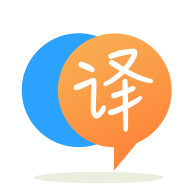
[英]I made a code for dynamically creating new buttons on a homepage from a separate activity but when I click my button it crashes the entire app
[英]My App crashes when i click a button to open new activity with error: NullPointerException
所以我使我的應用程序正常運行並在我的設備上運行(即使它什么也不做),然后我創建了第二個活動和一個切換到該第二個活動的按鈕)這是我的代碼:
AndroidManifest.xml`
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".menu_state"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity
android:name=".activity_game_state"
android:configChanges="orientation|keyboardHidden|screenSize"
android:label="@string/title_activity_activity_game_state"
android:theme="@style/FullscreenTheme" >
<intent-filter>
<action android:name="dk.carlemil.upanddown.activity_game_state" />
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
</activity>
</application>
和我的主要活動名為menu_state
import android.content.Intent;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.ImageButton;
public class menu_state extends ActionBarActivity {
private static ImageButton image_button_sbm;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_menu_state);
onClickImageButtonListener();
}
public void onClickImageButtonListener(){
image_button_sbm = (ImageButton)findViewById(R.id.imageButton2);
image_button_sbm.setOnClickListener(
new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent("dk.carlemil.upanddown.activity_game_state");
startActivity(intent);
}
}
);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_menu_state, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
// i don't know where this should be placed but I'm trying to make it switch to the other screen when the gamemenu button gets clicked
}
我的第二項活動名為activity_game_state
package dk.carlemil.upanddown;
import dk.carlemil.upanddown.util.SystemUiHider;
import android.annotation.TargetApi;
import android.app.Activity;
import android.os.Build;
import android.os.Bundle;
import android.os.Handler;
import android.view.MotionEvent;
import android.view.View;
/**
* An example full-screen activity that shows and hides the system UI (i.e.
* status bar and navigation/system bar) with user interaction.
*
* @see SystemUiHider
*/
public class activity_game_state extends Activity {
/**
* Whether or not the system UI should be auto-hidden after
* {@link #AUTO_HIDE_DELAY_MILLIS} milliseconds.
*/
private static final boolean AUTO_HIDE = true;
/**
* If {@link #AUTO_HIDE} is set, the number of milliseconds to wait after
* user interaction before hiding the system UI.
*/
private static final int AUTO_HIDE_DELAY_MILLIS = 3000;
/**
* If set, will toggle the system UI visibility upon interaction. Otherwise,
* will show the system UI visibility upon interaction.
*/
private static final boolean TOGGLE_ON_CLICK = true;
/**
* The flags to pass to {@link SystemUiHider#getInstance}.
*/
private static final int HIDER_FLAGS = SystemUiHider.FLAG_HIDE_NAVIGATION;
/**
* The instance of the {@link SystemUiHider} for this activity.
*/
private SystemUiHider mSystemUiHider;
/*@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_activity_game_state);
final View controlsView = findViewById(R.id.fullscreen_content_controls);
final View contentView = findViewById(R.id.fullscreen_content);
// Set up an instance of SystemUiHider to control the system UI for
// this activity.
mSystemUiHider = SystemUiHider.getInstance(this, contentView, HIDER_FLAGS);
mSystemUiHider.setup();
mSystemUiHider
.setOnVisibilityChangeListener(new SystemUiHider.OnVisibilityChangeListener() {
// Cached values.
int mControlsHeight;
int mShortAnimTime;
@Override
@TargetApi(Build.VERSION_CODES.HONEYCOMB_MR2)
public void onVisibilityChange(boolean visible) {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.HONEYCOMB_MR2) {
// If the ViewPropertyAnimator API is available
// (Honeycomb MR2 and later), use it to animate the
// in-layout UI controls at the bottom of the
// screen.
if (mControlsHeight == 0) {
mControlsHeight = controlsView.getHeight();
}
if (mShortAnimTime == 0) {
mShortAnimTime = getResources().getInteger(
android.R.integer.config_shortAnimTime);
}
controlsView.animate()
.translationY(visible ? 0 : mControlsHeight)
.setDuration(mShortAnimTime);
} else {
// If the ViewPropertyAnimator APIs aren't
// available, simply show or hide the in-layout UI
// controls.
controlsView.setVisibility(visible ? View.VISIBLE : View.GONE);
}
if (visible && AUTO_HIDE) {
// Schedule a hide().
delayedHide(AUTO_HIDE_DELAY_MILLIS);
}
}
});
// Set up the user interaction to manually show or hide the system UI.
contentView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
if (TOGGLE_ON_CLICK) {
mSystemUiHider.toggle();
} else {
mSystemUiHider.show();
}
}
});
}
*/
@Override
protected void onPostCreate(Bundle savedInstanceState) {
super.onPostCreate(savedInstanceState);
// Trigger the initial hide() shortly after the activity has been
// created, to briefly hint to the user that UI controls
// are available.
delayedHide(100);
}
/**
* Touch listener to use for in-layout UI controls to delay hiding the
* system UI. This is to prevent the jarring behavior of controls going away
* while interacting with activity UI.
*/
View.OnTouchListener mDelayHideTouchListener = new View.OnTouchListener() {
@Override
public boolean onTouch(View view, MotionEvent motionEvent) {
if (AUTO_HIDE) {
delayedHide(AUTO_HIDE_DELAY_MILLIS);
}
return false;
}
};
Handler mHideHandler = new Handler();
Runnable mHideRunnable = new Runnable() {
@Override
public void run() {
mSystemUiHider.hide();
}
};
/**
* Schedules a call to hide() in [delay] milliseconds, canceling any
* previously scheduled calls.
*/
private void delayedHide(int delayMillis) {
mHideHandler.removeCallbacks(mHideRunnable);
mHideHandler.postDelayed(mHideRunnable, delayMillis);
}
}
和我的主要活動(menu_sate).xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".menu_state"
android:screenOrientation="portrait">
<ImageButton
android:layout_width="150sp"
android:layout_height="150sp"
android:id="@+id/imageButton"
android:layout_above="@+id/imageButton2"
android:layout_centerHorizontal="true"
android:layout_marginBottom="50dp"
android:background="@drawable/logo_image"/>
<ImageButton
android:layout_width="wrap_content"
android:layout_height="120sp"
android:id="@+id/imageButton2"
android:background="@drawable/start_image1"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true" />
<ImageButton
android:layout_width="wrap_content"
android:layout_height="120sp"
android:id="@+id/imageButton3"
android:background="@drawable/start_image2"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:layout_marginBottom="30sp"/>
</RelativeLayout>
and my second activity(activity_game_state) .xml file
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" android:background="#ffffffff"
tools:context="dk.carlemil.upanddown.activity_game_state"
android:orientation="horizontal">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="1"
android:id="@+id/button1"
android:background="@drawable/cirkle_button"
android:layout_above="@+id/button4"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="2"
android:id="@+id/button2"
android:background="@drawable/cirkle_button"
android:layout_above="@+id/button5"
android:layout_centerHorizontal="true" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="3"
android:id="@+id/button3"
android:background="@drawable/cirkle_button"
android:layout_above="@+id/button6"
android:layout_alignLeft="@+id/button6"
android:layout_alignStart="@+id/button6" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="4"
android:id="@+id/button4"
android:background="@drawable/cirkle_button"
android:layout_alignParentBottom="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="5"
android:id="@+id/button5"
android:background="@drawable/cirkle_button"
android:layout_alignTop="@+id/button6"
android:layout_centerHorizontal="true" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="6"
android:id="@+id/button6"
android:background="@drawable/cirkle_button"
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true" />
</RelativeLayout>
最后是我認為問題所在的logcat
rocess: dk.carlemil.upanddown, PID: 9017
java.lang.NullPointerException: Attempt to invoke virtual method 'void dk.carlemil.upanddown.util.SystemUiHider.hide()' on a null object reference
at dk.carlemil.upanddown.activity_game_state$2.run(activity_game_state.java:145)
at android.os.Handler.handleCallback(Handler.java:739)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:145)
at android.app.ActivityThread.main(ActivityThread.java:5942)
at java.lang.reflect.Method.invoke(Native Method)
at java.lang.reflect.Method.invoke(Method.java:372)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:1400)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:1195)
07-20 22:14:43.974 871-1210/? E/CSLegacyTypeTracker﹕ add() : Adding agent NetworkAgentInfo{ ni{[type: WIFI[] - WIFI, state: CONNECTED/CONNECTED, reason: (unspecified), extra: "fam_bager_net", roaming: false, failover: false, isAvailable: true, isConnectedToProvisioningNetwork: false]} network{545} lp{{InterfaceName: wlan0 LinkAddresses: [192.168.1.12/24,] Routes: [192.168.1.0/24 -> 0.0.0.0 wlan0,0.0.0.0/0 -> 192.168.1.1 wlan0,] DnsAddresses: [192.168.1.1,] Domains: null MTU: 0 TcpBufferSizes: 524288,1048576,4525824,524288,1048576,4525824}} nc{[ Transports: WIFI Capabilities: INTERNET&NOT_RESTRICTED&TRUSTED&NOT_VPN LinkUpBandwidth>=1048576Kbps LinkDnBandwidth>=1048576Kbps]} Score{60} validated{false} created{true} explicitlySelected{false} } for legacy network type 1
07-20 22:14:44.884 8415-5428/? E/SPPClientService﹕ [e] Push Channel Exception : java.net.SocketException: recvfrom failed: ETIMEDOUT (Connection timed out)
07-20 22:14:44.924 8415-5428/? E/SPPClientService﹕ [e] exceptionCaught(). NET_TIMEOUT
07-20 22:14:48.784 9179-9179/? E/SysUtils﹕ ApplicationContext is null in ApplicationStatus
您的Intent
不正確。
Intent intent = new Intent(menu_state.this, activity_game_state.class);
startActivity(intent);
此外,您第二個活動中的onCreate
方法也會被注釋掉。 這需要不加注釋。
這樣寫你的意圖,它應該可以工作:
Intent intent = new Intent(menu_state.this, activity_game_state.class);
startActivity(intent);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.