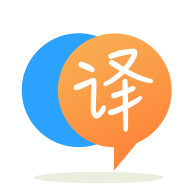
[英]How can I make a new Array and copy all the postive elements from the other array in the new array and return it?
[英]How can I make an array without one number of the other array?
我正在嘗試用兩個數組制作代碼。 除了最小的數目,第二個數組具有與第一個相同的值。 我已經編寫了一個代碼,其中z是最小的數字。 現在我只想制作一個沒有z的新數組,任何反饋將不勝感激。
public static int Second_Tiny() {
int[] ar = {19, 1, 17, 17, -2};
int i;
int z = ar[0];
for (i = 1; i < ar.length; i++) {
if (z >ar[i]) {
z=ar[i];
}
}
}
Java 8流具有內置功能,可以實現您想要的功能。
public static void main(String[] args) throws Exception {
int[] ar = {19, 1, 17, 17, -2, -2, -2, -2, 5};
// Find the smallest number
int min = Arrays.stream(ar)
.min()
.getAsInt();
// Make a new array without the smallest number
int[] newAr = Arrays
.stream(ar)
.filter(a -> a > min)
.toArray();
// Display the new array
System.out.println(Arrays.toString(newAr));
}
結果:
[19, 1, 17, 17, 5]
否則,您將看到以下內容:
public static void main(String[] args) throws Exception {
int[] ar = {19, 1, 17, 17, -2, -2, -2, -2, 5};
// Find the smallest number
// Count how many times the min number appears
int min = ar[0];
int minCount = 0;
for (int a : ar) {
if (minCount == 0 || a < min) {
min = a;
minCount = 1;
} else if (a == min) {
minCount++;
}
}
// Make a new array without the smallest number
int[] newAr = new int[ar.length - minCount];
int newIndex = 0;
for (int a : ar) {
if (a != min) {
newAr[newIndex] = a;
newIndex++;
}
}
// Display the new array
System.out.println(Arrays.toString(newAr));
}
結果:
[19, 1, 17, 17, 5]
我認為OP在看到他的評論時走在錯誤的軌道上:
“我正在嘗試找出數組ar []中的第二個最小整數。完成后,我應該得到1的輸出。要實現這一目標的方法是制作一個名為newar []的新數組,並使其包含ar []的所有索引,但不包括-2除外。”
這是解決此問題的非常低效的方法。 您將需要進行3次遍歷,一次是找到索引最小的元素,另一遍是刪除元素(這是一個數組,因此刪除元素將需要一整遍),另一遍是再次查找最小的元素。
您應該只執行一次遍歷算法,並跟蹤最小的兩個整數,甚至更好地使用樹來提高效率。 這是此問題的最佳答案:
更新:這是具有OP要求,3次通過且沒有外部庫的算法:
public static int Second_Tiny() {
int[] ar = {19, 1, 17, 17, -2};
//1st pass - find the smallest item on original array
int i;
int z = ar[0];
for (i = 1; i < ar.length; i++) {
if (z >ar[i]){
z=ar[i];
}
}
//2nd pass copy all items except smallest one to 2nd array
int[] ar2 = new int[ar.length-1];
int curIndex = 0;
for (i=0; i<ar.length; i++) {
if (ar[i]==z)
continue;
ar2[curIndex++] = ar[i];
}
//3rd pass - find the smallest item again
z = ar2[0];
for (i = 1; i < ar2.length; i++) {
if (z >ar2[i]){
z=ar2[i];
}
}
return z;
}
這將獲取變量z中指定的元素的索引,然后將第二個數組設置為第一個數組減去一個元素。
本質上,這給出了ar2 = ar1減去元素z
public static int Second_Tiny() {
int[] ar = {19, 1, 17, 17, -2};
int[] ar2;
int i;
int z = ar[0];
int x = 0;
for (i = 1; i < ar.length; i++) {
if (z >ar[i]){
z=ar[i];
x=i;
}
}
ar2 = ArrayUtils.remove(ar, x);
return(z);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.