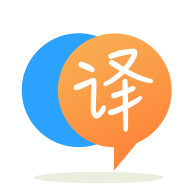
[英]Create Google Script that saves sheet as pdf to specified folder in google drive
[英]Trying to create multiple PDF for each row in a Google Sheet
我正在嘗試使用Google文檔作為模板為Google表格中的每一行創建單獨的PDF。 使用活動行時,它可以正常工作; 但是,當我嘗試循環執行createpdf函數時,剩下的唯一PDF是最后一行。 我的想法是,我要事先刪除所有文件,但無法弄清楚錯誤在哪里。 任何幫助都是極好的。
var TEMPLATE_ID = '1Mxrm0kny8R7ROlBou8kSUc8dOvvH8PvtQ-EC_Nd3FUQ';
function onOpen() {
SpreadsheetApp
.getUi()
.createMenu('Create PDF')
.addItem('Create PDF', 'createPdf')
.addToUi();
}
for (var i=2; i < 3; i++) { function createPdf() {
if (TEMPLATE_ID === '') {
SpreadsheetApp.getUi().alert('TEMPLATE_ID needs to be defined in code.gs');
return;
}
// Set up the docs and the spreadsheet access
var copyFile = DriveApp.getFileById(TEMPLATE_ID).makeCopy(),
copyId = copyFile.getId(),
copyDoc = DocumentApp.openById(copyId),
copyBody = copyDoc.getBody(),
activeSheet = SpreadsheetApp.getActiveSheet(),
numberOfColumns = activeSheet.getLastColumn(),
activeRow = activeSheet.getRange(i, 1, 1, numberOfColumns).getValues(),
headerRow = activeSheet.getRange(1, 1, 1, numberOfColumns).getValues(),
columnIndex = 0,
fileName = activeRow[0][0] + activeRow[0][1],
pdfFile,
pdfFile2;
// Replace the keys with the spreadsheet values
for (;columnIndex < headerRow[0].length; columnIndex++) {
copyBody.replaceText('%' + headerRow[0][columnIndex] + '%',
activeRow[0][columnIndex]);
}
// Create the PDF file and delete the doc copy
copyDoc.saveAndClose();
pdfFile = DriveApp.createFile(copyFile.getAs("application/pdf"));
pdfFile2 = pdfFile.setName(fileName)
copyFile.setTrashed(true);
return pdfFile2;
}}
提示:注意正確格式化代碼,因為它可以幫助您理解其結構。 這是通過jsbeautifier傳遞后的內容 :
var TEMPLATE_ID = '1Mxrm0kny8R7ROlBou8kSUc8dOvvH8PvtQ-EC_Nd3FUQ';
function onOpen() {
SpreadsheetApp
.getUi()
.createMenu('Create PDF')
.addItem('Create PDF', 'createPdf')
.addToUi();
}
for (var i = 2; i < 3; i++) {
function createPdf() {
if (TEMPLATE_ID === '') {
SpreadsheetApp.getUi().alert('TEMPLATE_ID needs to be defined in code.gs');
return;
}
// Set up the docs and the spreadsheet access
var copyFile = DriveApp.getFileById(TEMPLATE_ID).makeCopy(),
copyId = copyFile.getId(),
copyDoc = DocumentApp.openById(copyId),
copyBody = copyDoc.getBody(),
activeSheet = SpreadsheetApp.getActiveSheet(),
numberOfColumns = activeSheet.getLastColumn(),
activeRow = activeSheet.getRange(i, 1, 1, numberOfColumns).getValues(),
headerRow = activeSheet.getRange(1, 1, 1, numberOfColumns).getValues(),
columnIndex = 0,
fileName = activeRow[0][0] + activeRow[0][1],
pdfFile,
pdfFile2;
// Replace the keys with the spreadsheet values
for (; columnIndex < headerRow[0].length; columnIndex++) {
copyBody.replaceText('%' + headerRow[0][columnIndex] + '%',
activeRow[0][columnIndex]);
}
// Create the PDF file and delete the doc copy
copyDoc.saveAndClose();
pdfFile = DriveApp.createFile(copyFile.getAs("application/pdf"));
pdfFile2 = pdfFile.setName(fileName)
copyFile.setTrashed(true);
return pdfFile2;
}
}
縮進澄清了一些問題:
i
上的for
循環在全局范圍內,而不是任何函數的一部分。 因此,它將在每次調用腳本中的ANY函數時執行,但不會像在函數中那樣被顯式調用。
函數createPDF
在for
循環中聲明。 這是全局范圍的(請參見第1點),因此仍然可以從其他函數(例如,由onOpen()
創建的菜單onOpen()
。 但是,因為它只是一個聲明,所以i loop
不會調用它! (這是您的問題的主要關注點。)
在createPdf()
中有一個return
調用,它可能是此代碼的原始副本遺留下來的代碼,該代碼生成了一個PDF。 因為它是第一次在循環中返回,所以仍會生成一個PDF。 該行需要移動或刪除。
return pdfFile2;
翻轉這幾行並刪除return
應該可以解決問題。
var TEMPLATE_ID = '1Mxrm0kny8R7ROlBou8kSUc8dOvvH8PvtQ-EC_Nd3FUQ';
function onOpen() {
SpreadsheetApp
.getUi()
.createMenu('Create PDF')
.addItem('Create PDF', 'createPdf')
.addToUi();
}
function createPdf() {
for (var i = 2; i < 3; i++) {
if (TEMPLATE_ID === '') {
SpreadsheetApp.getUi().alert('TEMPLATE_ID needs to be defined in code.gs');
return;
}
// Set up the docs and the spreadsheet access
var copyFile = DriveApp.getFileById(TEMPLATE_ID).makeCopy(),
copyId = copyFile.getId(),
copyDoc = DocumentApp.openById(copyId),
copyBody = copyDoc.getBody(),
activeSheet = SpreadsheetApp.getActiveSheet(),
numberOfColumns = activeSheet.getLastColumn(),
activeRow = activeSheet.getRange(i, 1, 1, numberOfColumns).getValues(),
headerRow = activeSheet.getRange(1, 1, 1, numberOfColumns).getValues(),
columnIndex = 0,
fileName = activeRow[0][0] + activeRow[0][1],
pdfFile,
pdfFile2;
// Replace the keys with the spreadsheet values
for (; columnIndex < headerRow[0].length; columnIndex++) {
copyBody.replaceText('%' + headerRow[0][columnIndex] + '%',
activeRow[0][columnIndex]);
}
// Create the PDF file and delete the doc copy
copyDoc.saveAndClose();
pdfFile = DriveApp.createFile(copyFile.getAs("application/pdf"));
pdfFile2 = pdfFile.setName(fileName)
copyFile.setTrashed(true);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.