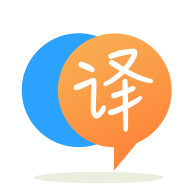
[英]Android - how to parse html by jsoup and fill into the arraylist?
[英]How to fill a form with Jsoup?
我正在嘗試導航到加利福尼亞網站http://kepler.sos.ca.gov/ 的描述頁面。 卻無法去。
然后,我有一個 html 表單,我正在提交請求,我無法在此處添加表單,但它是一個簡單的POST
請求到http://kepler.sos.ca.gov/並帶有所需的參數
我可以從我來到這里的上一頁獲得__EVENTTARGET
和__EVENTARGUMENT
。
我究竟做錯了什么?
代碼:
String url = "kepler.sos.ca.gov/";
Connection.Response resp = Jsoup.connect(url)
.timeout(30000)
.method(Connection.Method.GET)
.execute();
Document responseDocument = resp.parse();
Map<String, String> loginCookies = resp.cookies();
eventValidation=responseDocument.select("input[name=__EVENTVALIDATION]").first();
viewState = responseDocument.select("input[name=__VIEWSTATE]").first();
您想使用FormElement
。 這是 Jsoup 的一個有用功能。 它能夠找到表單中聲明的字段並為您發布它們。 在發布表單之前,您可以使用 Jsoup API 設置字段的值。
注意:
在下面的示例代碼中,您將始終看到對Element#select方法的調用,然后是對Elements#first方法的調用。
例如:
responseDocument.select("form#aspnetForm").first()
Jsoup 1.11.1引入了一個更有效的替代方案: Element#selectFirst 。 您可以將其用作原始替代品的直接替代品。
例如:
responseDocument.select("form#aspnetForm").first()
可以替換為responseDocument.selectFirst("form#aspnetForm")
// * Connect to website
String url = "http://kepler.sos.ca.gov/";
Connection.Response resp = Jsoup.connect(url) //
.timeout(30000) //
.method(Connection.Method.GET) //
.execute();
// * Find the form
Document responseDocument = resp.parse();
Element potentialForm = responseDocument.select("form#aspnetForm").first();
checkElement("form element", potentialForm);
FormElement form = (FormElement) potentialForm;
// * Fill in the form and submit it
// ** Search Type
Element radioButtonListSearchType = form.select("[name$=RadioButtonList_SearchType]").first();
checkElement("search type radio button list", radioButtonListSearchType);
radioButtonListSearchType.attr("checked", "checked");
// ** Name search
Element textBoxNameSearch = form.select("[name$=TextBox_NameSearch]").first();
checkElement("name search text box", textBoxNameSearch);
textBoxNameSearch.val("cali");
// ** Submit the form
Document searchResults = form.submit().cookies(resp.cookies()).post();
// * Extract results (entity numbers in this sample code)
for (Element entityNumber : searchResults.select("table[id$=SearchResults_Corp] > tbody > tr > td:first-of-type:not(td[colspan=5])")) {
System.out.println(entityNumber.text());
}
public static void checkElement(String name, Element elem) {
if (elem == null) {
throw new RuntimeException("Unable to find " + name);
}
}
C3036475
C3027305
C3236514
C3027304
C3034012
C3035110
C3028330
C3035378
C3124793
C3734637
在本例中,我們將使用FormElement類登錄到GitHub網站。
// # Constants used in this example
final String USER_AGENT = "Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/51.0.2704.103 Safari/537.36";
final String LOGIN_FORM_URL = "https://github.com/login";
final String USERNAME = "yourUsername";
final String PASSWORD = "yourPassword";
// # Go to login page
Connection.Response loginFormResponse = Jsoup.connect(LOGIN_FORM_URL)
.method(Connection.Method.GET)
.userAgent(USER_AGENT)
.execute();
// # Fill the login form
// ## Find the form first...
FormElement loginForm = (FormElement)loginFormResponse.parse()
.select("div#login > form").first();
checkElement("Login Form", loginForm);
// ## ... then "type" the username ...
Element loginField = loginForm.select("#login_field").first();
checkElement("Login Field", loginField);
loginField.val(USERNAME);
// ## ... and "type" the password
Element passwordField = loginForm.select("#password").first();
checkElement("Password Field", passwordField);
passwordField.val(PASSWORD);
// # Now send the form for login
Connection.Response loginActionResponse = loginForm.submit()
.cookies(loginFormResponse.cookies())
.userAgent(USER_AGENT)
.execute();
System.out.println(loginActionResponse.parse().html());
public static void checkElement(String name, Element elem) {
if (elem == null) {
throw new RuntimeException("Unable to find " + name);
}
}
所有的表單數據都由 FormElement 類為我們處理(甚至是表單方法檢測)。 調用FormElement#submit方法時會構建一個現成的Connection 。 我們所要做的就是用額外的頭文件(cookies、用戶代理等)完成這個連接並執行它。
這與上面已接受的答案中發布的代碼完全相同,但它反映了加利福尼亞州在發布原始答案后對其網站所做的更改。 所以在我寫這篇文章時,這段代碼有效。 我已經更新了原始評論,確定了任何更改。
// * Connect to website (Orignal url: http://kepler.sos.ca.gov/)
String url = "https://businesssearch.sos.ca.gov/";
Connection.Response resp = Jsoup.connect(url) //
.timeout(30000) //
.method(Connection.Method.GET) //
.execute();
// * Find the form (Original jsoup selector: from#aspnetForm)
Document responseDocument = resp.parse();
Element potentialForm = responseDocument.select("form#formSearch").first();
checkElement("form element", potentialForm);
FormElement form = (FormElement) potentialForm;
// * Fill in the form and submit it
// ** Search Type (Original jsoup selector: name$=RadioButtonList_SearchType)
Element radioButtonListSearchType = form.select("name$=SearchType]").first();
checkElement("search type radio button list", radioButtonListSearchType);
radioButtonListSearchType.attr("checked", "checked");
// ** Name search (Original jsoup selector: name$=TextBox_NameSearch)
Element textBoxNameSearch = form.select("[name$=SearchCriteria]").first();
checkElement("name search text box", textBoxNameSearch);
textBoxNameSearch.val("cali");
// ** Submit the form
Document searchResults = form.submit().cookies(resp.cookies()).post();
// * Extract results (entity numbers in this sample code, orignal jsoup selector: id$=SearchResults_Corp)
for (Element entityNumber : searchResults.select("table[id$=enitityTable] > tbody > tr > td:first-of-type:not(td[colspan=5])")) {
System.out.println(entityNumber.text());
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.