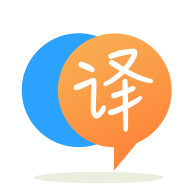
[英]How to pass the data from listview of one activity and pass it to another activity?
[英]Pass data from a listview to a new activity
有人可以幫我解決以下問題:
當我單擊listview
的項目時,我需要使用listview
的數據打開一個新活動...
這是我的代碼:
public class listar_clientes extends ListActivity {
private ProgressDialog pDialog;
// Creating JSON Parser object
JSONParser jParser = new JSONParser();
ArrayList<HashMap<String, String>> productsList;
// url to get all products list
private static String url_all_products = "http://192.168.137.100:81/androidapp/listar_clientes.php";
// JSON Node names
private static final String TAG_CLIENTE= "clientes";
private static final String TAG_NOME = "nome";
private static final String TAG_ABV = "nome2";
// products JSONArray
JSONArray products = null;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_listar_clientes);
// Hashmap for ListView
productsList = new ArrayList<HashMap<String, String>>();
// Loading products in Background Thread
new LoadAllProducts().execute();
// Log.v("COUNT-->",productsList.get(0).toString());
// Get listview
ListView list = getListView();
// launching Edit Product Screen
// lv.setOnItemClickListener(new AdapterView.OnItemClickListener() {
//
//
// // Starting new intent
// Intent in = new Intent(getApplicationContext(),
// list_item.class);
// // sending pid to next activity
// in.putExtra(TAG_PID, pid);
//
// // starting new activity and expecting some response back
// startActivityForResult(in, 100);
// }
// });
/* ListAdapter adapter = new SimpleAdapter(
encomendas_user.this, productsList,
R.layout.activity_list_item, new String[] { TAG_IDUSER,
TAG_TITULO},
new int[] { R.id.pid, R.id.name });
// updating listview
setListAdapter(adapter);*/
// on seleting single product
// launching Edit Product Screen
}
// Response from Edit Product Activity
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
// if result code 100
if (resultCode == 100) {
// if result code 100 is received
// means user edited/deleted product
// reload this screen again
Intent intent = getIntent();
finish();
startActivity(intent);
}
}
/**
* Background Async Task to Load all product by making HTTP Request
* */
class LoadAllProducts extends AsyncTask<String, String, String> {
/**
* Before starting background thread Show Progress Dialog
* */
@Override
protected void onPreExecute() {
super.onPreExecute();
pDialog = new ProgressDialog(listar_clientes.this);
pDialog.setMessage("A Carregar dados. Por favor Aguarde...");
pDialog.setIndeterminate(false);
pDialog.setCancelable(false);
pDialog.show();
}
/**
* getting All products from url
* */
protected String doInBackground(String... args) {
// Building Parameters
List<NameValuePair> params = new ArrayList<NameValuePair>();
// getting JSON string from URL
JSONObject json = jParser.makeHttpRequest(url_all_products, "GET", params);
// Check your log cat for JSON reponse
Log.d("All Products: ", json.toString());
try {
// Checking for SUCCESS TAG
// int success = json.getInt(TAG_IDUSER);
//Log.d("NAMSUCCESSE: ", ""+success);
//if (success == 1) {
// products found
// Getting Array of Products
products = json.getJSONArray(TAG_CLIENTE);
// looping through All Products
for (int i = 0; i < products.length(); i++) {
JSONObject c = products.getJSONObject(i);
// Storing each json item in variable
String name = c.getString(TAG_NOME);
String abv = c.getString(TAG_ABV);
Log.d("NAME TESTE: ", name);
// creating new HashMap
HashMap<String, String> map = new HashMap<String, String>();
// adding each child node to HashMap key => value
map.put(TAG_NOME, "Nome:"+" "+ name);
map.put(TAG_ABV, "Abreviatura:"+" "+abv);
// if(UserInfo.userID==(Integer.parseInt(name))){
// adding HashList to ArrayList
productsList.add(map);
// }
}
/* } else {
// no products found
// Launch Add New product Activity
//Intent i = new Intent(getApplicationContext(),
// NewProductActivity.class);
// Closing all previous activities
//i.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP);
// startActivity(i);
}*/
} catch (JSONException e) {
e.printStackTrace();
}
return null;
}
/**
* After completing background task Dismiss the progress dialog
* **/
protected void onPostExecute(String file_url) {
// dismiss the dialog after getting all products
pDialog.dismiss();
// updating UI from Background Thread
runOnUiThread(new Runnable() {
public void run() {
/**
* Updating parsed JSON data into ListView
* */
ListAdapter adapter = new SimpleAdapter(
listar_clientes.this, productsList,
R.layout.activity_list_item_clientes, new String[] { TAG_NOME, TAG_ABV},
new int[] { R.id.name, R.id.abv});
// updating listview
setListAdapter(adapter);
}
});
}
/*private AdapterView.OnItemClickListener OnListClick=new AdapterView.OnItemClickListener(){
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent i = new Intent(listar_clientes.this, detalhes_cliente.class);
in.putExtra(TAG_NOME, id_user.getText().toString());
startActivity(i);
}
}*/
public void detalhes (View v){
Intent i = new Intent(listar_clientes.this, detalhes_cliente.class);
}
}
}
這是xml代碼:
<?xml version="1.0" encoding="utf-8"?>
<ScrollView
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/scroll"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:fillViewport="false">
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:paddingBottom="5dp"
android:longClickable="true"
android:padding="10dp"
android:onClick="detalhes"
>
<!-- Product id (pid) - will be HIDDEN - used to pass to other activity -->
<TextView
android:id="@+id/pid"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:visibility="gone"
android:paddingBottom="5dp"/>
<!-- Name Label -->
<TextView
android:id="@+id/name"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:paddingTop="6dip"
android:paddingLeft="6dip"
android:textSize="17dip"
android:textStyle="bold"
android:textColor="#ff014cff"
android:text="Nome Cliente"
android:paddingBottom="5dp"/>
<TextView
android:id="@+id/abv"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/name"
android:paddingTop="6dip"
android:paddingLeft="6dip"
android:textSize="17dip"
android:textStyle="bold"
android:textColor="#ff000000"
android:text="Abreviatura" />
</RelativeLayout>
</ScrollView>
只需根據要發送的數據更改您的方法。
public void detalhes (View v){
Intent i = new Intent(listar_clientes.this, detalhes_cliente.class);
Bundle mBundle = new Bundle();
mBundle.putString(key1, value1);
mBundle.putInt(key2,value2);
mBundle.putStringArray(key3, value3);
mBundle.putStringArrayList(key4, value4);
..
....
mBundle.putInt(key, value);
i.putExtras(mBundle);
startActivity(i);
}
作為參考,您可以使用此鏈接來了解如何從捆綁軟件中取回數據,並且更加清楚。 可能會有所幫助..謝謝
將數據從ListView的 onItemClick 傳遞到其他Activity
初始化變量:
private ListView listView;
綁定視圖:
listView = (ListView)findViewById(R.id.listView);
ListView的onItemClick:
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent intent = new Intent(this, Your_New_Activity.class);
intent.putExtra("key 1", "Value 1");
intent.putExtra("key 2", "Value 2");
intent.putExtra("key 3", "Value 3");
startActivity(intent);
}
});
現在,在新活動的onCreate上接收數據:
String key_one = getIntent().getStringExtra("key 1");
String key_two = getIntent().getStringExtra("key 2");
String key_three = getIntent().getStringExtra("key 3");
完成
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.