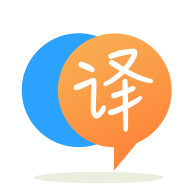
[英]How to let other app access my app's data like Google Drive app do
[英]Access Google drive data in my app
我需要訪問我的應用程序上的一個共享文件夾,這是在Google驅動器“ drive.google.com”上創建的共享文件夾。 這將包括我應用程序中的所有子文件和文件夾。 我嘗試使用Google Drive API訪問數據,但未顯示驅動器上的任何數據。 我已使用此鏈接訪問該文件夾。
請幫我解決這個問題。 請提供有效的參考鏈接給我。
盡管沒有明確記錄,但可以將Android應用程序連接到公共Drive文件夾。
此處描述了前三個步驟。 在那里描述了第四步。 您可能需要Drive REST API文檔。
我做了一個片段。 我只是在這里保留了基本的東西。 我正在請求公用文件夾的文件列表。
import android.app.ProgressDialog;
import android.content.Context;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.os.AsyncTask;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v7.app.ActionBar;
import android.support.v7.widget.RecyclerView;
import android.text.TextUtils;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Toast;
import com.google.api.client.googleapis.auth.oauth2.GoogleCredential;
import com.google.api.client.http.HttpTransport;
import com.google.api.client.json.JsonFactory;
import com.google.api.client.extensions.android.http.AndroidHttp;
import com.google.api.client.json.jackson2.JacksonFactory;
import com.google.api.services.drive.DriveScopes;
import com.google.api.services.drive.model.File;
import com.google.api.services.drive.model.FileList;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class FragmentDownload extends Fragment {
GoogleCredential aCredential;
ProgressDialog mProgress;
/**
* Required empty public constructor
*/
public FragmentDownload() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_download, container, false);
}
@Override
public void onActivityCreated(Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
mProgress = new ProgressDialog(getContext());
mProgress.setMessage("Calling Drive API ...");
// Initialize credentials object.
try {
aCredential = GoogleCredential
.fromStream(getContext().getResources().openRawResource(R.raw.json_file))
.createScoped(DriveScopes.all());
} catch (Exception e) {
e.printStackTrace();
}
getResultsFromApi();
}
/**
* Checks whether the device currently has a network connection.
* @return true if the device has a network connection, false otherwise.
*/
private boolean isDeviceOnline() {
ConnectivityManager connMgr =
(ConnectivityManager) getActivity().getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = connMgr.getActiveNetworkInfo();
return (networkInfo != null && networkInfo.isConnected());
}
/**
* Attempt to call the API, after verifying that all the preconditions are
* satisfied. The preconditions are: Google Play Services installed, an
* account was selected and the device currently has online access. If any
* of the preconditions are not satisfied, the app will prompt the user as
* appropriate.
*/
private void getResultsFromApi() {
if (! isDeviceOnline()) {
Toast.makeText(getContext(), "No network connection available.", Toast.LENGTH_LONG).show();
} else {
new MakeRequestTask(aCredential).execute();
}
}
/**
* An asynchronous task that handles the Drive API call.
* Placing the API calls in their own task ensures the UI stays responsive.
*/
private class MakeRequestTask extends AsyncTask<Void, Void, List<String>> {
private com.google.api.services.drive.Drive mService = null;
private Exception mLastError = null;
public MakeRequestTask(GoogleCredential credential) {
HttpTransport transport = AndroidHttp.newCompatibleTransport();
JsonFactory jsonFactory = JacksonFactory.getDefaultInstance();
mService = new com.google.api.services.drive.Drive.Builder(
transport, jsonFactory, credential)
.setApplicationName("appName")
.build();
}
/**
* Background task to call Drive API.
* @param params no parameters needed for this task.
*/
@Override
protected List<String> doInBackground(Void... params) {
try {
return getDataFromApi();
} catch (Exception e) {
mLastError = e;
cancel(true);
return null;
}
}
/**
* Fetch a list of up to 10 file names and IDs.
* @return List of Strings describing files, or an empty list if no files
* found.
* @throws IOException
*/
private List<String> getDataFromApi() throws IOException {
// Get a list of up to 10 files.
List<String> fileInfo = new ArrayList<>();
FileList result = mService.files().list()
.setCorpus("user")
.setQ("'public_gdrive_folder_id' in parents")
.setOrderBy("name")
.setSpaces("drive")
.setFields("files(id, name)")
.execute();
List<File> files = result.getFiles();
if (files != null) {
for (File file : files) {
fileInfo.add(String.format("%s (%s)\n",
file.getName(), file.getId()));
}
}
return fileInfo;
}
@Override
protected void onPreExecute() {
mProgress.show();
}
@Override
protected void onPostExecute(List<String> output) {
mProgress.hide();
if (output == null || output.size() == 0) {
Toast.makeText(getContext(), "No results returned.", Toast.LENGTH_LONG).show();
} else {
//output.add(0, "Data retrieved using the Drive API:");
Toast.makeText(getContext(), TextUtils.join("\n", output), Toast.LENGTH_LONG).show();
}
}
@Override
protected void onCancelled() {
mProgress.hide();
if (mLastError != null) {
Toast.makeText(getContext(), "The following error occurred:\n"
+ mLastError.getMessage(), Toast.LENGTH_LONG).show();
// }
} else {
Toast.makeText(getContext(), "Request cancelled.", Toast.LENGTH_LONG).show();
}
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.