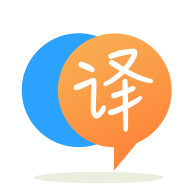
[英]replace special character }} in a string with “” using regexp in java
[英]find and replace characters using regexp java string
我想替換以下字符串之間的特殊字符,僅當它們位於兩個單詞 BEGIN 和 END 之間時。 xxx 到 CCC 和 yyy 到 DDD,使用 Java 正則表達式或某種方式? 你能幫我嗎
Raw string = "John Doe xxx Amazing man BEGIN reference xxx yes yyy indeed this is true xxx
no yyy END , so this xxx does not change"
converted String = "John Doe xxx Amazing man BEGIN reference CCC yes DDD indeed this is true
CCC no DDD END, so this xxx does not change"
您需要定義您的模式和匹配器:
Pattern MY_PATTERN = Pattern.compile("BEGIN" + "([ ]*+[0-9A-Za-z]++[ ]*+)*" + "xxx" + "([ ]*+[0-9A-Za-z]++[ ]*+)*" + "END");
Matcher m = MY_PATTERN.matcher(rawString);
然后你將在匹配器上調用 find 方法,每次它找到你想要的東西時都會用你需要的東西替換它:
while (m.find()) {
rawString = rawString.replaceFirst(m.group(0),m.group(0).replaceAll("xxx","CCC"));
}
即使您的示例數據在"yyy"
"xxx"
之前顯示"xxx"
,也沒有規范是"xxx"
在"yyy"
之前,反之亦然。 您只需聲明"special characters between the following String when only when they are between two words, BEGIN and END"
。
我看到有一個Map<String, String>
,其中鍵是您的“特殊”字符串,值是將替換“特殊”字符串的字符串。
遍歷此映射並提供其密鑰:
String.format("BEGIN.*?(%s).*?END", kvp.getKey())
在這個例子中,它將產生兩個正則表達式模式:
"BEGIN.*?(xxx).*?END"
"BEGIN.*?(yyy).*?END"
這會將您的“特殊”字符串捕獲到捕獲組 1 中,您將像這樣將其提供給String.replace()
:
raw = raw.replace(matcher.group(), matcher.group().replace(matcher.group(1), kvp.getValue()));
matcher.group()
是整個匹配字符串BEGIN ... END
和matcher.group(1)
將是xxx
或yyy
把這一切放在一起,你有:
public static void main(String[] args) throws Exception {
Map<String, String> replacerMap = new HashMap() {{
put("xxx", "CCC");
put("yyy", "DDD");
}};
String raw = "John Doe xxx Amazing man BEGIN reference xxx yes yyy indeed this is true xxx no yyy END , so this xxx does not change";
System.out.println("Before: ");
System.out.println(raw);
System.out.println();
for (Map.Entry<String, String> kvp : replacerMap.entrySet()) {
Matcher matcher = Pattern.compile(String.format("BEGIN.*?(%s).*?END", kvp.getKey())).matcher(raw);
if (matcher.find()) {
raw = raw.replace(matcher.group(), matcher.group().replace(matcher.group(1), kvp.getValue()));
}
}
System.out.println("After: ");
System.out.println(raw);
}
結果:
Before:
John Doe xxx Amazing man BEGIN reference xxx yes yyy indeed this is true xxx no yyy END , so this xxx does not change
After:
John Doe xxx Amazing man BEGIN reference CCC yes DDD indeed this is true CCC no DDD END , so this xxx does not change
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.