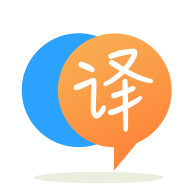
[英]Java error: unreported exception ioexception must be caught or declared to be thrown
[英]Java Error unreported exception IOException; must be caught or declared to be thrown
我正在編寫一個代碼,其中該程序將ping請求發送到另一台計算機。
import java.io.*;
class NetworkPing
{
private static boolean pingIP(String host) throws IOException, InterruptedException
{
boolean isWindows = System.getProperty("os.name").toLowerCase().contains("win");
ProcessBuilder processBuilder = new ProcessBuilder("ping", isWindows? "-n" : "-c", "1", host);
Process proc = processBuilder.start();
int returnVal = proc.waitFor();
return returnVal == 0;
}
public static void main(String args[])
{
pingIP("127.0.0.1");
}
}
在這段代碼中,我收到此錯誤
error: unreported exception IOException; must be caught or declared to be thrown
pingIP("127.0.0.1");
這行顯示錯誤
pingIP("127.0.0.1");
即使在pingIP函數中引發異常,代碼也有什么問題?
您的pingIp
函數會引發exception ,因此,在main中調用它時,您要么必須在那里處理異常 ,要么從main中引發異常 。 在Java中,不能有unhandled exceptions
。 所以你可以這樣:
public static void main(String args[]) throws IOException, InterruptedException
{
pingIP("127.0.0.1");
}
或這個 :
public static void main(String args[])
{
try{
pingIP("127.0.0.1");
} catch(IOException ex){
//TODO handle exception
} catch(InterruptedException ex){
//TODO handle exception
}
}
使用try-catch
塊來處理異常:
try{
pingIP("127.0.0.1");
} catch(IOException e){
e.printStackTrace();
}
或使用throws
public static void main(String args[]) throws IOException{
pingIP("127.0.0.1");
}
try{
pingIP("127.0.0.1");
} catch(IOException e){
e.printStackTrace();
}
or make it throws in public static void main(String args[])throws IOException.
刪除pingIP方法的拋出並將代碼放入try catch塊中
試試下面的代碼
class NetworkPing
{
private static boolean pingIP(String host)
{
Boolean b = false;
try{
boolean isWindows = System.getProperty("os.name").toLowerCase().contains("win");
ProcessBuilder processBuilder = new ProcessBuilder("ping", isWindows? "-n" : "-c", "1", host);
Process proc = processBuilder.start();
int returnVal = proc.waitFor();
b = (returnVal == 0)
}
catch(IOException e){}
catch(InterruptedException e){}
catch(Exception e){}
return b;
}
public static void main(String args[])
{
pingIP("127.0.0.1");
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.