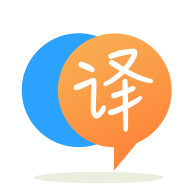
[英]How to make a new instance of an object that doesn't overwrite the existing one?
[英]How to delete an existing object and make a new one in java?
我想刪除在其他班級中創建的班級的上一個對象,並在其后創建一個新對象。
這是我的代碼的一部分:
//DataBase
DataBase data_base;
Document financial_document;
/**
* Creates new form FinancialDocumentsJFrame
*/
public FinancialDocumentsJFrame() {
frameWidth = this.getWidth();
frameHeight = this.getHeight();
panelWidth = frameWidth;
panelHeight = frameHeight;
data_base = new DataBase();
code_table_hashmap = data_base.code_table;
activity_table_hashmap = data_base.activity_table;
client_table_hashmap = data_base.client_table;
employee_table_hashmap = data_base.employee_table;
initComponents();
// Sets size of the frame.
if(false) // Full screen mode
{
// Disables decorations for this frame.
//this.setUndecorated(true);
// Puts the frame to full screen.
this.setExtendedState(this.MAXIMIZED_BOTH);
}
else // Window mode
{
// Size of the frame.
this.setSize(1100, 800);
// Puts frame to center of the screen.
this.setLocationRelativeTo(null);
// So that frame cannot be resizable by the user.
this.setResizable(false);
}
}
我在上課之前已經聲明了該對象,並在該類的一個方法中創建了一個新對象。
現在,我需要用另一種方法刪除該對象並制作一個新方法。
這是我的代碼:
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
if (bill_documentType==1){
fd_document_type = true;
}
else if (bill_documentType==0){
fd_document_type = false;
}
else {
System.out.println("Error in Document Type!!!!!!!!!");
}
if (bill_documentStatus==1){
fd_document_status = true;
}
else if (bill_documentStatus==0){
fd_document_status = false;
}
else {
System.out.println("Error in Document Status!!!!!!!!!");
}
if (jTextField1.getText().equals("") || jTextField2.getText().equals("") || jTextField3.getText().equals("")|| jTextField4.getText().equals("")|| jTextField5.getText().equals("")|| bill_documentStatus==-1){
JOptionPane.showConfirmDialog(null, "لطفا همه فيلد ها را پر كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else if (jTextField1.getText().length()!=7 || jTextField2.getText().length()!=10 || jTextField3.getText().length()>10 || jTextField5.getText().length()>10) {
JOptionPane.showConfirmDialog(null, "ورودي نامعتبر است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else if (!checkDocumentNumberText(jTextField1.getText())){
JOptionPane.showConfirmDialog(null, "ورودي شماره سند نامعتبر است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else if (!checkDocumentDateText(jTextField2.getText())){
JOptionPane.showConfirmDialog(null, "ورودي تارخ سند نامعتبر است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else if (!checkDocumentClientNumberText(jTextField3.getText())){
JOptionPane.showConfirmDialog(null, "ورودي شماره مشتري نامعتبر است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else if (!checkDocumentAmountText(jTextField4.getText())){
JOptionPane.showConfirmDialog(null, "ورودي مبلغ نامعتبر است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else if (!checkDocumentEmployeeNumberText(jTextField5.getText())) {
JOptionPane.showConfirmDialog(null, "ورودي شماره پرسنلي نامعتبر است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else if (!checkDocumentEmployeeNumberExistence(Integer.parseUnsignedInt(jTextField5.getText())) || !checkDocumentClientNumberExistence(Integer.parseUnsignedInt(jTextField3.getText()))){
JOptionPane.showConfirmDialog(null, "ورودي شماره مشتري يا شماره پرسنلي ناموجود است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else if (jComboBox2.getSelectedIndex()==-1){
JOptionPane.showConfirmDialog(null, "كد معين انتخاب نشده است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else if (jComboBox1.getSelectedIndex()==-1 && jTextField6.getText().equals("") ){
JOptionPane.showConfirmDialog(null, "شرح انتخاب نشده است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else {
boolean exists = data_base.selectQueryCheckDocument("Select * From document;",Integer.parseUnsignedInt(jTextField1.getText()), fd_document_type, fd_document_status, jTextField2.getText(), Integer.parseUnsignedInt(jTextField3.getText()));
if (exists) {
JOptionPane.showConfirmDialog(null, "ورودي موجود است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else {
jButton2.setEnabled(true);
jButton3.setEnabled(true);
jTextField1.setEnabled(false);
jTextField2.setEnabled(false);
jTextField3.setEnabled(false);
jButton1.setEnabled(false);
fd_document_number = Integer.parseUnsignedInt(jTextField1.getText());
fd_document_date = jTextField2.getText();
fd_document_statement = jComboBox1.getSelectedItem().toString();
fd_document_code_number = getCodeNumber(jComboBox2.getSelectedItem().toString());
fd_document_employee_number = Integer.parseUnsignedInt(jTextField5.getText());
fd_document_client_number = Integer.parseUnsignedInt(jTextField3.getText());
document_amount = Integer.parseUnsignedInt(jTextField4.getText());
***here is the object creation: financial_document = new Document(fd_document_number, fd_document_type, fd_document_status, fd_document_date, fd_document_statement, fd_document_code_number, fd_document_employee_number, fd_document_client_number, document_amount);
financial_document.printDocumentInformation();
}
}
}
現在,我需要刪除對象並創建新對象的下一個方法:
private void jButton2ActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
*** deleting: financial_document = null;
if (bill_documentType==1){
fd_document_type = true;
}
else if (bill_documentType==0){
fd_document_type = false;
}
else {
System.out.println("Error in Document Type!!!!!!!!!");
}
if (bill_documentStatus==1){
fd_document_status = true;
}
else if (bill_documentStatus==0){
fd_document_status = false;
}
else {
System.out.println("Error in Document Status!!!!!!!!!");
}
if (jTextField4.getText().equals("")|| jTextField5.getText().equals("")|| bill_documentStatus==-1){
JOptionPane.showConfirmDialog(null, "لطفا همه فيلد ها را پر كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else if (!checkDocumentAmountText(jTextField4.getText())){
JOptionPane.showConfirmDialog(null, "ورودي مبلغ نامعتبر است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else if (!checkDocumentEmployeeNumberText(jTextField5.getText())) {
JOptionPane.showConfirmDialog(null, "ورودي شماره پرسنلي نامعتبر است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else if (!checkDocumentEmployeeNumberExistence(Integer.parseUnsignedInt(jTextField5.getText())) || !checkDocumentClientNumberExistence(Integer.parseUnsignedInt(jTextField3.getText()))){
JOptionPane.showConfirmDialog(null, "ورودي شماره مشتري يا شماره پرسنلي ناموجود است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else if (jComboBox2.getSelectedIndex()==-1){
JOptionPane.showConfirmDialog(null, "كد معين انتخاب نشده است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else if (jComboBox1.getSelectedIndex()==-1 && jTextField6.getText().equals("") ){
JOptionPane.showConfirmDialog(null, "شرح انتخاب نشده است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else {
boolean exists = data_base.selectQueryCheckDocument("Select * From document;",Integer.parseUnsignedInt(jTextField1.getText()), fd_document_type, fd_document_status, jTextField2.getText(), Integer.parseUnsignedInt(jTextField3.getText()));
if (exists) {
JOptionPane.showConfirmDialog(null, "ورودي موجود است لطفا دوباره بررسي كنيد", "Message", JOptionPane.PLAIN_MESSAGE);
}
else {
fd_document_number = Integer.parseUnsignedInt(jTextField1.getText());
fd_document_date = jTextField2.getText();
fd_document_statement = jComboBox1.getSelectedItem().toString();
fd_document_code_number = getCodeNumber(jComboBox2.getSelectedItem().toString());
fd_document_employee_number = Integer.parseUnsignedInt(jTextField5.getText());
fd_document_client_number = Integer.parseUnsignedInt(jTextField3.getText());
document_amount = Integer.parseUnsignedInt(jTextField4.getText());
***New object creation: financial_document = new Document(fd_document_number, fd_document_type, fd_document_status, fd_document_date, fd_document_statement, fd_document_code_number, fd_document_employee_number, fd_document_client_number, document_amount);
financial_document.printDocumentInformation();
}
}
}
我想知道是否financial_document = null; 付諸實踐,代碼將正常運行。
如果您將financial_document = null
設置financial_document = null
,則在您的應用程序中沒有其他引用的情況下,它將在下一次“垃圾回收”期間從內存中刪除。 在下一次垃圾回收之前,它實際上已被刪除,但是從理論上講,您仍然可以通過轉儲運行應用程序的計算機的內存來訪問它。 通過將字段設置為private
字段而不是默認的訪問說明符,可以幫助防止對其進行其他引用。
問題不只是創建/刪除對象,而是問自己:“誰知道這個對象?”。
如果你做...
MyClass x = new MyClass();
...然后創建一個類型為“ MyClass”的新對象,並將對它的引用存儲在變量x中。 因此,無論在x中定義什么,它都“知道”新創建的MyClass對象。 如果你現在做...
x = null;
...然后,對“舊” MyClass對象的引用將被“忘記” /用null
覆蓋。 如果沒有在其他地方傳遞x,則其他任何對象都不會“知道” x,因此x實際上會“丟失”,並且會被垃圾收集器從內存中完全刪除。
同樣的事情是您覆蓋x:
x = new MyClass();
同樣,之前存儲在“ x”中的舊對象將被“遺忘”。
至於因為你宣布你的代碼不起作用 financial_document
類之外,很難猜測其是否工作正常,但如果你用一個新的替換你的舊文檔的參考,從理論上講,這應該有效地“刪除”您的是內存中的舊對象,是的。
編輯:當您刪除對financial_document的引用時,它應該有效地從內存中“刪除”舊對象,是的,除非您同時已將其傳遞到外部。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.