswer.R;
import com.meroanswer.SlidingTabLayout;
import com.meroanswer.ViewPagerAdapter;
import com.meroanswer.classes.BaseActivity;
import com.meroanswer.classes.Helper;
import com.meroanswer.connection.AsyncPut;
import com.meroanswer.connection.Connection;
import com.meroanswer.database.model.Stream;
import com.meroanswer.database.model.User;
import org.apache.http.message.BasicNameValuePair;
import org.json.JSONArray;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class SettingsPage extends BaseActivity {
public WebView webview;
Helper helper;
Context context;
private String temp_str;
private DrawerLayout drawer;
Toolbar toolbar;
RecyclerView mRecyclerView;
RecyclerView.Adapter mAdapter;
RecyclerView.LayoutManager mLayoutManager;
ActionBarDrawerToggle mDrawerToggle;
DrawerLayout Drawer;
String TITLES[] = {
"Coupons",
"Upgrade to Premium",
"Rate us",
"Share App",
"Settings",
"About",
"Log out"
};
int ICONS[] = {
R.drawable.coupon,
R.drawable.premium,
R.drawable.star,
R.drawable.share,
R.drawable.settings,
R.drawable.about,
R.drawable.logout
};
String NAME = "A B";
String EMAIL = "a@b.com";
int PROFILE = R.drawable.profile;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_settings_page);
getSupportActionBar().setIcon(R.drawable.ic_ab_drawer);
getSupportActionBar().setTitle("Settings");
helper = new Helper(context);
webview = (WebView) findViewById(R.id.dashboard_webview);
WebSettings settings = webview.getSettings();
settings.setJavaScriptEnabled(true);
settings.setUseWideViewPort(false);
settings.setLoadsImagesAutomatically(true);
settings.setCacheMode(WebSettings.LOAD_NO_CACHE);
int android_version = android.os.Build.VERSION.SDK_INT;
if (android_version >= 11) {
webview.setLayerType(View.LAYER_TYPE_SOFTWARE, null);
}
webview.addJavascriptInterface(new SettingsProvider(this, webview),
"Android");
webview.loadUrl("file:///android_asset/ma_html/setup.html");
drawer = (DrawerLayout) findViewById(R.id.drawer);
//drawer.setDrawerShadow(R.drawable.drawer_shadow, Gravity.START);
mRecyclerView = (RecyclerView) findViewById(R.id.RecyclerView); // Assigning the RecyclerView Object to the xml View
mRecyclerView.setHasFixedSize(true); // Letting the system know that the list objects are of fixed size
mAdapter = new DrawerAdapter(TITLES,ICONS,NAME,EMAIL,PROFILE); // Creating the Adapter of MyAdapter class(which we are going to see in a bit)
// And passing the titles,icons,header view name, header view email,
// and header view profile picture
mRecyclerView.setAdapter(mAdapter); // Setting the adapter to RecyclerView
mLayoutManager = new LinearLayoutManager(this); // Creating a layout Manager
mRecyclerView.setLayoutManager(mLayoutManager); // Setting the layout Manager
RecyclerView recyclerView = (RecyclerView) findViewById(R.id.RecyclerView);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_settings_page, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case android.R.id.home:
drawer.openDrawer(Gravity.START);
return true;
}
return super.onOptionsItemSelected(item);
}
@Override
protected int getLayoutResource() {
return R.layout.activity_settings_page;
}
}
}
這是我的活動布局資源文件:
<android.support.v4.widget.DrawerLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/drawer"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/white"
android:orientation="vertical">
<include
android:id="@+id/toolbar"
layout="@layout/toolbar"/>
<WebView
android:id="@+id/dashboard_webview"
android:layout_width="fill_parent"
android:layout_height="wrap_content">
</WebView>
</LinearLayout>
<android.support.v7.widget.RecyclerView
android:id="@+id/RecyclerView"
android:layout_width="320dp"
android:layout_height="match_parent"
android:layout_gravity="start"
android:background="#ffffff"
android:scrollbars="vertical">
</android.support.v7.widget.RecyclerView>
<!--<ListView
android:layout_width="260dp"
android:layout_height="match_parent"
android:layout_gravity="start"
android:background="@color/windowBackgroundColor"/>-->
</android.support.v4.widget.DrawerLayout>
菜單文件
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
tools:context="com.meroanswer.SettingsPage">
<item android:id="@+id/action_settings"
android:title="@string/action_settings"
android:orderInCategory="100"
app:showAsAction="never" />
</menu>
執行以下操作,檢查是否可以看到更改:
更改menu.xml,如下所示:
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
tools:context="com.meroanswer.SettingsPage">
<item android:id="@+id/action_settings"
android:title="@string/action_settings"
android:orderInCategory="100"
app:showAsAction="always" /> // This line has been changed from never to always
</menu>
在您的onOptionsItemSelected方法中添加以下部分:
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
Toast.makeText(this, "Settings button has been selected", Toast.Length_Long).show();
return true;
}
運行這些應用程序並單擊設置文本離子工具欄后,您將看到一條祝酒消息。
希望能幫助到你。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.