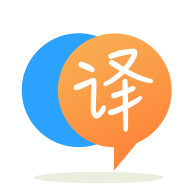
[英]How can I send a string from C++ winsock to Android via TCP socket?
[英]Send a string with sockets in C++ (Winsock TCP/IP)
我想從客戶端向服務器發送帶有套接字的字符串。
這是客戶端的代碼:
#include <iostream>
#include <winsock.h>
#include <unistd.h>
using namespace std;
int main()
{
//Load socket
WSADATA wsaData;
WSAStartup(0x0202, &wsaData);
//Create first socket
int thisSocket;
struct sockaddr_in destination;
destination.sin_family = AF_INET;
thisSocket = socket(AF_INET, SOCK_STREAM, IPPROTO_TCP);
if (thisSocket < 0)
{
cout << "Socket Creation FAILED!" << endl;
return 0;
}
//Connect to a host
destination.sin_port = htons(13374);
destination.sin_addr.s_addr = inet_addr("127.0.0.1");
if (connect(thisSocket,(struct sockaddr *)&destination,sizeof(destination))!=0){
cout << "Socket Connection FAILED!" << endl;
if (thisSocket) close(thisSocket);
return 0;
}
cout << "Connected!" << endl;
//Send the socket
//char *buffer = "Hello, this is a socket!";
string buffer = "Hello, this is a socket!";
//send(thisSocket, buffer, (int)strlen(buffer), 0);
send(thisSocket, buffer.c_str(), buffer.length(), 0);
//Close the socket
closesocket(thisSocket);
WSACleanup();
}
這是服務器的代碼:
#include <iostream>
#include <winsock.h>
#include <unistd.h>
using namespace std;
int main()
{
//Load the socket
WSADATA wsaData;
WSAStartup(0x0202, &wsaData);
//Create the first socket
int thisSocket;
struct sockaddr_in destination;
destination.sin_family = AF_INET;
thisSocket = socket(AF_INET, SOCK_STREAM, IPPROTO_TCP);
if (thisSocket < 0)
{
cout << "Socket Creation FAILED!" << endl;
return 0;
}
//Bind to a socket
destination.sin_port = htons (13374);
destination.sin_addr.s_addr = INADDR_ANY;
if (bind(thisSocket, (struct sockaddr *)&destination, sizeof(destination)) <0){
cout << "Binding Socket FAILED!" << endl;
if (thisSocket) close(thisSocket);
return 0;
}
//Listen on a socket
cout << "Listening on 13374..." << endl;
if (listen(thisSocket, 5)<0){
cout << "Listening on Socket FAILED!" << endl;
if (thisSocket) close(thisSocket);
return 0;
}
//Accept a connection
struct sockaddr_in clientAddress;
int clientSize = sizeof(clientAddress);
thisSocket= accept(thisSocket, (struct sockaddr *)&clientAddress, (int *) &clientSize);
if (thisSocket<0)
{
cout << "Socket Connection FAILED!" << endl;
if (thisSocket) close(thisSocket);
return 0;
}
cout <<"Connection Established!" << endl;
//Receive the socket
char buffer[512];
int newData;
newData = recv(thisSocket, buffer, 512, 0);
cout << newData << endl;
//Close the socket
closesocket(thisSocket);
WSACleanup();
}
如您所見,服務器將收到數字“ 24”。 我怎樣才能得到真正的字符串呢?
recv
讀取的數據最終存儲在buffer
。 recv
函數返回接收到的字節數,如果連接已關閉則為零,如果發生錯誤則為負值。
閱讀recv
參考資料將告訴您所有這些以及更多內容。
請注意,數據不會像字符串一樣終止。 要么像字符串一樣終止它
buffer[newData] = '\0';
在檢查recv
函數實際上收到了一些東西之后。 或者,您可以直接構造一個std::string
對象:
std::string receivedString{buffer, newData};
除非recv
函數實際上收到了一些東西,否則也不要這樣做。
代替:
cout << newData << endl;
做:
cout << buffer << endl;
recv函數返回讀取的字節數,緩沖區保存讀取的字節。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.