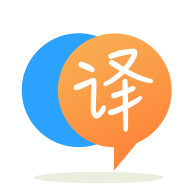
[英]How to insert attribute value that is the same with value right above element in XML using python parsing
[英]Insert XML Value/Element with Python
我一直在尋找將元素/值添加到當前XML文件的解決方案。 因此,假設我有以下XML文件:
<?xml version="1.0" encoding="UTF-8" standalone="yes"?>
<Root xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<Node>
<Name>Sprinkler</Name>
<Type>Blah</Type>
<Prob>0.82</Prob>
</Node>
<Node>
<Name>Rain</Name>
<Type>Bleh</Type>
<Prob>0.23</Prob>
</Node>
<Node>
<Name>Cloudy</Name>
<Type>Bluh</Type>
<Prob>0.71</Prob>
</Node>
</Root>
現在,我的目標是給定一個CSV文件,我想為每個節點添加新的元素和值。 假設我的CSV包含以下內容:
Cloudy,Or,Sprinkler,Rain
Sprinkler,And,Rain
Rain,Or,Sprinkler,Cloudy
我能夠毫無問題地讀取CSV,我的問題是添加了新元素'Parent0'和'Parent1'(如果有),因此輸出如下所示:
<?xml version="1.0" encoding="UTF-8" standalone="yes"?>
<Root xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<Node>
<Name>Sprinkler</Name>
<Type>Blah</Type>
<Prob>0.82</Prob>
<Gate>And</Gate>
<Parent0>Rain</Parent0>
</Node>
<Node>
<Name>Rain</Name>
<Type>Bleh</Type>
<Prob>0.23</Prob>
<Gate>Or</Gate>
<Parent0>Sprinkler</Parent0>
<Parent1>Cloudy</Parent1>
</Node>
<Node>
<Name>Cloudy</Name>
<Type>Bluh</Type>
<Prob>0.71</Prob>
<Gate>Or</Gate>
<Parent0>Sprinkler</Parent0>
<Parent1>Rain</Parent1>
</Node>
</Root>
到目前為止,我已經用Python編寫了以下內容:
import xml.etree.ElementTree as ET
xml = ET.parse('XML.xml')
for row in xml.iterfind('Node'):
i = 1
for item in csvFile:
row.append('<Gate>'+item[1]+'</Gate>\n')
if i != 1:
for x in xrange(0, len(item):
if row.findtext('Name') == item[x]:
row.append('<Parent0>'+item[x]+'</Parent0>\n')
else:
i = 0
在我的代碼上,現在所有這些都將轉到Parent0,但是我想看看如何在不刪除所有內容的情況下進行追加? 我聽說過lxml和minidom,但不確定它們如何工作。 如果我能夠使用xml.etree.ElementTree做到這一點,那就太好了。
一種簡單的方法是使用ElementTree.SubElement()
創建元素,它將自動將這些元素添加到父節點的末尾,並作為參數傳入。
示例/演示-
>>> import xml.etree.ElementTree as ET
>>> import csv
>>> with open('test.csv','r') as f:
... cfiles = list(csv.reader(f))
...
>>> xml = ET.parse('XML.xml')
>>> for row in xml.iterfind('.//Node'):
... name = row.find('./Name').text
... for i in cfiles:
... if i[0] == name:
... j = 1
... while j < len(i):
... if j == 1:
... g = ET.SubElement(row,'Gate')
... g.text = i[j]
... elif j == 2:
... g = ET.SubElement(row,'Parent0')
... g.text = i[j]
... elif j == 3:
... g = ET.SubElement(row,'Parent1')
... g.text = i[j]
... j += 1
...
>>> print(ET.tostring(xml.getroot()).decode())
<Root>
<Node>
<Name>Sprinkler</Name>
<Type>Blah</Type>
<Prob>0.82</Prob>
<Gate>And</Gate><Parent0>Rain</Parent0></Node>
<Node>
<Name>Rain</Name>
<Type>Bleh</Type>
<Prob>0.23</Prob>
<Gate>Or</Gate><Parent0>Sprinkler</Parent0><Parent1>Cloudy</Parent1></Node>
<Node>
<Name>Cloudy</Name>
<Type>Bluh</Type>
<Prob>0.71</Prob>
<Gate>Or</Gate><Parent0>Sprinkler</Parent0><Parent1>Rain</Parent1></Node>
</Root>
上面的cfiles
是我先前從csv文件創建的列表。
要將xml寫入新文檔,請執行以下操作-
with open('newxml.xml','w') as of:
of.write(ET.tostring(xml.getroot()).decode())
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.