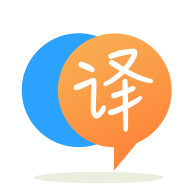
[英]Solve Dining Philosophers Problem Using pthreads, mutex locks, and condition variables
[英]No prints from the second thread - pthreads with mutex locks
我寫:
#include <pthread.h>
#include <stdio.h>
#include <sys/types.h>
pthread_mutex_t mutex1 = PTHREAD_MUTEX_INITIALIZER;
int globalVarX;
void *threadFunction (void *arg)
{
pthread_mutex_lock (&mutex1);
while (globalVarX < 1000)
{
printf("x increment by thread id: %d", gettid ());
++globalVarX;
}
pthread_mutex_unlock (&mutex1);
return NULL;
}
int main()
{
pthread_t threadA;
pthread_t threadB;
if (pthread_create (&threadA, NULL, threadFunction, NULL))
{
fprintf (stderr, "Error creating thread\n");
return 1;
}
if (pthread_create (&threadB, NULL, threadFunction, NULL))
{
fprintf (stderr, "Error creating thread\n");
return 1;
}
if (pthread_join (threadA, NULL))
{
fprintf (stderr, "Error joining thread\n");
return 2;
}
if (pthread_join (threadB, NULL))
{
fprintf (stderr, "Error joining thread\n");
return 2;
}
return 0;
}
我得到的打印如下:
~/studies$ ./a.out
x increment by thread id: 3154
x increment by thread id: 3154
x increment by thread id: 3154
x increment by thread id: 3154
x increment by thread id: 3154
x increment by thread id: 3154
x increment by thread id: 3154
x increment by thread id: 3154
x increment by thread id: 3154
x increment by thread id: 3154
~/studies$
未顯示來自其他線程的打印。
第一個線程將globalVarX
遞增到1000
,第二個線程無關。
我建議:
鎖定一個增量而不是整個循環。
也可以通過調用sched_yield()
來給另一個線程增加增量的機會,因為如果一個線程在其時間片中將globalVarX
增加到1000
,則第二個線程仍然沒有打印輸出。
#include <pthread.h>
#include <stdio.h>
#include <sys/types.h>
#include <sys/syscall.h>
#include <unistd.h>
pthread_mutex_t mutex1 = PTHREAD_MUTEX_INITIALIZER;
int globalVarX;
void *threadFunction (void *arg)
{
int flagbreak = 0;
for(;!flagbreak;) {
pthread_mutex_lock (&mutex1);
if (globalVarX >= 1000) flagbreak = 1;
else {
++globalVarX;
printf("x increment by thread id: %ld\n", syscall(SYS_gettid));
}
pthread_mutex_unlock (&mutex1);
sched_yield();
}
return NULL;
}
int main(void)
{
pthread_t threadA;
pthread_t threadB;
if (pthread_create (&threadA, NULL, threadFunction, NULL))
{
fprintf (stderr, "Error creating thread\n");
return 1;
}
if (pthread_create (&threadB, NULL, threadFunction, NULL))
{
fprintf (stderr, "Error creating thread\n");
return 1;
}
if (pthread_join (threadA, NULL))
{
fprintf (stderr, "Error joining thread\n");
return 2;
}
if (pthread_join (threadB, NULL))
{
fprintf (stderr, "Error joining thread\n");
return 2;
}
return 0;
}
要從概念上給兩個線程一個增加計數器的機會,請使用以下命令:
偽代碼:
Condition cond = condition_init;
Mutex mutex = mutex_init;
int counter = 0;
thread_func
{
mutex_lock(mutex);
while (counter < 1000)
{
++counter;
print thread, counter;
condition_signal(cond);
condition_wait(cond, mutex);
}
condition_signal(cond);
mutex_unlock(mutex);
}
main
{
Thread thread_a = thread_create(thread_func);
Thread thread_b = treadd_create(thread_func);
thread_join(thread_a);
thread_join(thread_b);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.