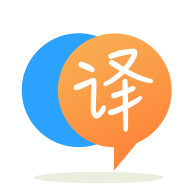
[英]Parcelable object is Null on receiving activity and good in other
[英]Parcelable object is Null on receiving activity
我已將Parcelable實施為一個對象,該對象要在成功登錄時在活動之間傳遞。 唯一的問題是,對象(用戶)在收到意圖后始終具有空字段。
我已經在這里搜索了很多問題,由於似乎無法找到問題,目前我完全陷入困境。
我作為額外對象傳入的對象具有正確的值,但是無論我如何嘗試接收它,它始終具有空字段。
這是我的User類:
public class User implements Parcelable{
private String name;
private String email;
private String password;
private int age;
private int weight;
private int height;
private long id;
public User(String name, String email, String password, int age, int weight, int height, long id) {
this.name = name;
this.email = email;
this.password = password;
this.age = age;
this.weight = weight;
this.height = height;
this.id = id;
}
public User(String name, String email, String password) {
this.name = name;
this.email = email;
this.password = password;
}
/**
* empty constructor
*/
public User(){
}
@Override
public String toString() {
return "User{" +
"name='" + name + '\'' +
", email='" + email + '\'' +
", password='" + password + '\'' +
", age=" + age +
", weight=" + weight +
", height=" + height +
", id=" + id +
'}';
}
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public int getWeight() {
return weight;
}
public void setWeight(int weight) {
this.weight = weight;
}
public int getHeight() {
return height;
}
public void setHeight(int height) {
this.height = height;
}
//Parcelable methods below.
// 99.9% of the time you can just ignore this
public int describeContents() {
return 0;
}
// write your object's data to the passed-in Parcel
public void writeToParcel(Parcel out, int flags) {
out.writeString(name);
out.writeString(email);
out.writeInt(age);
out.writeInt(weight);
out.writeInt(height);
out.writeLong(id);
}
// this is used to regenerate your object. All Parcelables must have a CREATOR that implements these two methods
public static final Parcelable.Creator< User> CREATOR = new Parcelable.Creator<User>() {
public User createFromParcel(Parcel in) {
return new User(in);
}
public User[] newArray(int size) {
return new User[size];
}
};
// example constructor that takes a Parcel and gives you an object populated with it's values
private User(Parcel in) {
User pUser = new User();
pUser.setName(in.readString());
pUser.setEmail(in.readString());
pUser.setAge(in.readInt());
pUser.setWeight(in.readInt());
pUser.setHeight(in.readInt());
pUser.setId(in.readLong());
}
}
這是我的主要活動,旨在發送額外的Parcelable:
public class MainActivity extends AppCompatActivity {
private DatabaseHelper db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// ifActEmpty(db);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu items for use in the action bar
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.menu_main, menu);
return super.onCreateOptionsMenu(menu);
}
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
/**
* Method called when the register link button is clicked. Calls intent to start the
* registerActivity.
* @param v
*/
public void navigateToRegister(View v){
Intent intent = new Intent(getApplicationContext(), RegisterActivity.class);
intent.addFlags(intent.FLAG_ACTIVITY_CLEAR_TOP);
startActivity(intent);
finish();
}
/**
* method called when the login button is clicked. Takes the input from username and password
* edittext fields and queries the database to see if valid user. Returns an intent to go to
* homeActivity.
* @param view
*/
public void login(View view){
db = new DatabaseHelper(this);
//when this button is clicked we need to access the DB to see if the users
// credentials typed into the two edittext fields match those in the DB.
final EditText emailEditText = (EditText) findViewById(R.id.loginEmail);
final EditText passwordEditText = (EditText) findViewById(R.id.loginPassword);
//get the strings from edittext fields.
String email = emailEditText.getText().toString();
String password = passwordEditText.getText().toString();
//debugging
Log.v("EditText", emailEditText.getText().toString());
Log.v("EditText", passwordEditText.getText().toString());
//check for user input
if (isEmpty(emailEditText) || isEmpty(passwordEditText)) {
Toast.makeText(MainActivity.this.getBaseContext(),
"Please complete all fields.", Toast.LENGTH_SHORT).show();
return;
} else {
//do some magic to see if the password and username match...
//if they match then send an intent to the home activity
User userResult = db.getUser(email, password);
//Log.v("UserRes getUser(email):", userResult.getEmail());
// Log.v("UserRes getUser(px):", userResult.getPassword());
if (userResult.getEmail() == null) {
Toast.makeText(MainActivity.this.getBaseContext(),
"Username or password invalid.", Toast.LENGTH_SHORT).show();
return;
} else {
Toast.makeText(MainActivity.this.getBaseContext(),
"Success.", Toast.LENGTH_SHORT).show();
Log.d("user MA: ", userResult.toString());
Intent intent;
intent = new Intent(MainActivity.this, HomeActivity.class);
intent.putExtra("User_Object", userResult);
MainActivity.this.startActivity(intent);
}
}
db.closeDB();
}
}
最后,我要在其中接收用戶對象的HomeActivity類:
公共類HomeActivity擴展了AppCompatActivity {
private User thisUser;//want this to be private!!
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_home);
User user;
Bundle bundle = getIntent().getExtras();
Intent i = getIntent();
user = (User) i.getParcelableExtra("User_Object");
User newUser = bundle.getParcelable("User_Object");
Log.d("user HA PE: ", newUser.toString());
Log.d("user HA B: ", user.toString());
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_home, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
/**
* method called to instantiate a new intent to go to viewScoreActivity screen.
* @param view
*/
public void viewScoresLink(View view){
Intent intent;
intent = new Intent(HomeActivity.this, ViewScoreActivity.class);
intent.putExtra("User_Object", thisUser);
HomeActivity.this.startActivity(intent);
}
/**
* method called to instantiate a new intent to go to the submitScoreActiviyt screen.
* @param view
*/
public void addNewScoreLink(View view){
Intent intent;
intent = new Intent(HomeActivity.this, AddScoreActivity.class);
intent.putExtra("User_Object", thisUser);
HomeActivity.this.startActivity(intent);
}
public void logout(View view){
Intent intent;
intent = new Intent(HomeActivity.this, MainActivity.class);
HomeActivity.this.startActivity(intent);
}
我在運行時沒有任何異常。 對象字段全為空
這是因為您為User
重載了私有構造函數。 不要創建該類的私有實例。 更改為:
private User(Parcel in) {
setName(in.readString());
setEmail(in.readString());
setAge(in.readInt());
setWeight(in.readInt());
setHeight(in.readInt());
setId(in.readLong());
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.