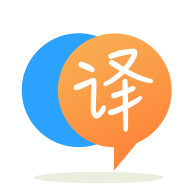
[英]Find minimum depth of binary tree solution does not work when one side of tree is null
[英]How does this code work to find the Minimum Depth of Binary Tree?
我從https://leetcode.com/discuss/37282/simple-python-recursive-solution-bfs-on-80ms看到此代碼
這是答案
給定二叉樹,找到其最小深度。
最小深度是沿距根最短路徑的節點數
節點到最近的葉節點。
class Solution:
# @param {TreeNode} root
# @return {integer}
def minDepth(self, root):
if not root:
return 0
nodes = [(root, 1)]
while nodes:
node, curDepth = nodes.pop(0)
if node.left is None and node.right is None:
return curDepth
if node.left:
nodes.append((node.left, curDepth + 1))
if node.right:
nodes.append((node.right, curDepth + 1))
所以我的困惑是,假設節點1具有節點2和節點3作為其.left和.right子代,那么堆棧將是[(node 2,someDepth),(node 3 someDepth)]。 然后,由於堆棧僅彈出列表的最后一個元素,因此(節點3 someDepth)將被解壓縮,而節點2被完全忽略。 因此,在節點2沒有子節點而節點3有子節點的情況下,使用節點3進行后續迭代是否不對呢?
你所缺少的是
nodes.pop(0)
彈出第0個元素。
因此,您在這里錯了:
然后,因為堆棧只會彈出列表的最后一個元素,所以...
想象一棵二叉樹:
1
/ \
2 3
/ \ / \
4 5 6 7
/ \ / \ /
8 9 10 11 12
這里的狀態空間將更改為(為簡單起見,節點被命名為其內容編號):
# Before 1st iteration.
nodes = [(1, 1)]
# 1st iteration.
node, curDepth = 1, 1
nodes = [(2, 2), (3, 2)]
# 2nd iteration.
node, curDepth = 2, 2
nodes = [(3, 2), (4, 3), (5, 3)]
# 3rd iteration.
node, curDepth = 3, 2
nodes = [(4, 3), (5, 3), (6, 3), (7, 3)]
# 4th iteration.
node, curDepth = 4, 3
nodes = [(5, 3), (6, 3), (7, 3), (8, 4), (9, 4)]
# 5th iteration.
node, curDepth = 5, 3
# Here if node.left is None and node.right is None becomes True and curDepth i.e. 3 is returned.
可以看出,對節點進行了(樹的)廣度處理,因此它是一個BFS。
遞歸解決方案更容易理解。
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution:
# @param {TreeNode} root
# @return {integer}
def minDepth(self, root):
if root is None:
return 0
if root.left is None:
return 1 + self.minDepth(root.right)
if root.right is None:
return 1 + self.minDepth(root.left)
return 1 + min(self.minDepth(root.left), self.minDepth(root.right))
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.